Write a Python program to calculate the average of list items using user defined sum() function, len(), mean, reduce functions, and for loop. You can use any of the following built-in functions or iteration approaches.
Python Program to calculate the Average of List Items using sum() and len() functions
In this example, we use the built-in sum() function to find the total and the len() function to get the total number of items. Next, divide the list sum with the total items to get the average.
avlst = [10, 20, 90, 30, 40, 50] avg = sum(avlst) / len(avlst) print('The Result = ', avg)
The Result = 40.0
This example allows entering list items and calculates the sum and average using the sum function.
avglist = [] Number = int(input("Total Number of List Items = ")) for i in range(1, Number + 1): value = int(input("Please enter the %d List Item = " %i)) avglist.append(value) total = sum(avglist) avg = total / Number print('\nThe Sum Of List Items = ', total) print('\nThe Average Of List Items = ', avg)
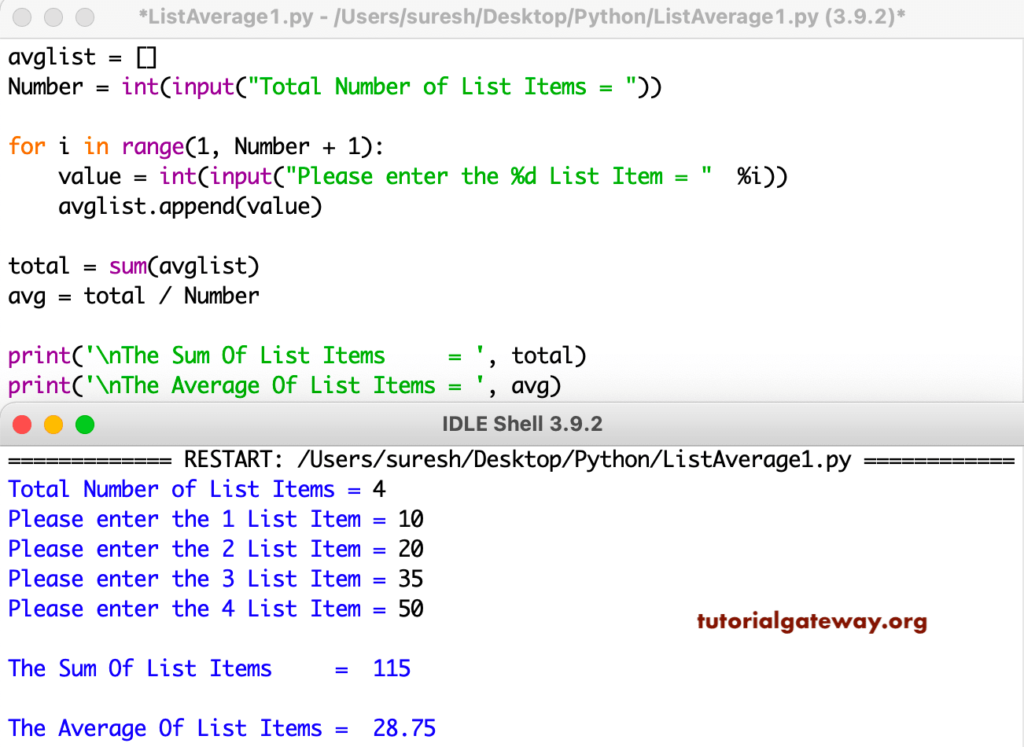
Python Program to find List Average using for loop
This program uses the for loop to iterate the items and calculates the sum and average of all list elements.
avglist = [] total = 0 Number = int(input("Total Number of List Items = ")) for i in range(1, Number + 1): value = int(input("Please enter the %d List Item = " %i)) avglist.append(value) for i in range(Number): total = total + avglist[i] avg = total / Number print('\nThe Sum Of List Items = ', total) print('\nThe Average Of List Items = ', avg)
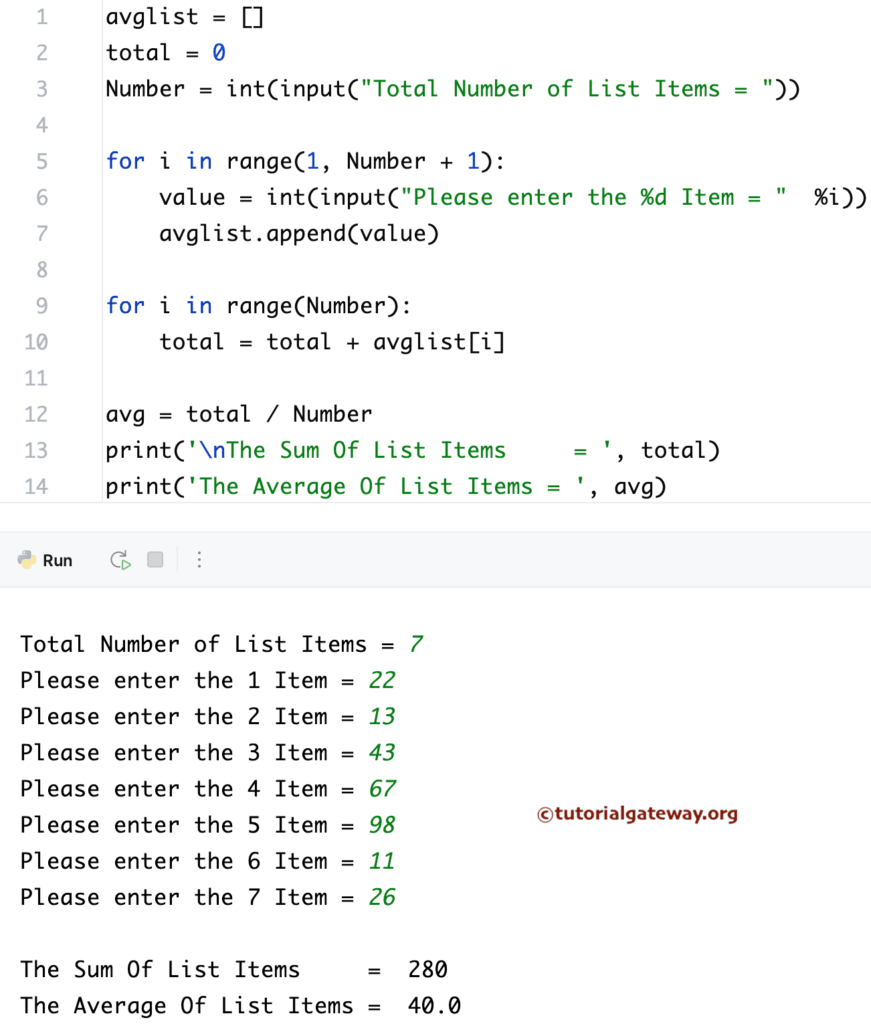
The above example uses the for loop range function. However, you can use a simple for loop to calculate the list average.
avlst = [17, 25, 85, 90, 33, 40, 50] tot = 0 for i in avlst: tot += i avg = tot / len(avlst) print('The Result = ', avg)
The Result = 48.57142857142857
Python Program to Calculate the Average of List Items using functions
In this example, the listAverage function accepts a list and returns the sum and average of all items.
def listAverage(avglist): total = 0 for i in range(Number): total = total + avglist[i] avg = total / len(avglist) return total, avg avglist = [] Number = int(input("Total Number of Items = ")) for i in range(1, Number + 1): value = int(input("Please enter the %d List Item = " %i)) avglist.append(value) total, avg = listAverage(avglist) print('\nThe Sum = ', total) print('\nThe Average = ', avg)
Total Number of Items = 8
Please enter the 1 List Item = 2
Please enter the 2 List Item = 9
Please enter the 3 List Item = 11
Please enter the 4 List Item = 24
Please enter the 5 List Item = 58
Please enter the 6 List Item = -11
Please enter the 7 List Item = 17
Please enter the 8 List Item = 99
The Sum = 209
The Average = 26.125
Python Program to find the Average of List Items using built-in methods
This programming language has many options to calculate the list average, and this section covers most of them.
Using mean and reduce functions
In this Python program, we imported the mean from the statistics module and reduce method from the functools module. Both these functions will calculate the average of list items.
from statistics import mean from functools import reduce avglist = [] Number = int(input("Total Number of List Items = ")) for i in range(1, Number + 1): value = int(input("Please enter the %d List Item = " %i)) avglist.append(value) total = sum(avglist) avg1 = mean(avglist) avg2 = reduce(lambda x, y: x + y, avglist) / Number #avg2 = reduce(lambda x, y: x + y, avglist) /len(avglist) print('\nThe Sum = ', total) print('\nThe Average = ', avg1) print('\nThe Average = ', avg2)
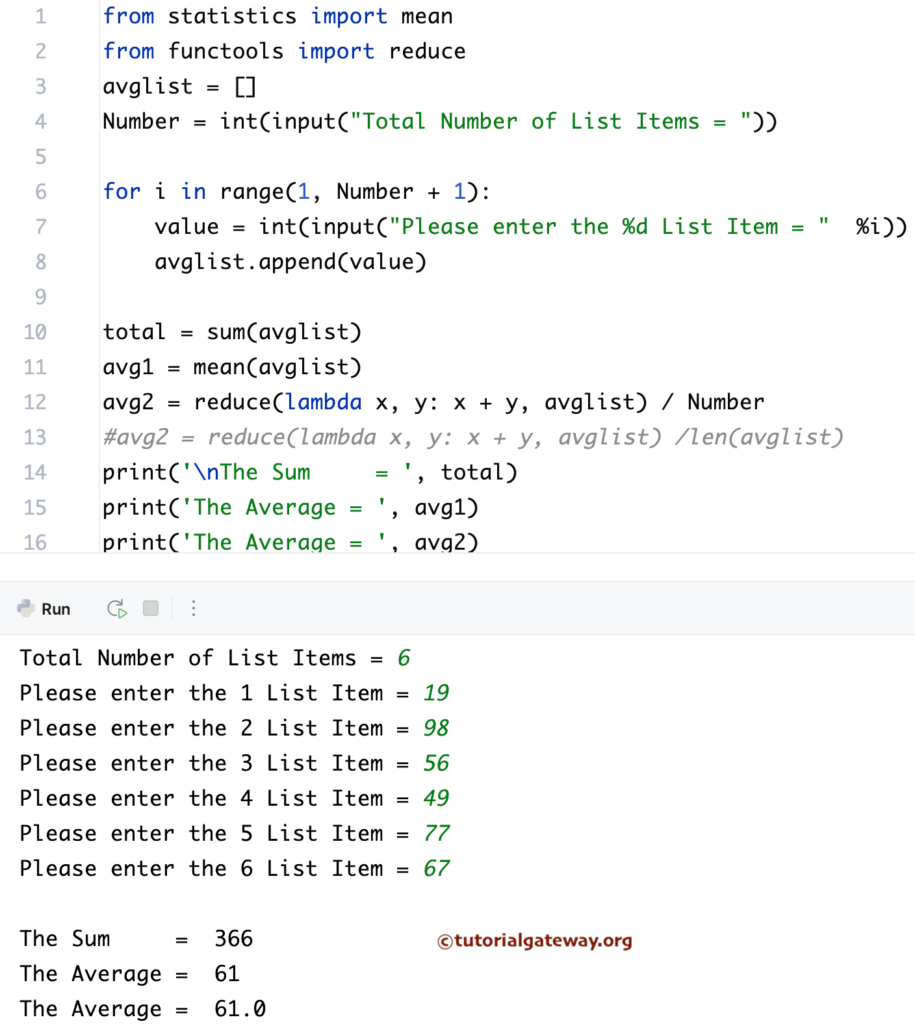
Using the numpy mean function
In this example, first, we have to import the numpy module to use the mean function.
import numpy as np avlst = [98, 44, 36, 89, 11, 14] avg = np.mean(avlst) print('The Result = ', avg)
The Result = 48.666666666666664
Python List Average using lambda function and generator expression
avlst = [10, 20, 30, 40, 50, 60, 70] avg = (lambda n: sum(n) / len(n))(avlst) print('The Result = ', avg) avg = sum(n for n in avlst) / len(avlst) print('The Result = ', avg)
The Result = 40.0
The Result = 40.0