Write a Python Program to calculate Sum of Series 1²+2²+3²+….+n² using For Loop and Functions with an example.
The Mathematical formula for Python Sum of series 1²+2²+3²+….+n² = ( n (n+1) (2n+1)) / 6
Python Program to calculate Sum of Series 1²+2²+3²+….+n²
This Python program asks the user to enter any positive integer. Next, the Python program finds the sum of series 12 + 22 + 32 + … + n2 using the above formula.
# Python Program to calculate Sum of Series 1²+2²+3²+….+n² number = int(input("Please Enter any Positive Number : ")) total = 0 total = (number * (number + 1) * (2 * number + 1)) / 6 print("The Sum of Series upto {0} = {1}".format(number, total))
Python Sum of Series 1²+2²+3²+….+n² output
Please Enter any Positive Number : 6
The Sum of Series upto 6 = 91.0
Sum = (Number * (Number + 1) * (2 * Number + 1 )) / 6
Sum = (6 * (6 + 1) * (2 * 6 +1)) / 6 => (6 * 7 * 13) / 6
and the output, Sum = 91
Python Program to calculate Sum of Series 1²+2²+3²+….+n² Example 2
If you want the Python to display the series order 12 + 22 + 32 +42 + 52, we have to add extra For Loop along with If Else
number = int(input("Please Enter any Positive Number : ")) total = 0 total = (number * (number + 1) * (2 * number + 1)) / 6 for i in range(1, number + 1): if(i != number): print("%d^2 + " %i, end = ' ') else: print("{0}^2 = {1}".format(i, total))
Please Enter any Positive Number : 7
1^2 + 2^2 + 3^2 + 4^2 + 5^2 + 6^2 + 7^2 = 140.0
Python Program to calculate Sum of Series 1²+2²+3²+….+n² using Functions
This Python Sum of Series 1²+2²+3²+….+n² program is the same as above. But in this Python program, we are defining a Functions to place logic.
def sum_of_square_series(number): total = 0 total = (number * (number + 1) * (2 * number + 1)) / 6 for i in range(1, number + 1): if(i != number): print("%d^2 + " %i, end = ' ') else: print("{0}^2 = {1}".format(i, total)) num = int(input("Please Enter any Positive Number : ")) sum_of_square_series(num)
Please Enter any Positive Number : 9
1^2 + 2^2 + 3^2 + 4^2 + 5^2 + 6^2 + 7^2 + 8^2 + 9^2 = 285.0
Python Program to calculate Sum of Series 1²+2²+3²+….+n² using Recursion
Here, we are using the Python Recursive function to find the Sum of Series 1²+2²+3²+….+n².
def sum_of_square_series(number): if(number == 0): return 0 else: return (number * number) + sum_of_square_series(number - 1) num = int(input("Please Enter any Positive Number : ")) total = sum_of_square_series(num) print("The Sum of Series upto {0} = {1}".format(num, total))
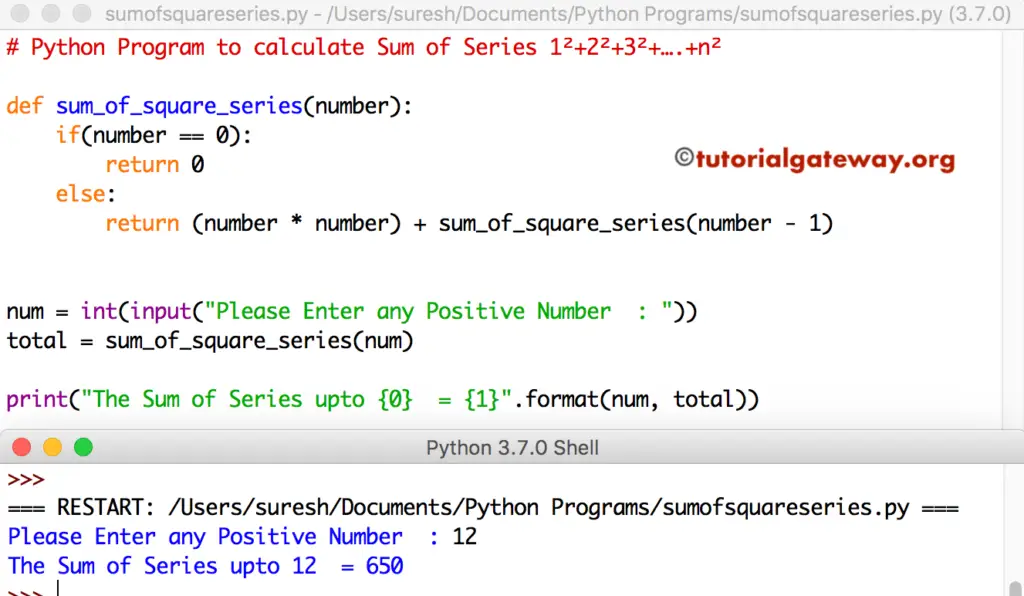