Write a Python Program to Print a Hollow Inverted or revered Mirrored Right Triangle Star Pattern using a for loop, while loop, and functions. This example uses the nested for loop to iterate and print the Hollow Inverted Mirrored Right Triangle Star Pattern.
rows = int(input("Enter Hollow Inverted Mirrored Right Triangle Rows = ")) print("====Hollow Inverted Mirrored Right Angled Triangle Star Pattern====") for i in range(rows, 0, -1): for j in range(rows - i, 0, -1): print(' ', end = '') for k in range(0, i): if i == 1 or i == rows or k == 0 or k == i - 1: print('*', end = '') else: print(' ', end = '') print()
Output.
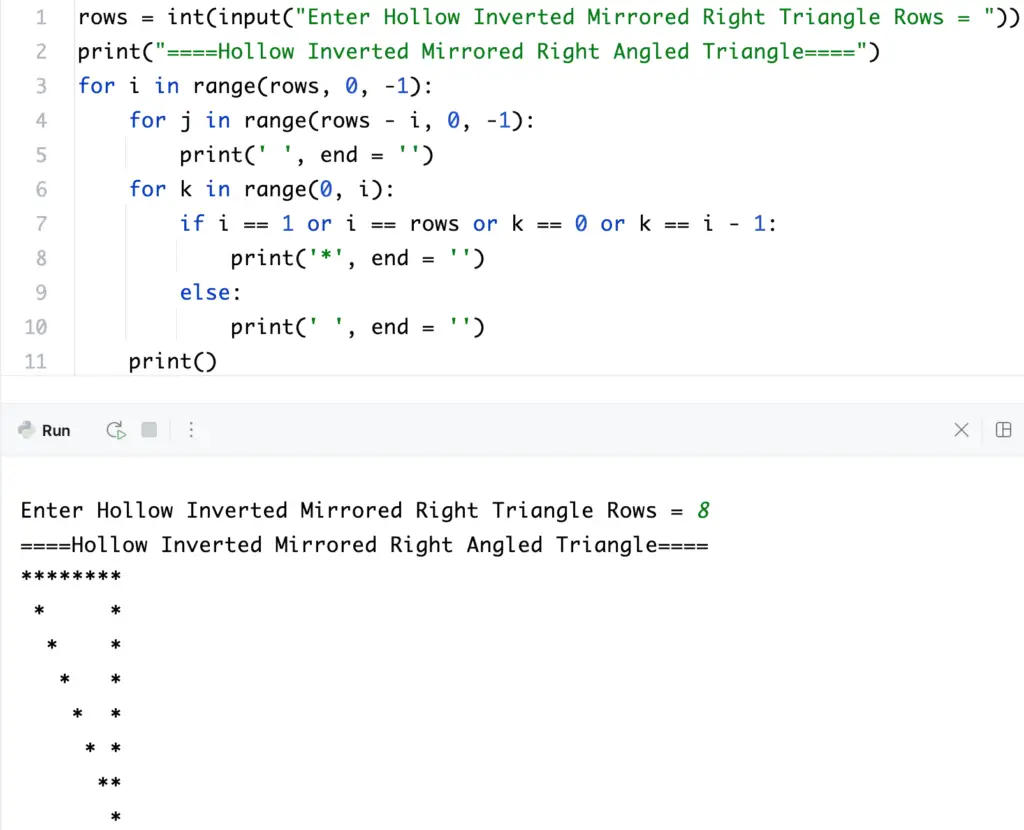
This Python program replaces the above for loop with a while loop to print the Hollow Inverted Mirrored Right Angled Triangle Star Pattern.
rows = int(input("Enter Hollow Inverted Mirrored Right Triangle Rows = ")) print("====Hollow Inverted Mirrored Right Angled Triangle Star Pattern====") i = rows while i > 0: j = rows - i while j > 0: print(' ', end = '') j = j - 1 k = 0 while k < i: if i == 1 or i == rows or k == 0 or k == i - 1: print('*', end = '') else: print(' ', end = '') k = k + 1 i = i - 1 print()
Output
Enter Hollow Inverted Mirrored Right Triangle Rows = 14
====Hollow Inverted Mirrored Right Angled Triangle Star Pattern====
**************
* *
* *
* *
* *
* *
* *
* *
* *
* *
* *
* *
**
*
In this Python example, we created a HolInvMirRightTri function that accepts the rows and characters to Print the Hollow Reversed Mirrored Right Angled Triangle Star Pattern. It replaces the star in the Hollow Inverted Mirrored Right Angled Triangle pattern with a given symbol.
def HolInvMirRightTri(rows, ch): for i in range(rows, 0, -1): for j in range(rows - i, 0, -1): print(' ', end = '') for k in range(0, i): if i == 1 or i == rows or k == 0 or k == i - 1: print('%c' %ch, end = '') else: print(' ', end = '') print() rows = int(input("Enter Hollow Inverted Mirrored Right Triangle Rows = ")) ch = input("Symbol in Hollow Inverted Mirrored Right Triangle = ") HolInvMirRightTri(rows, ch)
Output
Enter Hollow Inverted Mirrored Right Triangle Rows = 12
Symbol in Hollow Inverted Mirrored Right Triangle = $
$$$$$$$$$$$$
$ $
$ $
$ $
$ $
$ $
$ $
$ $
$ $
$ $
$$
$