The JavaScript while loop is a common one to perform repetitive tasks. This article will explain the working mechanism of the JavaScript while loop, syntax, flow chart, and how to iterate over data to perform calculations on individual items. Additionally, we cover the infinite while loop and a relay-time complex example.
A while Loop in JavaScript is useful to repeat a code block or block of statements for a given number of times until the given condition is False. This robust iterator will help you to loop over the data and implement complex logic for each iteration.
The JavaScript loop starts with the while keyword followed by the condition. If the condition is True, statements inside it will execute. If it is false, it won’t run at least once. It means while loop may execute zero or more times.
What is the Syntax of the JavaScript while loop?
The syntax of the Javascript While loop is as follows:
While( Condition ) { statement 1; statement 2; …………. } It is the statement Outside the While
From the above JavaScript syntax, the condition, also known as expression, determines whether the while loop should iterate or not. If the condition evaluates to True, the statement or group of statements within the flower brackets will execute. Once the expression becomes False, the loop will terminate and execute other statements outside the loop (after })
JavaScript while Loop Flow Chart
The flow chart of the while loop is as shown below:
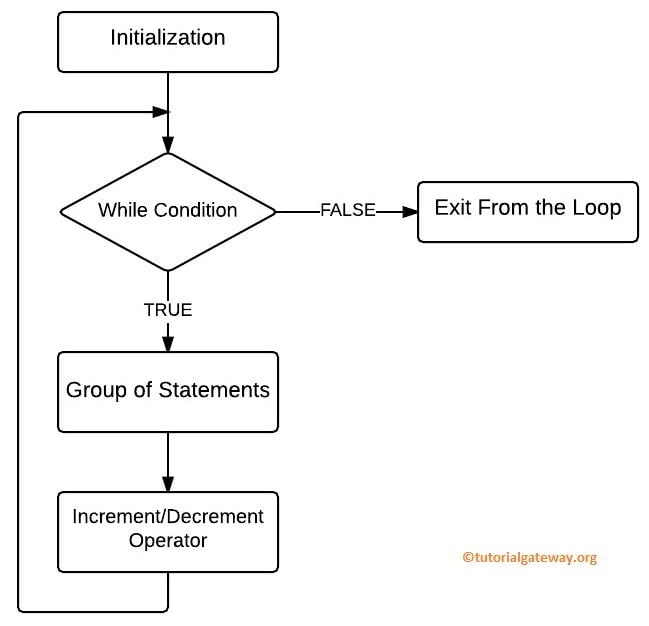
How does a while() loop work?
Within the Javascript code, when the while loop encounters, it will first evaluate the condition before the iteration starts. If the result is True, the statements within the loop will execute. Before the end of the loop, the Increment & Decrement Operator inside it will increase or decrease the value. After the iteration completes, the JavaScript while loop will again evaluate the expression with the updated value. This process will repeat until the condition returns a boolean false. Once it returns False, it will exit entirely from the loop.
What are the key components of a while loop in js?
- Condition: An expression that decides whether the Javascript while loop iteration should run or terminate. Remember, if the expression is false initially, it won’t return anything from the code block.
- Code block: The code or multiple statements enclosed with the curly braces {}. As long as the condition returns True, these lines will execute. We can name the execution of the code block as the iteration.
- Iteration: Each loop is called an iteration. It Starts from { point and ends at }.
- Increment or Decrement Value: It is the most crucial component. If you miss it, it becomes an infinite loop.
By now, you have the theoretical knowledge of JavaScript while loop; let us see the practical example for a better understanding.
JavaScript while Loop example to add numbers
This example will add the numbers from 6 to 10 and return their sum. It is a simple example to demonstrate this Javascript while loop. However, you can change the variable number value to 1, condition from 10 to 20, or increment by two.
In this JavaScript while loop program, we declared a number and total variables and initialized the values as 6 and 0. By using it, the compiler will add those values up to 10.
<!DOCTYPE html> <html> <head> <title> Example </title> </head> <body> <h1> Example </h1> <script> var number = 6, total=0; while (number <= 10) { total = total + number; number++; } document.write("Total = ", total); </script> </body> </html>
Analysis
- In this JavaScript code example, we defined the while Loop with the condition (number <= 10).
- The value inside the number variable (6) will test against the condition, i.e., (6 <= 10).
- If the condition results true, the number will add to the total. Otherwise, it will exit from the JavaScript iteration.
- After adding the value to the total variable, we used the ++ operator to increment the number value. After incrementing, the process repeats until the condition results False.
- The loop will continue executing until the number reaches 11.
Here, Our number = 6. It means, total = 6+7+8+9+10 = 40
Example
Total = 40
What is Infinite while Loop in Javascript?
Suppose you forget to increment or decrease the value inside the JavaScript while loop code block. In that case, the condition becomes true, and the loop will execute infinite times (also called an infinite). For example:
<!DOCTYPE html> <html> <head> <title> JavaScriptWhileLoop </title> </head> <body> <h1> JavaScriptWhileLoop </h1> <script> var x = 1; while (x < 10) { document.write("<br\> Value From the While Loop is = " + x); } </script> </body> </html>
Here x is always 1, and x is always less than 10. So, it will go on to execute infinite times.
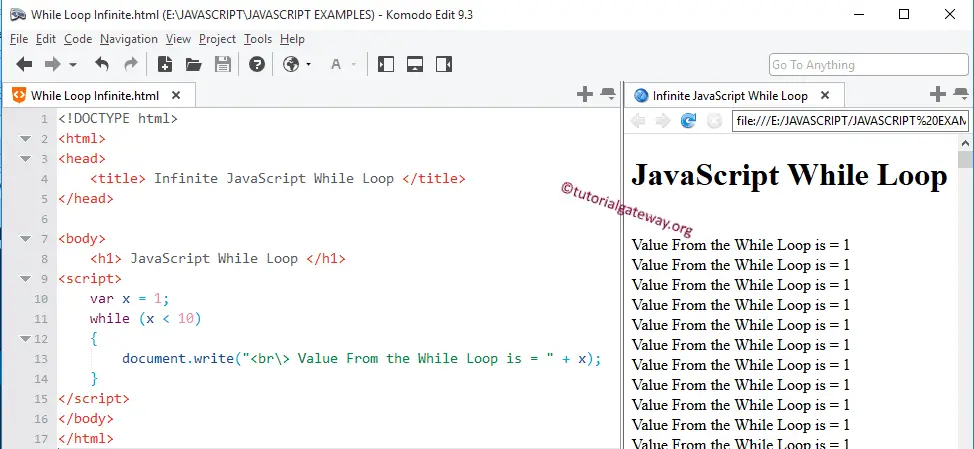
How can we prevent an infinite loop in JavaScript while loop?
You have to ensure that the condition will eventually return to False. Every JavaScript while loop has to use either an increment or decrement operator to increase or decrease the value so that the expression becomes false.
Let us add the increment operator (x++) inside the while loop to the above example.
<!DOCTYPE html> <html> <head> <title> Sample </title> </head> <body> <h1> Example </h1> <script> var x = 1; while (x < 10) { document.write("<br\> Value = " + x); x++; } </script> </body> </html>
Now, when it reaches 10, the condition will fail. Let us see the output.
Example
Value = 1
Value = 2
Value = 3
Value = 4
Value = 5
Value = 6
Value = 7
Value = 8
Value = 9
How to use the break and continue statements within a while loop?
JavaScript allows you to use the break and continue statements within the while loop. The break statement helps to terminate or exit the loop. In contrast, the continue statement will skip the current iteration and move to the next one.
The JavaScript example below demonstrates break and continue statements in the while loop. Here, we initialized the number to 1. Next, we used two if statements to check the conditions.
- If the number equals three, the continue statement will skip printing that number and move to the next iteration.
- If the number equals 6, the break statement will exit from the loop.
<!DOCTYPE html> <html> <head> </head> <body> <script> var number = 1; while (number <= 10) { if(number == 3) { number++; continue; } document.write("<br\>" + number); if (number == 6) { break; } number++; } </script> </body> </html>
1
2
4
5
6
The break statement will terminate the loop. If you use the if statement along with the break, the loop will end when the condition meets.
Yes. We call it the nested while loops.