The JavaScript break Statement is an important keyword used to alter the flow of a program. Loops are used to execute a certain block of code n number of times until the test condition is false. There will be situations where we have to terminate the loop without executing all the statements. In these situations, we can use JavaScript break and Continue statements.
The JavaScript break Statement is very useful for exiting any loop, such as For, While, and Do While. While executing these loops, if the compiler finds the JavaScript break statement inside them, it will stop running the code block and immediately exit from the loop.
For example, we have five lines inside the loop, and we want to exit from the loop when a certain condition is True; otherwise, it has to execute all of them. We can place the JavaScript Break statement inside the If condition in these situations. If the condition is True, then the compiler will execute this one. It means the break statement will completely exit the controller from the loop. Otherwise, it will execute all the lines.
NOTE: The JavaScript break statement is the most important one in Switch Case. Without using Break, the compiler won’t exit from the Switch Case.
JavaScript break Statement Examples
The syntax of the break Statement is as follows:
break;
We would like to share two examples to display the working functionality of the JavaScript Break statement in both For loop and While loop
JavaScript break Statement inside For Loop
This program will use the JavaScript break statement inside the For Loop to exit from the loop iteration.
<!DOCTYPE html> <html> <head> <title> JavaScriptBreak in For Loop </title> </head> <body> <h1> JavaScriptBreak </h1> <script> var i; for (i = 10; i > 0; i--) { if(i == 6) { document.write("<br \>Coming Out of <b> For Loop</b> Where i = " + i); break; } document.write("<br \ ><b>Numbers </b>= " + i); } </script> </body> </html>
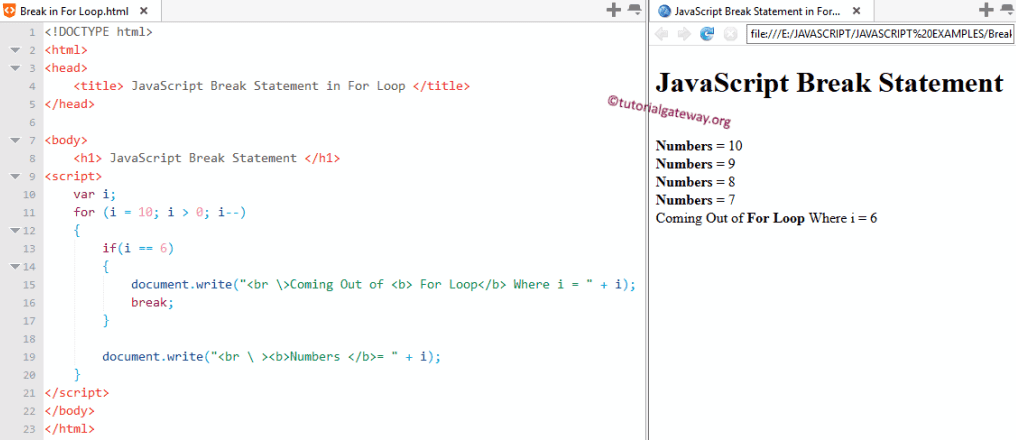
Within the For loop, we initialized the value of i as i =10. And we used the Decrement operator to decrement the value by 1. If you have difficulty understanding the For, please visit our JavaScript For article.
Inside the JavaScript For loop, we placed the If condition to test whether i equals 6. If the condition is false, it will skip the break and print that number as output (In Our case, 10, 9, 8, 7).
If this condition is True, then the break will be executed. Next, the iteration will stop at that number without printing the other lines.
JavaScript break Statement inside While Loop
We will use the break statement inside the While loop to exit from the loop iteration.
<!DOCTYPE html> <html> <head> <title> For Loop </title> </head> <body> <h1> Example </h1> <script> var i = 0; while (i <= 10) { document.write("<br \ ><b>The Value of the Variable </b>= " + i); i++; if(i == 4) { break; } } document.write("<br \>This statement is from Outside While Loop = " + i); </script> </body> </html>
Example
The Value of the Variable = 0
The Value of the Variable = 1
The Value of the Variable = 2
The Value of the Variable = 3
This statement is from Outside While Loop = 4
We initialized the value of i as i = 0 at the beginning of the code. Within the While loop, we check for the condition of whether i is less than or equal to 10 or not. If you have difficulty understanding the While, please visit our JS While. article.
Inside the While, we placed if condition to test whether i equal to 4. If the condition is false, it will skip the break statement and print that number as output (In Our case, 0, 1, 2, 3).
If this condition is True, the JavaScript Break statement will execute. Next, the iteration will stop at that number without printing the other printf function.