The Else If in JavaScript is instrumental when we have to check several conditions. We can also use Nested If to achieve the same. Still, as the number of conditions increases, code complexity will also increase.
JavaScript ElseIf Syntax
The syntax of JavaScript Else If statement is as follows
if (condition1) statements 1 else if (condition2) statements 2 else if (condition3) statements 3 ........... else if (conditionn) statements n else default statements
The JavaScript Else If statement handles multiple expressions effectively by executing them sequentially. It will check for the first condition. If the expression is evaluated to TRUE, it will execute the lines of code present in that block. If the expression is evaluated as FALSE, it will check the Next one (Else If condition) and so on.
There will be some situations in JavaScript Else If statement where condition1, condition2 is TRUE, for example: x = 20, y = 10
Condition1: x > y //TRUE
Condition2: x != y //TRUE
In these situations, code under Condition1 will execute. Because ELSE IF conditions will only execute if its previous IF statement fails.
Else If in JavaScript Example
In this program, we will calculate whether he is eligible for a scholarship or not using the JavaScript Else if statement.
<!DOCTYPE html> <html> <head> <title>ElseIfinJavaScript</title> </head> <h1>ElseIfinJavaScript</h1> <body> <script> var Totalmarks = 380; if (Totalmarks >= 540) { document.write("<b> Congratulations </b>"); document.write("<br\> You are eligible for Full Scholarship " ); } else if(Totalmarks >= 480) { document.write("<b> Congratulations </b>"); document.write("<br\> You are eligible for 50 Percent Scholarship " ); } else if (Totalmarks >= 400) { document.write("<b> Congratulations </b>"); document.write("<br\> You are eligible for 10 Percent Scholarship " ); } else { document.write("<b> You are Not eligible for Scholarship </b>"); document.write("<br\> We are really Sorry for You" ); } </script> </body> </html>
OUTPUT 1: In this JS example, the Totalmarks= 550. First, If condition is TRUE; that’s why the code inside the If Statement displayed as Browser output
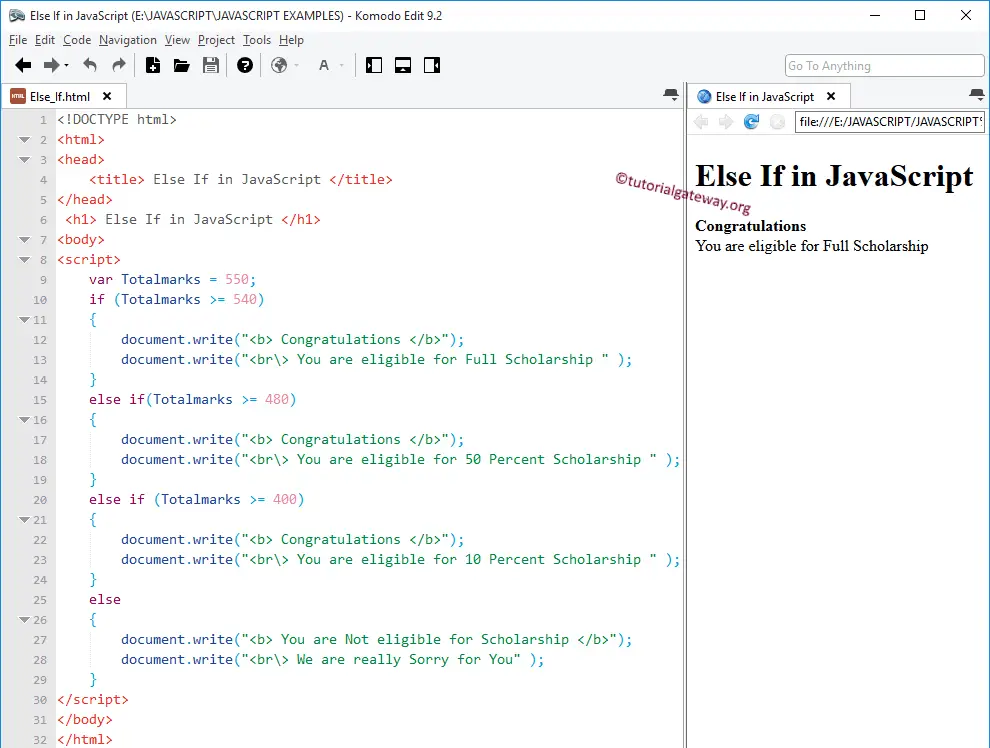
OUTPUT 2: To demonstrate the JavaScript Else if statement, we will change the Totalmarks to 500 means the first IF condition is FALSE. It will check the (Totalmarks>= 480), which is TRUE, to display the lines inside this block. Although the (Total marks>= 400) condition is TRUE, it won’t check that expression.
Congratulations
You are eligible for 50 Percent Scholarship
output 3: We will change Totalmarks to 440 means the first IF condition (Total marks= 480) is FALSE. So, It will check the (Totalmarks>= 400), which is TRUE, to print the code inside this block.
Congratulations
You are eligible for 10 Percent Scholarship
OUTPUT 4: We will change Totalmarks to 380 means all the IF expressions Fail. So, It will print the code inside the else block.
You are Not eligible for Scholarship
We are really Sorry for You