The JavaScript Increment and Decrement Operators useful to increase or decrease the value by 1. For instance, Incremental operator ++ used to increase the existing variable value by 1 (x = x + 1). The decrement operator – – is used to decrease or subtract the existing value by 1 (x = x – 1).
The syntax for both the increment and decrement operators in JavaScript is
- Increment Operator : ++x or x++
- Decrement Operator: –x or x–
Increment and Decrement Operators in JavaScript Example
In this example, we show you the working functionality of Increment and Decrement Operators in the JavaScript programming language.
<!DOCTYPE html> <html> <head> <title> Increment and Decrement Operators in JavaScript </title> </head> <body> <script> var x = 10, y = 20; document.write("<b>----INCREMENT OPERATOR EXAMPLE---- </b>"); document.write("<br \> Value of x : "+ x); //Original Value document.write("<br \> Value of x : "+ x++); // Using increment Operator document.write("<br \> Value of x : "+ x + "<br \>"); //Incremented value document.write("<br \> <b>----DECREMENT OPERATOR EXAMPLE---- </b>"); document.write("<br \> Value of y : "+ y); //Original Value document.write("<br \> Value of y : "+ y--); // using decrement Operator document.write("<br \> Value of y : "+ y); //decremented value </script> </body> </html>
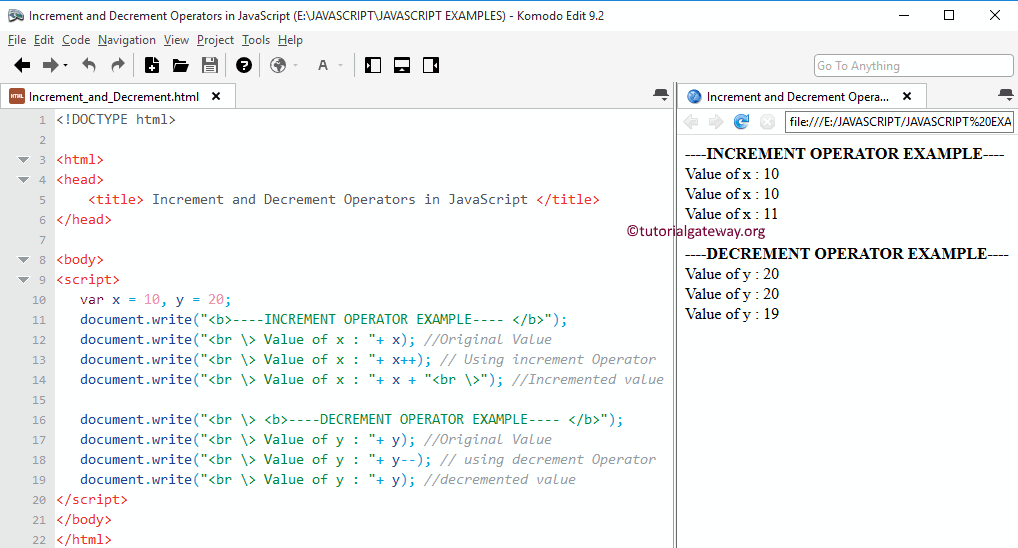
At Line 13 we used increment operator. So the value of the X is returned first (i.e, 10) then X value was incremented by 1.
Line 14: We called the X value again, and it was displaying 11 because the value is updated already. Same with the decrement operator.
JavaScript Prefix and Postfix
If you observe the above syntax, we can assign the JavaScript increment and decrement operators either before operand or after the operand. When ++ or — is used before operand like: ++x, –x then we call it as prefix, if ++ or — is used after the operand like: x++ or x– then we called it as postfix.
Let’s explore the JavaScript prefix and postfix
- ++i (Pre increment): It will increment the value of i even before assigning it to the variable i.
- i++ (Post-increment): The operator returns the variable value first (i.e, i value) then only i value will incremented by 1.
- –i (Pre decrement): It decrements the value of i even before assigning it to the variable i.
- i– (Post decrement): The JavaScript operator returns the variable value first (i.e., i value), then only i value decrements by 1.
JavaScript Prefix and Postfix Example
This example will show you, How to use JavaScript Increment and Decrement Operators as the Prefix and Postfix in JavaScript
<!DOCTYPE html> <html> <head> <title> javascript prefix and Postfix </title> </head> <body> <script> var x = 10, y = 20, a = 5, b= 4; document.write("<b>----PRE INCREMENT OPERATOR EXAMPLE---- </b>"); document.write("<br \> Value of X : " + x); //Original Value document.write("<br \> Value of X : "+ (++x)); // Using increment Operator document.write("<br \> Value of X Incremented: " + x + "<br \>"); //Incremented value document.write("<br \> <b>----POST INCREMENT OPERATOR EXAMPLE---- </b>"); document.write("<br \> Value of Y : "+ y); //Original Value document.write("<br \> Value of Y : "+ y++); // Using increment Operator document.write("<br \> Value of Y Incremented: "+ y + "<br \>"); //Incremented value document.write("<br \> <b>----PRE DECREMENT OPERATOR EXAMPLE---- </b>"); document.write("<br \> Value of A : "+ a); //Original Value document.write("<br \> Value of A : "+ --a); // using decrement Operator document.write("<br \> Value of A Decremented: "+ a + "<br \>"); //decremented value document.write("<br \> <b>----POST DECREMENT OPERATOR EXAMPLE---- </b>"); document.write("<br \> Value of B : "+ b); //Original Value document.write("<br \> Value of B : "+ b--); // using decrement Operator document.write("<br \> Value of B Decremented: "+ b + "<br \>"); //decremented value </script> </body> </html>
----PRE INCREMENT OPERATOR EXAMPLE----
Value of X : 10
Value of X : 11
Value of X Incremented: 11
----POST INCREMENT OPERATOR EXAMPLE----
Value of Y : 20
Value of Y : 20
Value of Y Incremented: 21
----PRE DECREMENT OPERATOR EXAMPLE----
Value of A : 5
Value of A : 4
Value of A Decremented: 4
----POST DECREMENT OPERATOR EXAMPLE----
Value of B : 4
Value of B : 4
Value of B Decremented: 3
Increment and Decrement operators in JavaScript programming used in For Loop, While loop, and Do While loops. Try to learn the idea of the JavaScript prefix and postfix so that you can understand if, for loop, while loop and do-while loop syntax’s easy