The JavaScript substr string function is one of the String functions used to extract the part of a string and return a new string. The syntax of the JavaScript substr function to return results:
String_Object.substr(Start, Length)
The JavaScript substr function will accept two arguments. The first integer value is the index position where the extraction will start, and the second integer value is the length of the text.
JavaScript substr Function Example
The following set of examples will help you understand the substr function, and here, we declared an Str1 variable.
The second statement will extract five characters from the original, starting at index position 2.
Next, We omitted the second argument. It means the JavaScript substr function will start at index position two and end when it reaches last.
Within the following two JavaScript statements (Str5 and Str6), we used Negative and Zero values as the second argument. As we said before, this will return an empty string.
Next, we used negative indexing. The following Str7 statement starts extracting from -3 (index position of 3 from right to left) and ends at the last position.
HINT: If you are using the Negative numbers as an index, it will start looking for items from right to left (Here, -1 is the Last Item, -2 is the Last but one, etc.).
<!DOCTYPE html> <html> <head> <title> SubStrJavaScript </title> </head> <body> <h1> JavaScriptSubStr </h1> <script> var Str1 = "Tutorial Gateway"; var Str2 = Str1.substr(2, 8); var Str3 = Str1.substr(0, 8); var Str4 = Str1.substr(2); var Str5 = Str1.substr(2, -3); var Str6 = Str1.substr(2, 0); var Str7 = Str1.substr(-3); var Str8 = Str1.substr(-3, 2); document.write(Str2 + "<br \>"); document.write(Str3 + "<br \>"); document.write(Str4 + "<br \>"); document.write(Str5 + "<br \>"); document.write(Str6 + "<br \>"); document.write(Str7 + "<br \>"); document.write(Str8 ); </script> </body> </html>
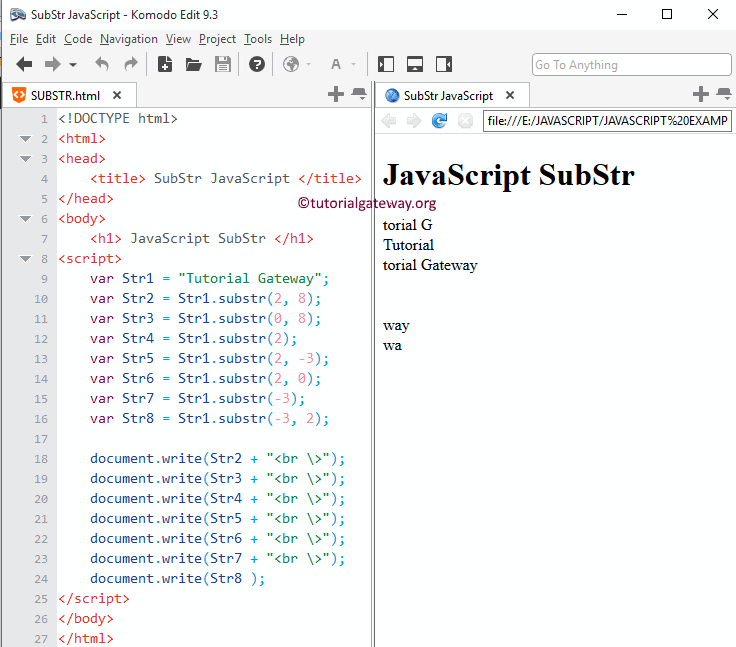