The JavaScript LocaleCompare function is one of the String Functions, which is used to compare two strings and check whether the two are equal or not.
The JavaScript LocaleCompare method will perform a string compare or comparison based on local language settings and returns any of the following three values:
- Returns -1 if String_Object1 sorts before the String_Object2
- Returns +1 if String_Object1 sorts after the String_Object2
- It will return 0 if String_Object1 and String_Object2 are equal
JavaScript String Compare Syntax
The basic syntax of the JavaScript String Compare function is as shown below:
String_Object1.localcompare(String_Object2, locales)
- String_Object1: Please specify the valid string to perform a comparison.
- String_Object2: This argument will check against the String_Object1
- Locales: This argument is optional. Here, you can specify one or an array of languages or local tags. The compare method will consider this argument while comparing String_Object1 and String_Object1.
JavaScript String Compare Example
The following set of JavaScript examples will help you understand the LocaleCompare function to do a string compare. In this example, we declared three variables using the first three statements.
The fourth statement will compare the substring ‘abc’ with ‘def’ and return the output. As we all know, ‘abc’ will come before the ‘def’ while sorting the data that’s why the localeCompare method is returning -1 (Negative one)
The next line differentiates the substring ‘ghi’ from ‘def’ and returns the output. As we know, ‘ghi’ will come after the ‘def’ while sorting the data that’s why the localeCompare method is returning 1 (Positive one)
The last JavaScript statement will check the substring ‘abc’ with ‘abc’ and return the output. As we all know, ‘abc’ is equal to ‘abc’ that’s why the localeCompare method is returning 0 (Zero).
<!DOCTYPE html> <html> <head> <title> JavaScriptStringCompare </title> </head> <body> <h1> JavaScript StringComparison </h1> <script> var Str1 = "abc"; var Str2 = "def"; var Str3 = "ghi"; var Str4 = Str1.localeCompare(Str2); var Str5 = Str3.localeCompare(Str2); var Str6 = Str1.localeCompare("abc"); document.write(Str4 + "<br \>"); document.write(Str5 + "<br \>"); document.write(Str6 + "<br \>"); </script> </body> </html>
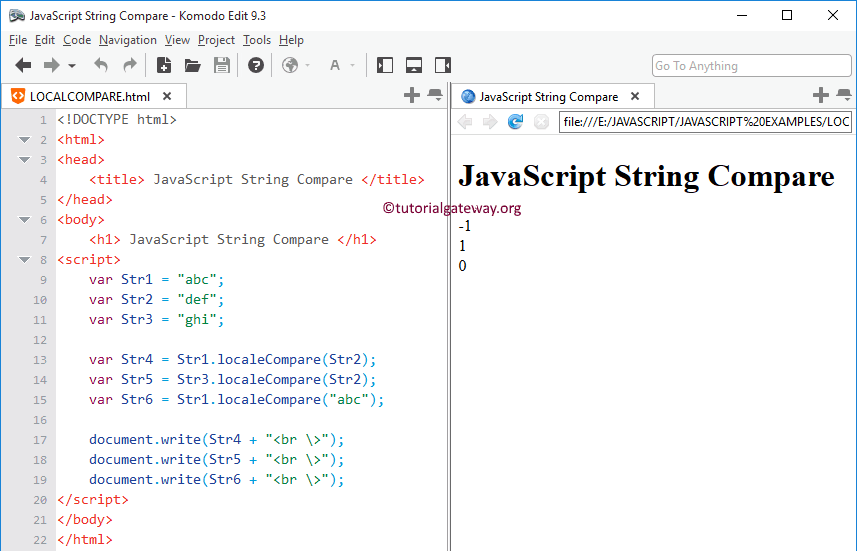