This JavaScript method splits the original string into an array of substrings based on the separator we specified and returns them in an array. Remember, it will not alter the original string.
JavaScript split String Syntax
This method accepts two arguments. And the syntax of the JavaScript split string function is shown below.
String_Object.split(Separator, limit)
- Separator: It can be a simple text or a Regular Expression. For example, empty str, space, ‘,’ or ‘.’
- Limit (integer): Restrict the number of elements written by the array.
If you ignore the first argument, the JavaScript split function breaks each character and assigns them to a string array. If you omit the second index, it starts from the beginning and continues until the end.
JavaScript split String Function Example
This example will help you understand this method. Here, we declared an str variable with a sentence.
The first statement will break the original text into individual characters. Next, we used the empty space as a separator for the split function. So, it will separate the original str1 into an array of words based on white space.
In the fourth statement, we used the second argument to restrict the JavaScript array output to four. Next, we used the substring “abc” as a separator for this method. Here i is the regular expression to perform case insensitive search.
<!DOCTYPE html> <html> <head> <title> Example</title> </head> <body> <h1> JsExample </h1> <script> var Str1 = "We are Abc working in abc company since abc years"; var Str2 = Str1.split(""); var Str3 = Str1.split(" "); var Str4 = Str1.split(" ", 4); var Str5 = Str1.split(/abc/i); var Str6 = Str1.split(/abc/i, 2); document.write(Str2 + "<br \>"); document.write(Str3 + "<br \>"); document.write(Str4 + "<br \>"); document.write(Str5 + "<br \>"); document.write(Str6 + "<br \>"); </script> </body> </html>
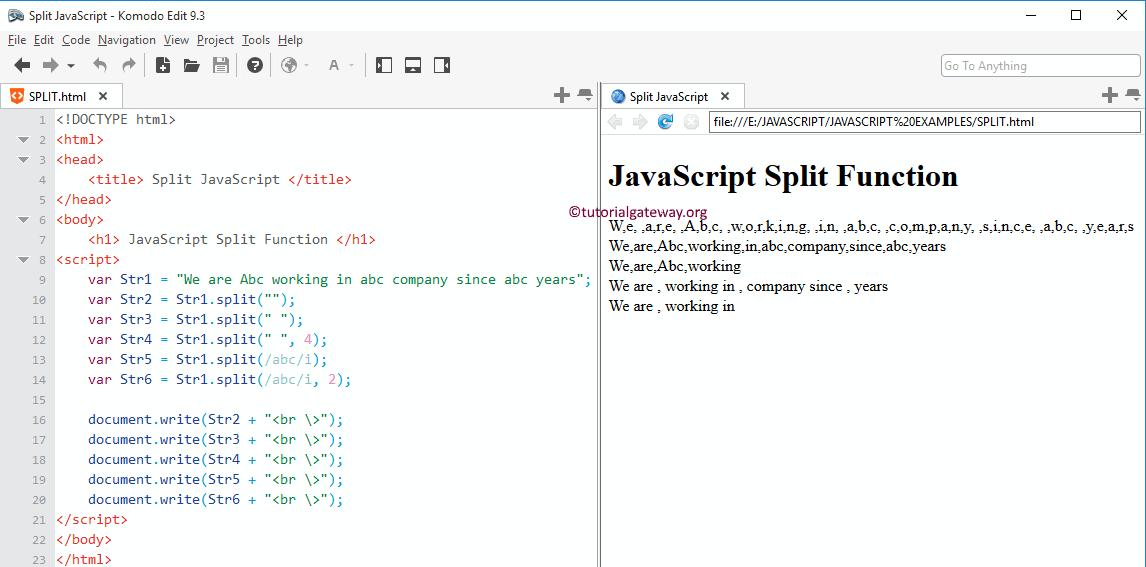