The JavaScript search method is useful for finding and returning the index position of the first occurrence of a specified string in a regular expression. It will return -1 if the defined string is not found. The syntax of the JavaScript search function is
String_Object.search(RegExp)
JavaScript search Example
The following set of examples will help you understand the JavaScript search Function.
The third statement looks for the index position of a substring “Script” and stores the index value in Str3. Remember, You should count the space as One Character.
For Str4, we are looking for a non-existing string “abc” inside Str1. Since the JavaScript Search function doesn’t find the substring, it returns -1 as output.
In the next line, we are looking for “abc” inside Str2. From the below JavaScript image, you can observe that the term abc repeats multiple times. However, the JS function considers the string “ABC” different from “abc”.
Now, let us use i regular expression along with string. This statement performs the case insensitive string search. So, it returns the index position of the first occurrence, i.e., ABC.
<!DOCTYPE html> <html> <head> <title> JavaScriptSearch </title> </head> <body> <h1> JavaScriptSearch </h1> <script> var Str1 = "Learn JavaScript at Tutorial Gateway.org"; var Str2 = "We are ABC working at abc company"; var Str3 = Str1.search("Script"); var Str4 = Str1.search("abc"); // Non existing item var Str5 = Str2.search("abc"); var Str6 = Str2.search(/abc/i); document.write("<b> Index position of Script is:</b> " + Str3); document.write("<br \> <b> Index position of abc is:</b> " + Str4); document.write("<br \> <b> Index position of abc is:</b> " + Str5); document.write("<br \> <b> Index position of abc is:</b> " + Str6); </script> </body> </html>
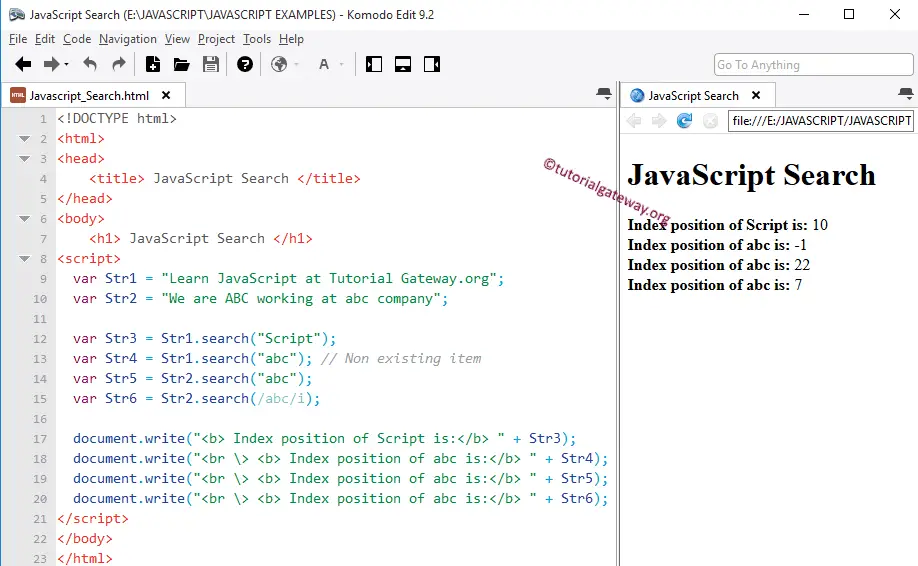