The JavaScript replace function searches for a specified string and updates the searched substring with the newly specified string. The syntax of the JavaScript string replace is
String_Object.replace(String_you_want_to_change, Replacing_String)
- String_Object to perform a search. It will update a portion of a string.
- Substring you want to change: Whatever you place here, the function will change it with a new string.
- Replacing_String: Substring you want to insert into the String_Object.
JavaScript replace String Example
This example will help you understand the String replace function.
The third statement finds the substring ‘abc’ and substitutes it with ‘JavaScript’. If you observe the below screenshot, Although the ‘abc’ substring is repeated twice in Str2, this Function is changing the first occurrence.
In the next line, we used a regular expression to search for the substring ‘abc’ globally. So, this function substitutes every occurrence of the word ‘abc’ with ‘JavaScript‘.
Next, using regular expression gi to perform the case-insensitive search.
<!DOCTYPE html> <html> <head> <title>ReplaceinJavaScript</title> </head> <body> <h1>JavaScriptReplace</h1> <script> var Str1 = "We are ABC working at abc company"; var Str2 = "We are abc working at abc company"; var Str3 = Str2.replace("abc","JavaScript"); var Str4 = Str2.replace(/abc/g,"JavaScript"); var Str5 = Str1.replace("abc","JavaScript"); var Str6 = Str1.replace(/abc/gi,"JavaScript"); document.write(Str3 + "<br \>"); document.write(Str4 + "<br \>"); document.write(Str5 + "<br \>"); document.write(Str6 + "<br \>"); </script> </body> </html>
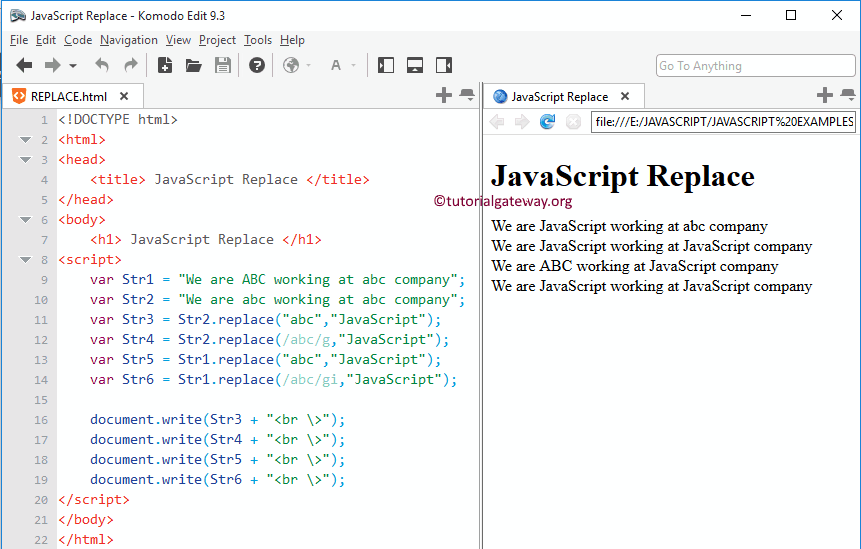