JavaScript Comparison operators are mostly used either in If Statements or Loops. For example, JavaScript Comparison operators are commonly used to check two variables’ relationships. If the relation is true, it will return Boolean TRUE; if the relation is false, it will return Boolean FALSE.
The below table shows all the JavaScript Relational Operators with examples.
JavaScript Comparison Operators | Usage | Description | Example |
---|---|---|---|
> | a > b | a greater than b | 7 > 3 returns True |
< | a < b | a less than b | 7 < 3 returns False |
>= | a >= b | a greater than or equal to b | 7 >= 3 returns True |
<= | a <= b | a less than or equal to b | 7 <= 3 return False |
== | a == b | a equal to b | 7 == “7” returns True |
=== | a === 7 | a Exactly equal to b | 7 === “7” returns False |
!= | a != b | a is not equal to b | 7 != 3 returns True |
!== | a !== b | a is not equal to b, and its Type | 7 !== 3 returns True |
JavaScript Comparison Operators Example
This example helps you to understand the JavaScript Comparison Operators practically. For this example, We are using two variables, a and b, whose values are 9 and 4. Next, we will use these two variables to perform various JavaScript relational operations.
<!DOCTYPE html> <html> <head> <title>JavaScriptComparisonOperators </title> </head> <body> <h1>PerformingComparisonOperations </h1> <script> var a = 12, b = 4; document.write("Result of " + a +" Greater than " + b +" is = " + (a > b) + "<br />"); document.write("Result of " + a +' Greater than or Equal to ' + b +" is = " + (a >= b) + "<br />"); document.write("Result of " + a +' Less than or Equal to ' + b +" is = " + (a <= b) + "<br />"); document.write("Result of " + a +' Less than ' + b +" is = " + (a < b) + "<br />"); document.write("Result of " + a +' Equal to ' + b +" is = " + (a ==b ) + "<br />"); document.write("Result of " + a +' Not Equal to ' + b +" is = " + (a !=b ) + "<br />"); </script> </body> </html>
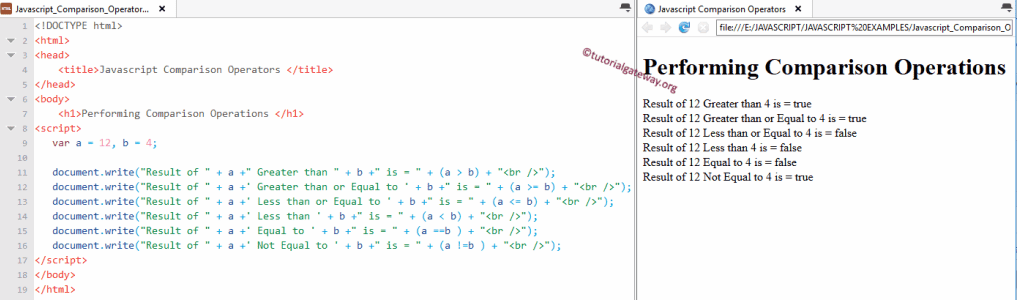
In this JavaScript Comparison Operators example, we assigned 2 integer values, a and b, and we assigned the values 9 and 4 using the below statement.
var a = 12, b = 4;
In the next line, We checked these values against each and every Comparison operator we have.
JavaScript Comparison Operators using If Condition
This example helps you to understand how JavaScript Comparison operators are used in the If Else Statement. For this JavaScript example, we use two variables, x, and y, with values of 10 and 25. We will use the == operator to check whether variable x is equal to y or not.
<!DOCTYPE html> <html> <head> <title>Javascript ComparisonOperators 2</title> </head> <body> <h1> Comparison Operations </h1> <p id = 'result'> Addition </p> <script> var x = 12, y = 24; if (x === y) { document.getElementById("result").innerHTML = x +' is Equal to ' + y; } else { document.getElementById("result").innerHTML = x +' is Not Equal to ' + y; } </script> </body> </html>
Comparison Operations
12 is Not Equal to 24
In this JavaScript relational Operators example, We assigned 2 integer values, x, and y, and we assigned the values 10 and 25 using the below statement.
var x = 12, y = 24;
If Condition
x is exactly equal to y, then the first statement (statement inside the If block) will be executed.
document.getElementById("result").innerHTML = x +' is Equal to ' + y;
If x is not equal to y, the second statement (statement inside the Else block) will be executed.
document.getElementById("result").innerHTML = x +' is Not Equal to ' + y;
Here x value (12) is obviously not equal to 25, so the Second statement will be displayed in the paragraph.
Difference between == , === and !=, !==
This JavaScript Comparison Operators example helps you understand the difference between ==, ===, !=, and !== operators.
- == Check whether the value on the left-hand side is equal to the value on the right-hand side.
- === Check whether the value and the data type on the left-hand side are equal to the value and the data type on the right-hand side.
- In JavaScript Comparison Operators, != check whether the value on the left-hand side is not equal to the value on the right-hand side.
- !== check whether the value and the data type on the left-hand side are not equal to the value and the data type on the right-hand side.
<!DOCTYPE html> <html> <head> <title>Example </title> </head> <body> <h1> Example </h1> <script> document.write("Equal to: " + (12 == "12")); document.write(" <br /> Exactly Equal to: " + (12 === "12")); document.write(" <br /> Not Equal to: " + (12 != "12")); document.write(" <br /> Exactly Not Equal to: " + (12 !== "12")); </script> </body> </html>
Example
Equal to: true
Exactly Equal to: false
Not Equal to: false
Exactly Not Equal to: true