The SQL Server Right Join returns all the records present in the Right table and matches rows from the Left table.
The SQL Server Right join can also call Right outer Join. So, it is optional to use the Outer Keyword. Remember, All the Unmatched rows from the Left table will fill with NULL Values. The syntax of it is as follows:
SELECT Table1.Column(s), Table2.Column(s), FROM Table1 RIGHT OUTER JOIN Table2 ON Table1.Common_Column = Table2.Common_Column --OR We can Simply Write it as SELECT Table1. Column(s), Table2. Column(s), FROM Table1 RIGHT JOIN Table2 ON Table1.Common_Column = Table2.Common_Column
Let us see the visual representation of the SQL Server Right join for a better understanding. From the above image, you can easily understand that it displays all the records present in Table2 and matching records in Table1.
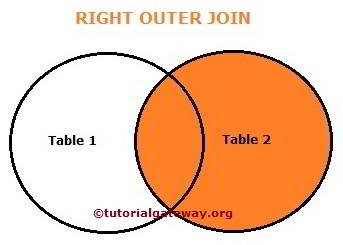
For this SQL Right Outer Join example, we will use two tables in our Database. Data present in the Employee is:
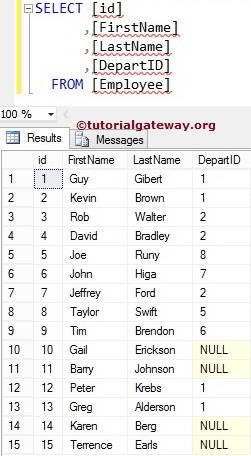
Data present in the SQL Server Department is:
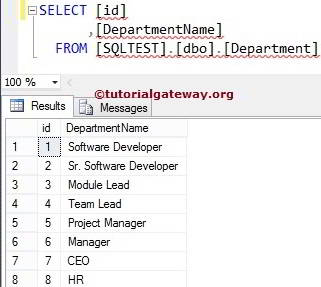
SQL Right Join Select All Columns
The following SQL Right Join Query will display all the columns present in the Department table and matching records from the Employees table.
SELECT * FROM [Employee] RIGHT OUTER JOIN [Department] ON [Employee].[DepartID] = [Department].[id]
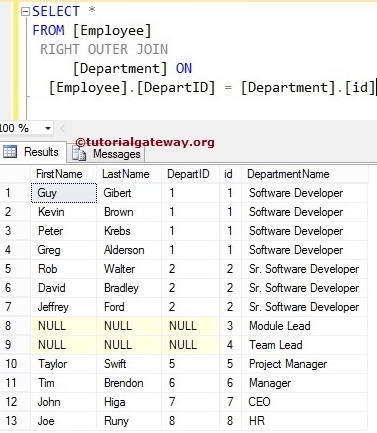
Without Outer keyword
As we said before, it is optional to use an Outer keyword. So let me remove the Outer keyword, and work will SQL server RIGHT JOIN.
SELECT * FROM [Employee] RIGHT OUTER JOIN [Department] ON [Employee].[DepartID] = [Department].[id]
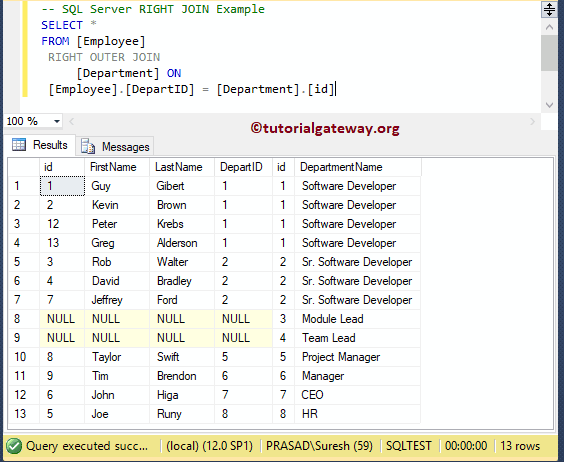
Although the Employee table has 15 records, Right Join displays 13 records. It is because
- Department Id for the 14th and 15th records in the Employee table is NULLS, so there are no matching records in the right table.
- If you observe the 8th and 9th records, they display NULL values. Because in the Employee table, there are no matching records for Department Id 3, and 4 (Module Lead and Team Lead) in the Department table. So they are replaced by NULLS.
NOTE: The [Department ID] column repeats twice, which annoys the user. By selecting individual column names, we can avoid unwanted columns. So, please avoid SELECT * Statements.
SQL Right Outer Join Select Few Columns
Please place the required columns after the SELECT Statement to avoid unwanted columns in Joins.
SELECT [FirstName] ,[LastName] ,[DepartmentName] FROM [Employee] RIGHT JOIN [Department] ON [Employee].[DepartID] = [Department].[id]
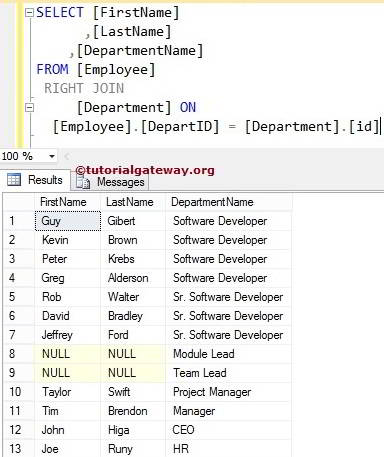
The above Right Join query will excellently work as long as the column names from both Employee and Department tables are different like above. If they have the same Column names, you get an error. Let us see how to solve the issue.
Here, we used the above right outer join query. However, we added the id from the department table as an additional column.
SELECT [FirstName] ,[LastName] ,id ,[DepartmentName] FROM [Employee] RIGHT OUTER JOIN [Department] ON [Employee].[DepartID] = [Department].[id]
As you see, the right join throws an error: Ambiguous column name id. It is because the id column is available in both Employee and department tables. And it doesn’t recognize which column you are claiming.
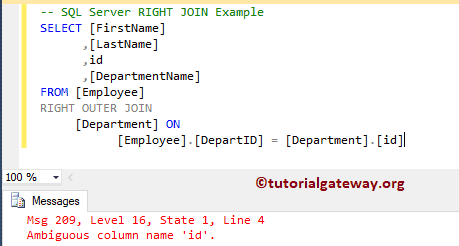
To resolve this kind of concern, practice the table name before the column name. For example, the following right outer join query uses the ALIAS table name before the column names.
By this approach, we can notify the Server that we are looking for the id column belonging to the department table.
We can rewrite the earlier SQL Server right outer join query as:
SELECT Emp.[FirstName] AS [First Name] ,Emp.[LastName] AS [Last Name] ,Dept.id ,Dept.[DepartmentName] AS [Department Name] FROM [Employee] AS Emp RIGHT JOIN [Department] AS Dept ON Emp.[DepartID] = Dept.[id]
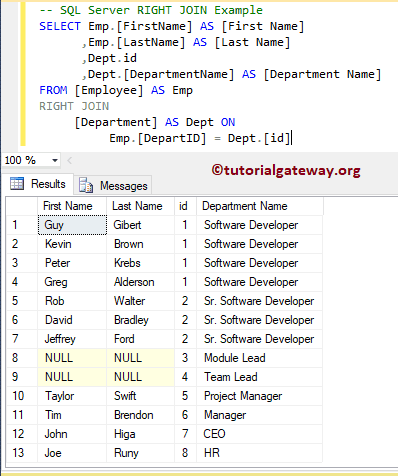
SQL Server Right Join Where Clause
It also allows us to use the Where Clause to restrict the records returned. In this example, we use the WHERE Clause along with the Right Outer Join.
SELECT Emp.[FirstName] AS [First Name] ,Emp.[LastName] AS [Last Name] ,Dept.[DepartmentName] AS [Department Name] FROM [Employee] AS Emp RIGHT JOIN [Department] AS Dept ON Emp.[DepartID] = Dept.[id] WHERE Emp.[FirstName] IS NOT NULL
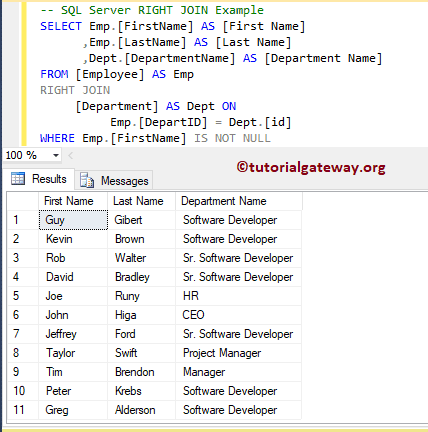
SQL Right Join Order By Clause
It allows us to use Order By Clause to rearrange the order of the records.
SELECT Emp.[FirstName] AS [First Name] ,Emp.[LastName] AS [Last Name] ,Dept.[DepartmentName] AS [Department Name] FROM [Employee] AS Emp RIGHT JOIN [Department] AS Dept ON Emp.[DepartID] = Dept.[id] ORDER BY [FirstName] ASC
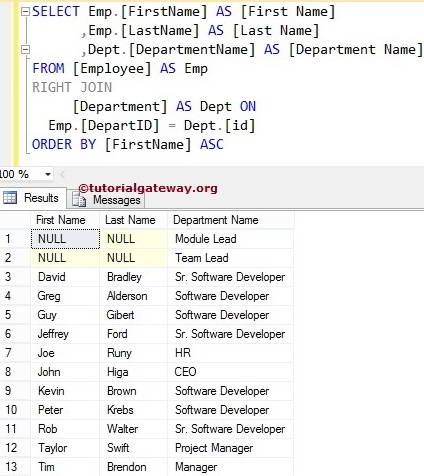