Write a Python program to right rotate a Numpy Array by n times or positions. In this Python example, we used the negative numbers to slice the array from the right side to right rotate and combined the two slices using a numpy concatenate method.
import numpy as np rtArray = np.array([10, 15, 25, 35, 67, 89, 97, 122, 175]) rtRotate = int(input("Enter Position to Right Rotate Array Elements = ")) print('Original Array Elements Before Right Rotating') print(rtArray) arr = np.concatenate((rtArray[-rtRotate:], rtArray[:-rtRotate]), axis = 0) print('\nFinal Array Elements After Right Rotating') print(arr)
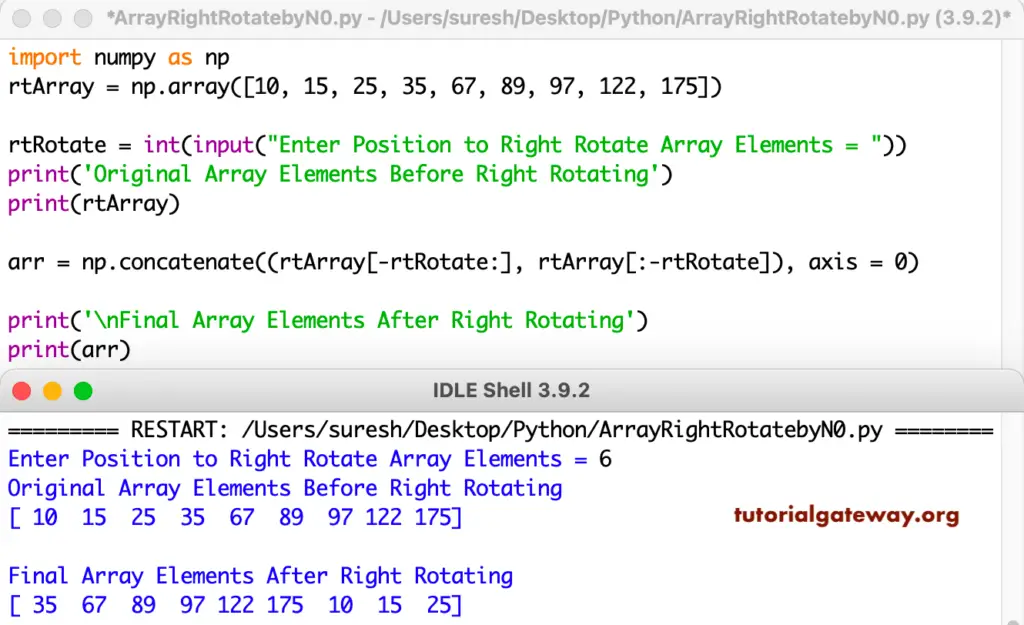
This Python program uses the nested for loops to right rotate the Numpy array items by the user given number n or position.
import numpy as np rtArray = np.array([4, 6, 8, 11, 15, 29, 44, 77, 99]) rtRotate = int(input("Enter Position to Right Rotate Array Elements = ")) print('Original Array Elements Before Right Rotating') print(rtArray) length = rtArray.size - 1 for i in range(rtRotate): lastValue = rtArray[length] for j in range(length, -1, -1): rtArray[j] = rtArray[j - 1] rtArray[0] = lastValue print('\nFinal Array Elements After Right Rotating') print(rtArray)
Enter Position to Right Rotate Array Elements = 4
Original Array Elements Before Right Rotating
[ 4 6 8 11 15 29 44 77 99]
Final Array Elements After Right Rotating
[29 44 77 99 4 6 8 11 15]
In this Python example, the RightRotateArray function will rotate the array items to the right hand side, and printRightArrayItems prints the numpy array items.
import numpy as np def printRightArrayItems(rtArray): for i in rtArray: print(i, end = ' ') def RightRotateArray(rtArray, rtRotate): length = rtArray.size - 1 for i in range(rtRotate): lastValue = rtArray[length] for j in range(length, -1, -1): rtArray[j] = rtArray[j - 1] rtArray[0] = lastValue rtArray = np.random.randint(11, 110, size = 10) rtRotate = int(input("\nEnter Position to Right Rotate Array Elements = ")) print('Original Array Elements Before Right Rotating') printRightArrayItems(rtArray) RightRotateArray(rtArray, rtRotate) print('\nFinal Array Elements After Right Rotating') printRightArrayItems(rtArray)
Enter Position to Right Rotate Array Elements = 3
Original Array Elements Before Right Rotating
16 63 31 106 18 51 61 35 106 72
Final Array Elements After Right Rotating
35 106 72 16 63 31 106 18 51 61
Python program to right rotate a Numpy Array by n using a while loop.
import numpy as np def printRightArrayItems(rtArray): i = 0 while i < len(rtArray): print(rtArray[i], end = ' ') i = i + 1 def RightRotateArray(rtArray, rtRotate): length = rtArray.size - 1 i = 0 while(i < rtRotate): lastValue = rtArray[length] j = length while(j >= 0): rtArray[j] = rtArray[j - 1] j = j - 1 rtArray[0] = lastValue i = i + 1 rtArray = np.random.randint(90, 350, size = 15) rtRotate = int(input("\nEnter Position to Right Rotate Array Elements = ")) print('Original Array Elements Before Right Rotating') printRightArrayItems(rtArray) RightRotateArray(rtArray, rtRotate) print('\nFinal Array Elements After Right Rotating') printRightArrayItems(rtArray)
Enter Position to Right Rotate Array Elements = 5
Original Array Elements Before Right Rotating
291 249 100 283 189 101 341 333 316 344 285 291 234 336 211
Final Array Elements After Right Rotating
285 291 234 336 211 291 249 100 283 189 101 341 333 316 344