Write a Python program to remove punctuations from a string. We declared a string of possible punctuation characters and used for loop to iterate the given text.
In this example, the if statement checks each character against punctuations, and if it is not found, the char will assign to a new string, which removes the punctuations.
punctuations = '''`~!@#$%^&*()-_=+{}[]\|;:'",.<>?''' orgStr = "Hi!!, Welcome, Tutorial-Gateway?" newStr = "" for char in orgStr: if char not in punctuations: newStr = newStr + char print("\nThe Original String Before Removing Punctuations") print(orgStr) print("\nThe Final String After Removing Punctuations") print(newStr)
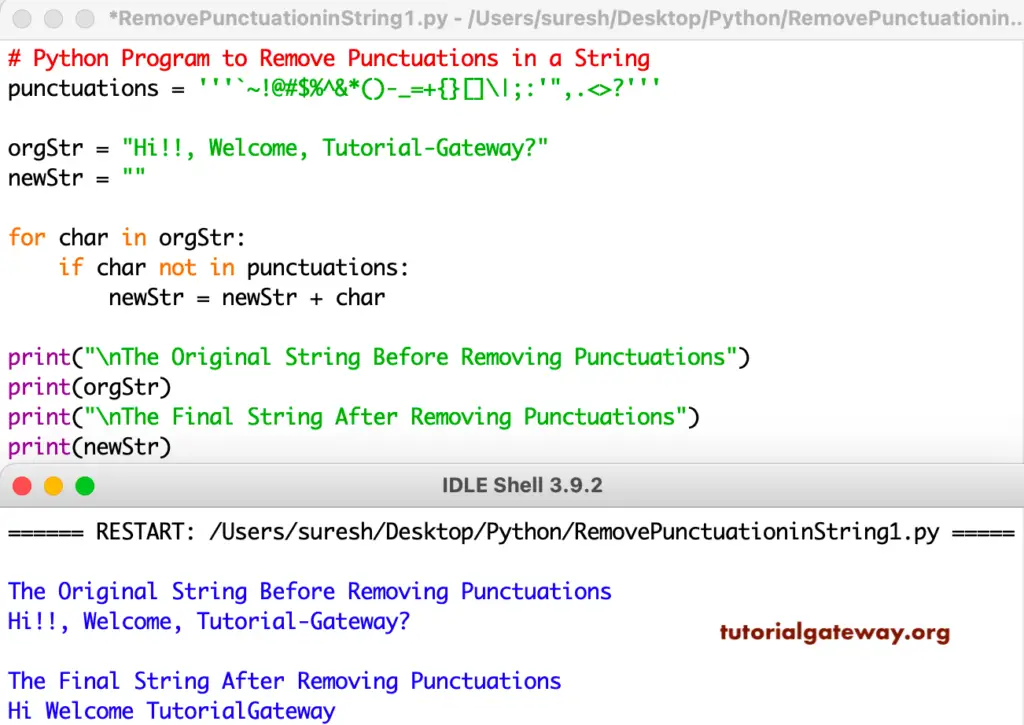
This Python program allows the user to enter a string and removes the punctuation from it.
orgStr = input("Please Enter Any String = ") punctuations = '''`~!@#$%^&*()-_=+{}[]\|;:'",.<>?''' newStr = "" for char in orgStr: if char not in punctuations: newStr = newStr + char print("\nBefore Removing") print(orgStr) print("\nAfter Removing") print(newStr)
Please Enter Any String = Learn@#&^%$# Python<>? Programs?
Before Removing
Learn@#&^%$# Python<>? Programs?
After Removing
Learn Python Programs
The below Python program helps to remove punctuations from a string using a while loop.
orgStr = input("Please Enter Any Text = ") punctuations = '''`~!@#$%^&*()-_=+{}[]\|;:'",.<>?''' newStr = "" i = 0 while i < len(orgStr): if orgStr[i] not in punctuations: newStr = newStr + orgStr[i] i = i + 1 print("\nBefore Removing") print(orgStr) print("\nTAfter Removing") print(newStr)
Please Enter Text = hi!!@ tutorial @@#&^ gateway {}\ followers
Before Removing
hi!!@ tutorial @@#&^ gateway {}\ followers
After Removing
hi tutorial gateway followers