Write a Python Program to remove or delete an Item from Tuple. In Python, we can’t delete an item from a tuple. Instead, we have to assign it to a new Tuple. In this example, we used tuple slicing and concatenation to remove the tuple item. The first one, numTuple[:3] + numTuple[4:] removes the third tuple item.
# Remove an Item from Tuple numTuple = (9, 11, 22, 45, 67, 89, 15, 25, 19) print("Tuple Items = ", numTuple) numTuple = numTuple[:3] + numTuple[4:] print("After Removing 4th Tuple Item = ", numTuple) numTuple = numTuple[:5] + numTuple[7:] print("After Removing 5th and 6th Tuple Item = ", numTuple)
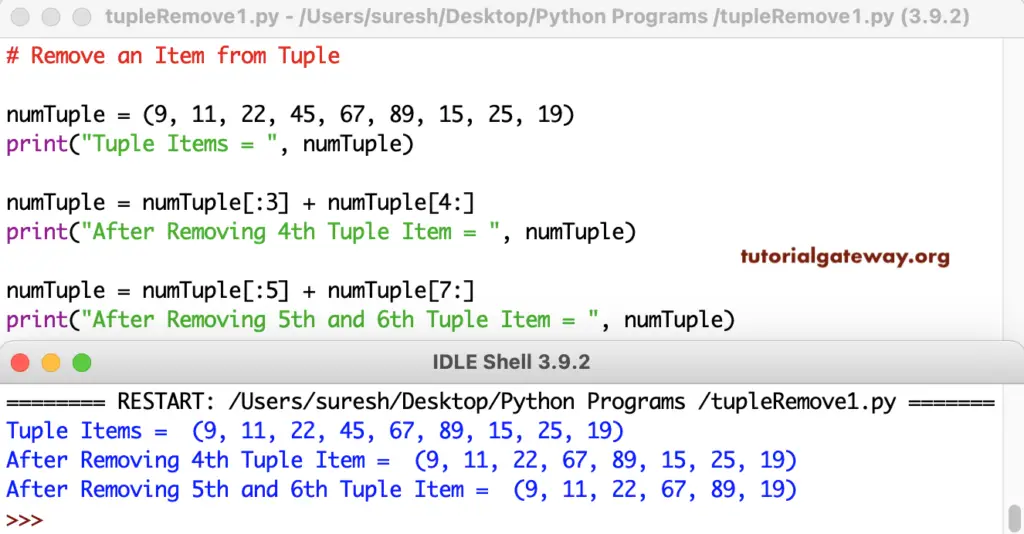
The other option in Python is to convert the Tuple to the list and use the remove function, not remove the list item. Next, convert back to Tuple.
# Remove an Item from Tuple numTuple = (2, 22, 33, 44, 5, 66, 77) print("Tuple Items = ", numTuple) numList = list(numTuple) numList.remove(44) numTuple1 = tuple(numList) print("After Removing 3rd Tuple Item = ", numTuple1)
Tuple Items = (2, 22, 33, 44, 5, 66, 77)
After Removing 3rd Tuple Item = (2, 22, 33, 5, 66, 77)