This blog post shows how to write a Python Program to Print Triangle Pattern of Numbers, stars, and Alphabets (characters) using for loop, while loop, and functions with an example.
Python Program to Print Triangle Star Pattern
This program accepts the user input rows and uses the nested for loop to print the triangle pattern of stars. The first for loop iterates from 0 to rows, and the nested loop starts at 0 and traverses up to i + 1 to print stars.
rows = int(input("Enter Rows = ")) for i in range(rows): for j in range(i + 1): print('*', end = ' ') print()
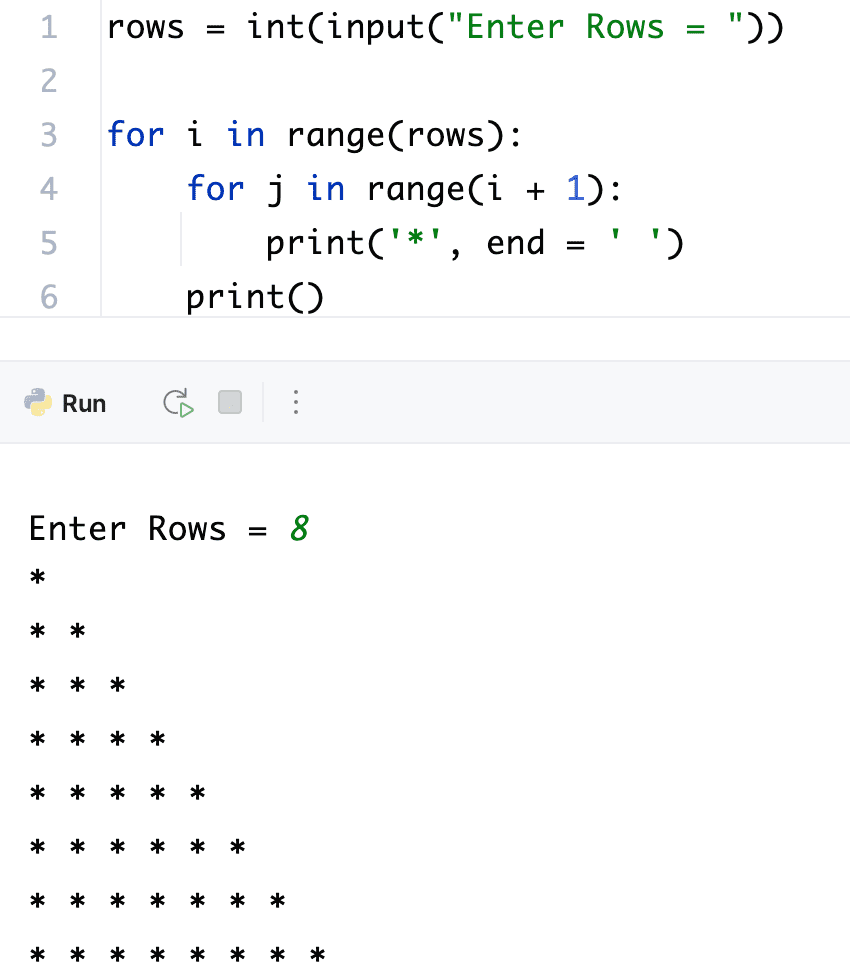
In this program, we use the while loop to print the Triangle pattern of stars.
rows = int(input("Enter Rows = ")) i = 0 while i < rows: j = 0 while j <= i: print('*', end = ' ') j += 1 print() i += 1
Enter Rows = 6
*
* *
* * *
* * * *
* * * * *
* * * * * *
In this Python program, we have created a function that accepts the rows, and the user entered symbol (instead of a star) as a parameter and prints the Triangle pattern.
def patTri(rows, ch): for i in range(rows): for j in range(i + 1): print('%c' % ch, end=' ') print() rows = int(input("Enter Rows = ")) ch = input("Enter Character = ") patTri(rows, ch)
Enter Rows = 7
Enter Character = $
$
$ $
$ $ $
$ $ $ $
$ $ $ $ $
$ $ $ $ $ $
$ $ $ $ $ $ $
Downward Triangle Star Pattern
For more information >> Click Here.
r = int(input("Enter Rows = ")) for i in range(r, 0, -1): for j in range(i): print('*', end = ' ') print()
Output
Enter Rows = 7
* * * * * * *
* * * * * *
* * * * *
* * * *
* * *
* *
*
Python Program to Print Left Pascal Triangle of Stars Pattern
For more information >> Click Here.
def loopLogic(i, r): for l in range(i, r): print(end=' ') for m in range(1, i + 1): print('*', end=' ') print() r = int(input("Enter Rows = ")) for i in range(1, r + 1): loopLogic(i, r) for i in range(r - 1, 0, -1): loopLogic(i, r)
Output
Enter Rows = 5
*
* *
* * *
* * * *
* * * * *
* * * *
* * *
* *
*
Hollow Left Pascals Triangle of Stars
For more information >> Click Here.
r = int(input("Enter Rows = ")) for i in range(1, r + 1): for j in range(r, i, -1): print(end = ' ') for k in range(1, i + 1): if k == 1 or k == i: print('*', end = '') else: print(end = ' ') print() for i in range(1, r + 1): for j in range(1, i + 1): print(end = ' ') for k in range(r - 1, i - 1, -1): if k == r - 1 or k == i: print('*', end = '') else: print(end = ' ') print()
Output
Enter Rows = 6
*
**
* *
* *
* *
* *
* *
* *
* *
**
*
Right Pascals Triangle of Stars
For more information >> Click Here.
def loopLogic(i, r): for j in range(i): print('*', end=' ') print() r = int(input("Enter Rows = ")) for i in range(0, r): loopLogic(i, r) for i in range(r, -1, -1): loopLogic(i, r)
Output
Enter Rows = 5
*
* *
* * *
* * * *
* * * * *
* * * *
* * *
* *
*
Hollow Right Pascals Triangle of Stars
For more information >> Click Here.
r = int(input("Enter Rows = ")) for i in range(1, r + 1): for j in range(1, i + 1): if j == 1 or j == i: print('*', end = '') else: print(end = ' ') print() for i in range(1, r + 1): for j in range(r - 1, i - 1, -1): if j == r - 1 or j == i or i == r: print('*', end = '') else: print(end = ' ') for k in range(1, i): print(end = ' ') print()
Output
Enter Rows = 6
*
**
* *
* *
* *
* *
* *
* *
* *
**
*
Python Program to Print Triangle Numbers Pattern
This section covers the different types of triangle patterns filled with numbers. For more information >> Click Here.
r = int(input("Enter Rows = ")) for i in range(1, r + 1): for j in range(r, i, -1): print(end = ' ') for k in range(1, i + 1): print(k, end = ' ') print()
Output
Enter Rows = 5
1
1 2
1 2 3
1 2 3 4
1 2 3 4 5
Triangle of Numbers in Reverse Pattern
For more information >> Click Here.
r = int(input("Enter Rows = ")) for i in range(r, 0, -1): for j in range(1, i): print(end = ' ') for k in range(i, r + 1): print(k, end = ' ') print()
Output
Enter Rows = 7
7
6 7
5 6 7
4 5 6 7
3 4 5 6 7
2 3 4 5 6 7
1 2 3 4 5 6 7
Downward Triangle of Mirrored Numbers Pattern
For more information >> Click Here.
r = int(input("Enter Rows = ")) for i in range(1, r + 1): for j in range(i, r + 1): print(j, end = ' ') for k in range(r - 1, i - 1, -1): print(k, end = ' ') print()
Output
Enter Rows = 9
1 2 3 4 5 6 7 8 9 8 7 6 5 4 3 2 1
2 3 4 5 6 7 8 9 8 7 6 5 4 3 2
3 4 5 6 7 8 9 8 7 6 5 4 3
4 5 6 7 8 9 8 7 6 5 4
5 6 7 8 9 8 7 6 5
6 7 8 9 8 7 6
7 8 9 8 7
8 9 8
9
Python Program to Print Floyd’s Triangle
For more information >> Click Here.
r = int(input("Enter Rows = ")) n = 1 for i in range(1, r + 1): for j in range(1, i + 1): print(n, end = ' ') n = n + 1 print()
Output
Enter Rows = 6
1
2 3
4 5 6
7 8 9 10
11 12 13 14 15
16 17 18 19 20 21
Inverted Triangle Numbers Pattern
For more information >> Click Here.
r = int(input("Enter Rows = ")) for i in range(1, r + 1): for j in range(1, i): print(end = ' ') for k in range(1, r - i + 2): print(k, end = ' ') print()
Output
Enter Rows = 9
1 2 3 4 5 6 7 8 9
1 2 3 4 5 6 7 8
1 2 3 4 5 6 7
1 2 3 4 5 6
1 2 3 4 5
1 2 3 4
1 2 3
1 2
1
Triangle of Mirrored Numbers Pattern
For more information >> Click Here.
r = int(input("Enter Rows = ")) for i in range(1, r + 1): for j in range(r, i, -1): print(end = ' ') for k in range(1, i + 1): print(k, end = '') for l in range(i - 1, 0, -1): print(l, end = '') print()
Output
Enter Rows = 8
1
121
12321
1234321
123454321
12345654321
1234567654321
123456787654321
Left Pascal Triangle of Numbers
For more information >> Click Here.
def loopLogic(i, r): for j in range(i, r): print(end=' ') for k in range(1, i + 1): print(k, end=' ') print() r = int(input("Enter Rows = ")) for i in range(1, r + 1): loopLogic(i, r) for i in range(r - 1, 0, -1): loopLogic(i, r)
Output
Enter Rows = 7
1
1 2
1 2 3
1 2 3 4
1 2 3 4 5
1 2 3 4 5 6
1 2 3 4 5 6 7
1 2 3 4 5 6
1 2 3 4 5
1 2 3 4
1 2 3
1 2
1
Right Pascal Triangle of Numbers
For more information >> Click Here.
def loopLogic(i, r): for j in range(1, i + 1): print(j, end=' ') print() r = int(input("Enter Rows = ")) for i in range(1, r + 1): loopLogic(i, r) for i in range(r - 1, 0, -1): loopLogic(i, r)
Output
Enter Rows = 7
1
1 2
1 2 3
1 2 3 4
1 2 3 4 5
1 2 3 4 5 6
1 2 3 4 5 6 7
1 2 3 4 5 6
1 2 3 4 5
1 2 3 4
1 2 3
1 2
1
Right Pascals Triangle of Multiplication Numbers Pattern
For more information >> Click Here.
def loopLogic(i, r): for j in range(1, i + 1): print(j * i, end=' ') print() r = int(input("Enter Rows = ")) for i in range(1, r + 1): loopLogic(i, r) for i in range(r - 1, 0, -1): loopLogic(i, r)
Output
Enter Rows = 6
1
2 4
3 6 9
4 8 12 16
5 10 15 20 25
6 12 18 24 30 36
5 10 15 20 25
4 8 12 16
3 6 9
2 4
1
Right Pascals Triangle of Mirrored Numbers Pattern
For more information >> Click Here.
def loopLogic(i, r): for j in range(i, r + 1): print(j, end=' ') for k in range(r - 1, i - 1, -1): print(k, end=' ') print() r = int(input("Enter Rows = ")) for i in range(r, 0, -1): loopLogic(i, r) for i in range(2, r + 1): loopLogic(i, r)
Output
Enter Rows = 7
7
6 7 6
5 6 7 6 5
4 5 6 7 6 5 4
3 4 5 6 7 6 5 4 3
2 3 4 5 6 7 6 5 4 3 2
1 2 3 4 5 6 7 6 5 4 3 2 1
2 3 4 5 6 7 6 5 4 3 2
3 4 5 6 7 6 5 4 3
4 5 6 7 6 5 4
5 6 7 6 5
6 7 6
7
Python Program to Print Triangle Pattern of Alphabets
This section shows the various triangle patterns of alphabets or characters. For more information >> Click Here.
r = int(input("Enter Rows = ")) alp = 65 for i in range(r): for j in range(r, i, -1): print(end = ' ') for k in range(i + 1): print('%c' %(alp + k), end = ' ') print()
Output
Enter Rows = 7
A
A B
A B C
A B C D
A B C D E
A B C D E F
A B C D E F G
Downward Triangle Mirrored Alphabets Pattern
For more information >> Click Here.
r = int(input("Enter Rows = ")) alp = 65 for i in range(r): for j in range(i, r): print('%c' %(alp + j), end = ' ') for k in range(r - 2, i - 1, -1): print('%c' %(alp + k), end = ' ') print()
Output
Enter Rows = 7
A B C D E F G F E D C B A
B C D E F G F E D C B
C D E F G F E D C
D E F G F E D
E F G F E
F G F
G
Inverted Triangle Alphabets Pattern
For more information >> Click Here.
r = int(input("Enter Rows = ")) alp = 65 for i in range(0, r): for j in range(r - 1, i - 1, -1): print('%c' %(alp + j), end = ' ') print()
Output
Enter Rows = 9
I H G F E D C B A
I H G F E D C B
I H G F E D C
I H G F E D
I H G F E
I H G F
I H G
I H
I
Triangle of Same Alphabets Pattern
For more information >> Click Here.
r = int(input("Enter Rows = ")) alp = 65 for i in range(r): for j in range(r - 1, i, -1): print(end = ' ') for k in range(i + 1): print('%c' %(alp + i), end = ' ') print()
Output
Enter Rows = 8
A
B B
C C C
D D D D
E E E E E
F F F F F F
G G G G G G G
H H H H H H H H
Triangle of Mirrored Alphabets Pattern
For more information >> Click Here.
r = int(input("Enter Rows = ")) alp = 65 for i in range(r): for j in range(r - 1, i - 1, -1): print(end = ' ') for k in range(i + 1): print('%c' %(alp + k), end = '') for l in range(i - 1, -1, -1): print('%c' %(alp + l), end = '') print()
Output
Enter Rows = 9
A
ABA
ABCBA
ABCDCBA
ABCDEDCBA
ABCDEFEDCBA
ABCDEFGFEDCBA
ABCDEFGHGFEDCBA
ABCDEFGHIHGFEDCBA
Triangle of Alphabets in Reverse Pattern
For more information >> Click Here.
r = int(input("Enter Rows = ")) alp = 65 for i in range(r - 1, -1, -1): for j in range(i): print(end = ' ') for k in range(i, r): print('%c' %(alp + k), end = ' ') print()
Output
Enter Rows = 11
K
J K
I J K
H I J K
G H I J K
F G H I J K
E F G H I J K
D E F G H I J K
C D E F G H I J K
B C D E F G H I J K
A B C D E F G H I J K
Downward Triangle Alphabets Pattern
For more information >> Click Here.
r = int(input("Enter Rows = ")) alp = 65 for i in range(r, 0, -1): for j in range(i): print('%c' %(alp + j), end = ' ') print()
Output
Enter Rows = 9
A B C D E F G H I
A B C D E F G H
A B C D E F G
A B C D E F
A B C D E
A B C D
A B C
A B
A