Write a Python Program to Print Rectangle Star Pattern using For Loop and While Loop with an example.
Python Program to Print Rectangle Star Pattern using For Loop
This Python program allows user to enter the total number of rows and columns for drawing rectangle. Next, we used Python Nested For Loop to print rectangle of stars.
# Python Program to Print Rectangle Star Pattern rows = int(input("Please Enter the Total Number of Rows : ")) columns = int(input("Please Enter the Total Number of Columns : ")) print("Rectangle Star Pattern") for i in range(rows): for j in range(columns): print('*', end = ' ') print()
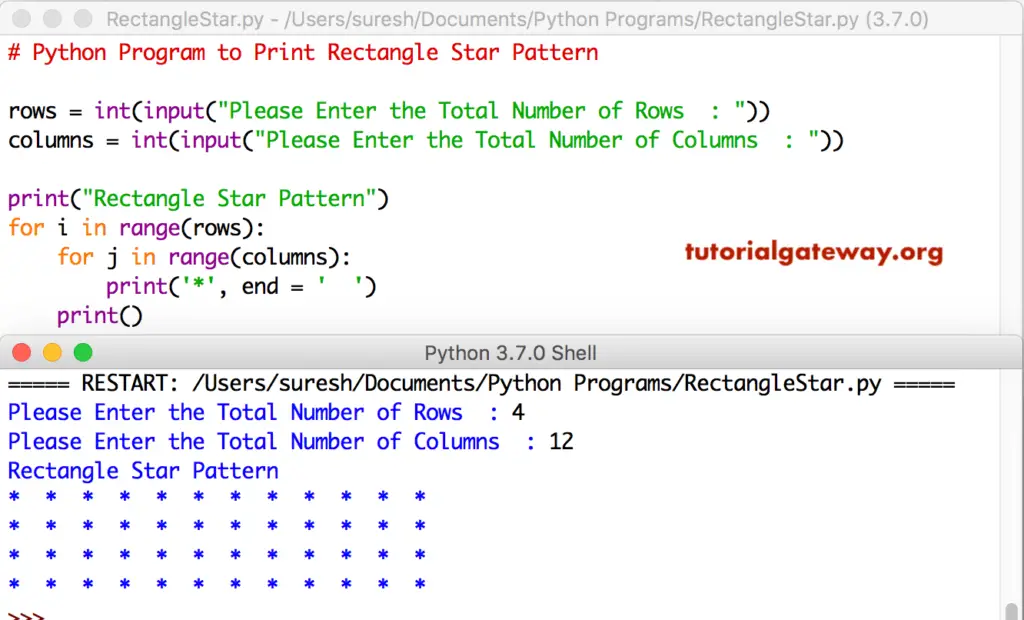
Python Program to Print Rectangle Star Example 2
This Python program allows user to enter his/her own character. Next, it prints the rectangle of the user-specified character.
# Python Program to Print Rectangle Star Pattern rows = int(input("Please Enter the Total Number of Rows : ")) columns = int(input("Please Enter the Total Number of Columns : ")) ch = input("Please Enter any Character : ") print("Rectangle Star Pattern") for i in range(rows): for j in range(columns): print('%c' %ch, end = ' ') print()
Please Enter the Total Number of Rows : 15
Please Enter the Total Number of Columns : 18
Please Enter any Character : $
Rectangle Star Pattern
$ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $
$ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $
$ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $
$ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $
$ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $
$ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $
$ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $
$ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $
$ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $
$ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $
$ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $
$ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $
$ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $
$ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $
$ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $ $
>>>
Python Program to Print Rectangle of Stars using While Loop
This Python rectangle of stars program is the same as the first example. However, we replaced the For Loop with While Loop.
# Python Program to Print Rectangle Star Pattern rows = int(input("Please Enter the Total Number of Rows : ")) columns = int(input("Please Enter the Total Number of Columns : ")) print("Rectangle Star Pattern") i = 0 while(i < rows): j = 0 while(j < columns): print('*', end = ' ') j = j + 1 i = i + 1 print()
Please Enter the Total Number of Rows : 15
Please Enter the Total Number of Columns : 20
Rectangle Star Pattern
* * * * * * * * * * * * * * * * * * * *
* * * * * * * * * * * * * * * * * * * *
* * * * * * * * * * * * * * * * * * * *
* * * * * * * * * * * * * * * * * * * *
* * * * * * * * * * * * * * * * * * * *
* * * * * * * * * * * * * * * * * * * *
* * * * * * * * * * * * * * * * * * * *
* * * * * * * * * * * * * * * * * * * *
* * * * * * * * * * * * * * * * * * * *
* * * * * * * * * * * * * * * * * * * *
* * * * * * * * * * * * * * * * * * * *
* * * * * * * * * * * * * * * * * * * *
* * * * * * * * * * * * * * * * * * * *
* * * * * * * * * * * * * * * * * * * *
* * * * * * * * * * * * * * * * * * * *
>>>