Write a Python program to print pyramid alphabets pattern using for loop.
rows = int(input("Enter Alphabets Pyramid Pattern Rows = ")) print("====The Pyramid of Alphabets Pattern====") alphabet = 64 for i in range(1, rows + 1): for j in range(1, rows - i + 1): print(end = ' ') for k in range(i, 0, -1): print('%c' %(alphabet + k), end = '') for l in range(2, i + 1): print('%c' %(alphabet + l), end = '') print()
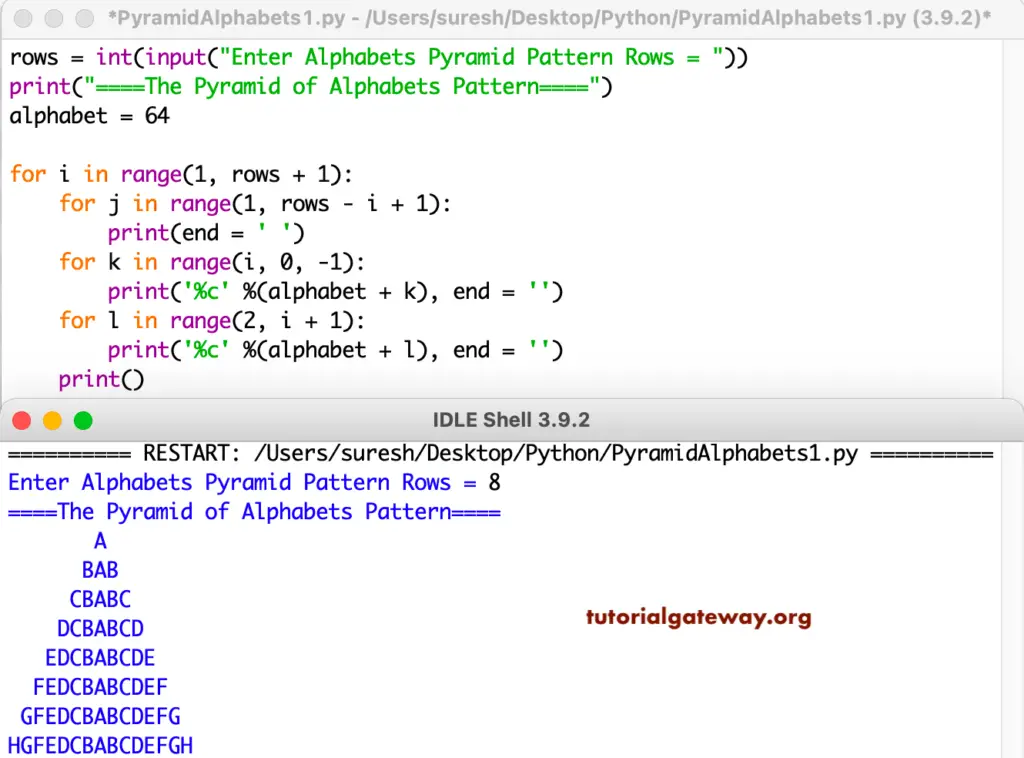
This Python example program prints the pyramid pattern of alphabets using a while loop.
rows = int(input("Enter Rows = ")) print("========") alphabet = 64 i = 1 while(i <= rows): j = 1 while(j <= rows - i): print(end = ' ') j = j + 1 k = i while(k > 0): print('%c' %(alphabet + k), end = '') k = k - 1 l = 2 while(l <= i): print('%c' %(alphabet + l), end = '') l = l + 1 print() i = i + 1
Enter Rows = 12
========
A
BAB
CBABC
DCBABCD
EDCBABCDE
FEDCBABCDEF
GFEDCBABCDEFG
HGFEDCBABCDEFGH
IHGFEDCBABCDEFGHI
JIHGFEDCBABCDEFGHIJ
KJIHGFEDCBABCDEFGHIJK
LKJIHGFEDCBABCDEFGHIJKL