Write a Python program to print Pronic numbers from 1 to 100 or within a range using a while loop. This Python example accepts the minimum and maximum values and displays the Pronic numbers within that range.
def checkPronic(Number): i = 0 flag = 0 while i <= Number: if Number == i * (i + 1): flag = 1 break i = i + 1 return flag minPro = int(input("Enter the Minimum Pronic Number = ")) maxPro = int(input("Enter the Maximum Pronic Number = ")) print("\nThe List of Pronic Numbers from {0} and {1}".format(minPro, maxPro)) for i in range(minPro, maxPro): if(checkPronic(i) == 1): print(i, end = ' ')
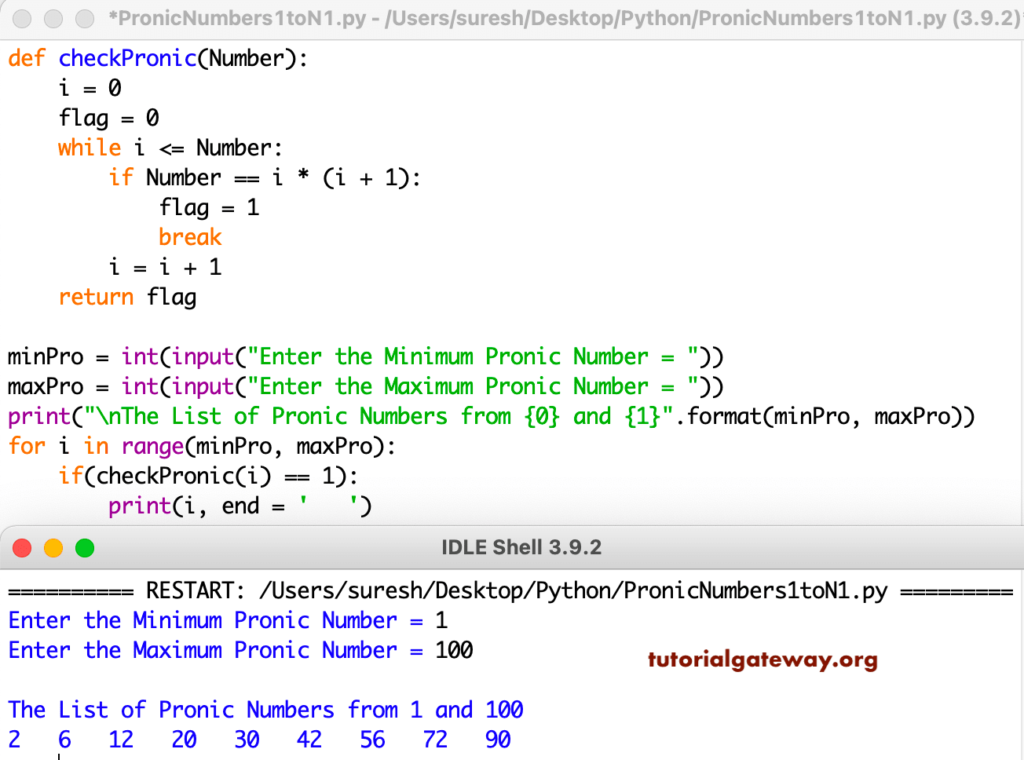
Python program to print Pronic numbers from 1 to n using the for loop.
def checkPronic(Number): flag = 0 for i in range(Number + 1): if Number == i * (i + 1): flag = 1 break return flag minPro = int(input("Enter the Minimum = ")) maxPro = int(input("Enter the Maximum = ")) print("\nThe List of Pronic Numbers from {0} and {1}".format(minPro, maxPro)) for i in range(minPro, maxPro): if(checkPronic(i) == 1): print(i, end = ' ')
Enter the Minimum = 200
Enter the Maximum = 400
The List of Pronic Numbers from 200 and 400
210 240 272 306 342 380