Write a Python Program to Print Positive Numbers in a Tuple using for loop range. The if statement (if(posTuple[i] >= 0)) checks whether the Tuple item is greater than or equals to zero. If True, print that Positive Tuple number.
# Tuple Positive Numbers posTuple = (3, -8, 6, 4, -88, -11, 13, 19) print("Positive Tuple Items = ", posTuple) print("\nThe Positive Numbers in posTuple Tuple are:") for i in range(len(posTuple)): if(posTuple[i] >= 0): print(posTuple[i], end = " ")
Positive Tuple Items = (3, -8, 6, 4, -88, -11, 13, 19)
The Positive Numbers in posTuple Tuple are:
3 6 4 13 19
Python Program to Print Positive Numbers in Tuple using the For Loop.
In this Python Positive numbers example, we used the for loop (for potup in posTuple) to iterate the actual tuple posTuple items.
# Tuple Positive Numbers posTuple = (25, 32, -14, 17, -6, -1, 98, 11, -9, 0) print("Positive Tuple Items = ", posTuple) print("\nThe Positive Numbers in this posTuple Tuple are:") for potup in posTuple: if(potup >= 0): print(potup, end = " ")
Positive Tuple Items = (25, 32, -14, 17, -6, -1, 98, 11, -9, 0)
The Positive Numbers in this posTuple Tuple are:
25 32 17 98 11 0
Python Program to return or display Positive Numbers in Tuple using the While Loop.
# Tuple Positive Numbers posTuple = (25, -11, -22, 17, 0, -9, 43, 17, -10) print("Positive Tuple Items = ", posTuple) i = 0 print("\nThe Positive Numbers in posTuple Tuple are:") while (i < len(posTuple)): if(posTuple[i] >= 0): print(posTuple[i], end = " ") i = i + 1
Positive Tuple Items = (25, -11, -22, 17, 0, -9, 43, 17, -10)
The Positive Numbers in posTuple Tuple are:
25 17 0 43 17
In this Python Tuple example, we created a function (tuplePositiveNumbers(posTuple)) that finds and prints the Positive numbers.
# Tuple Positive Numbers def tuplePositiveNumbers(posTuple): for potup in posTuple: if(potup >= 0): print(potup, end = " ") posTuple = (19, -32, -17, 98, 44, -12, 0, 78, -2, 4, -5) print("Positive Tuple Items = ", posTuple) print("\nThe Positive Numbers in this posTuple Tuple are:") tuplePositiveNumbers(posTuple)
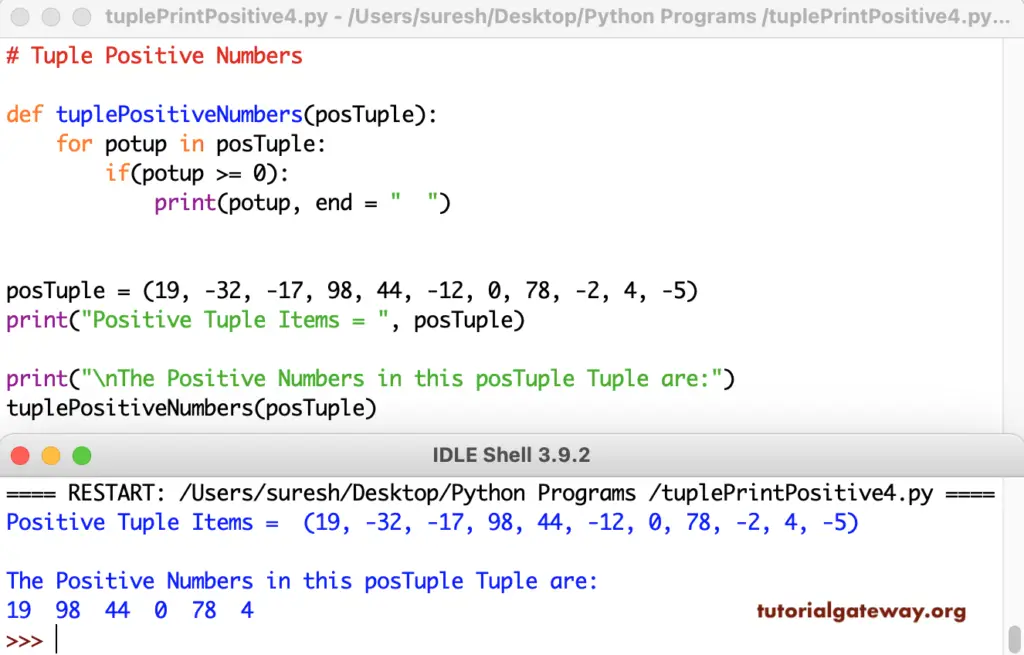