Write a Python Program to Print Positive Numbers or Items in a Set. The if statement (if(posVal >= 0)) inside the for loop (for posVal in PositiveSet) checks the Set item is greater than or equals to zero. If True, print that Positive Set number.
# Set Positive Numbers PositiveSet = {7, -8, -11, 4, -85, 14, -22, 78, 11} print("Positive Set Items = ", PositiveSet) print("\nThe Positive Numbers in this PositiveSet Set are:") for posVal in PositiveSet: if(posVal >= 0): print(posVal, end = " ")
Print Positive Numbers in a Python Set output
Positive Set Items = {4, 7, -22, 11, -85, 14, 78, -11, -8}
The Positive Numbers in this PositiveSet Set are:
4 7 11 14 78
In this Python Program, we allow you to enter the set items and Print the Positive Numbers in Set.
# Set Positive Numbers positiveSet = set() number = int(input("Enter the Total Positive Set Items = ")) for i in range(1, number + 1): value = int(input("Enter the %d Set Item = " %i)) positiveSet.add(value) print("Positive Set Items = ", positiveSet) print("\nThe Positive Numbers in this positiveSet Set are:") for posVal in positiveSet: if(posVal >= 0): print(posVal, end = " ")
Python Print Positive Set items output
Enter the Total Positive Set Items = 4
Enter the 1 Set Item = -32
Enter the 2 Set Item = 23
Enter the 3 Set Item = -99
Enter the 4 Set Item = 77
Positive Set Items = {-32, 77, -99, 23}
The Positive Numbers in this positiveSet Set are:
77 23
In this Python Set example, we created a setPositiveNumbers function that finds and prints the Positive numbers.
# Set Positive Numbers def setPositiveNumbers(positiveSet): for posVal in positiveSet: if(posVal >= 0): print(posVal, end = " ") positiveSet = set() number = int(input("Enter the Total Positive Set Items = ")) for i in range(1, number + 1): value = int(input("Enter the %d Set Item = " %i)) positiveSet.add(value) print("Positive Set Items = ", positiveSet) print("\nThe Positive Numbers in this positiveSet Set are:") setPositiveNumbers(positiveSet)
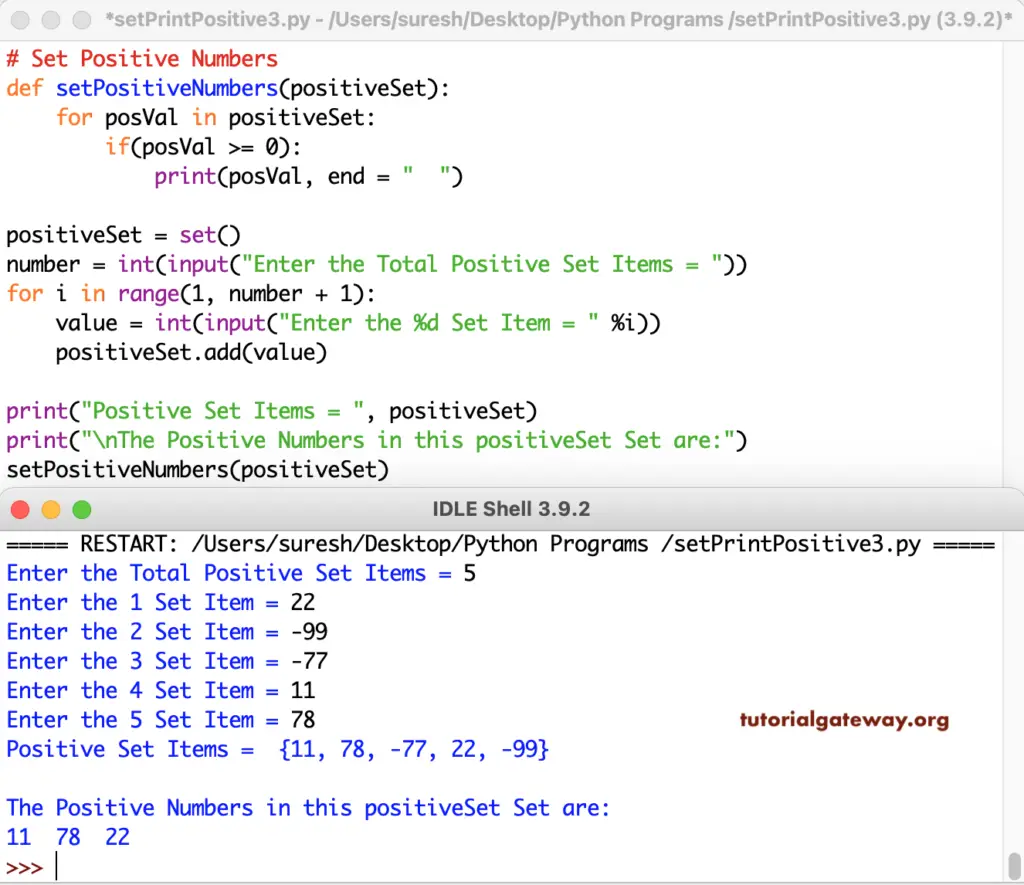