Write a Python program to print positive numbers in a range or from 1 to n. This example allows minimum and maximum numbers and prints the positive numbers within that range.
minimum = int(input("Enter the Minimum Number = ")) maximum = int(input("Enter the Maximum Number = ")) print("\nAll Positive Numbers from {0} and {1}".format(minimum, maximum)) for num in range(minimum, maximum + 1): if num >= 0: print(num, end = ' ')
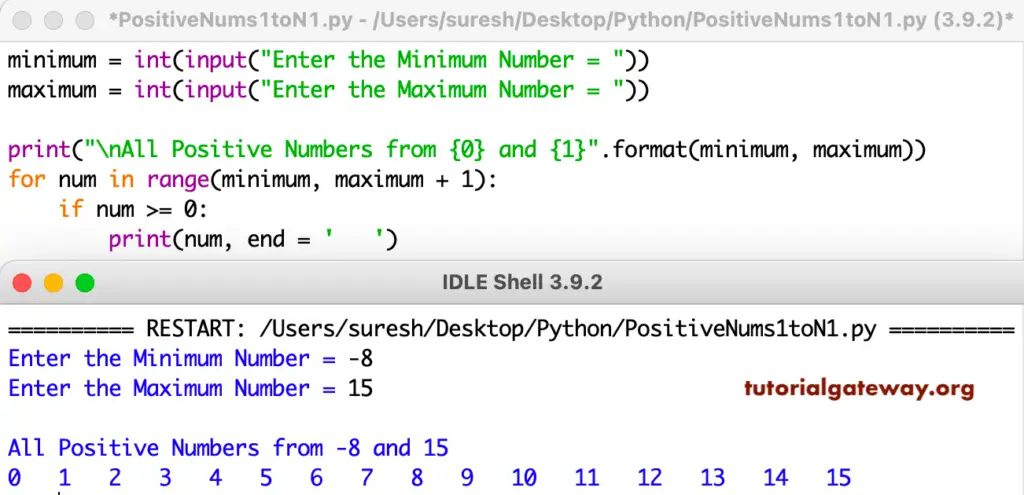
This program helps to print positive numbers in a range using a while loop.
minimum = int(input("Enter the Minimum Number = ")) maximum = int(input("Enter the Maximum Number = ")) print("\nAll Positive Numbers from {0} and {1}".format(minimum, maximum)) while minimum <= maximum: if minimum >= 0: print(minimum, end = ' ') minimum = minimum + 1
Enter the Minimum Number = -50
Enter the Maximum Number = 39
All Positive Numbers from -50 and 39
0 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39
In this example, the positiveNumbers function accepts two integers and prints the positive numbers between them.
def positiveNumbers(x, y): for num in range(x, y + 1): if num >= 0: print(num, end = ' ') minimum = int(input("Enter the Minimum Number = ")) maximum = int(input("Enter the Maximum Number = ")) print("\nAll Positive Numbers from {0} and {1}".format(minimum, maximum)) positiveNumbers(minimum, maximum)
Enter the Minimum Number = -90
Enter the Maximum Number = 50
All Positive Numbers from -90 and 50
0 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50