Write a Python Program to Print Plus Star Pattern using a for loop. In this Python example, both the for loop will traverse from 1 to 2 * rows. The if statement checks whether i or j equals rows and, if valid, print stars.
# Python Program to Print Plus Star Pattern rows = int(input("Enter Plus Pattern Rows = ")) print("Plus Star Pattern") for i in range(1, 2 * rows): for j in range(1, 2 * rows): if i == rows or j == rows: print('*', end = '') else: print(' ', end = '') print()
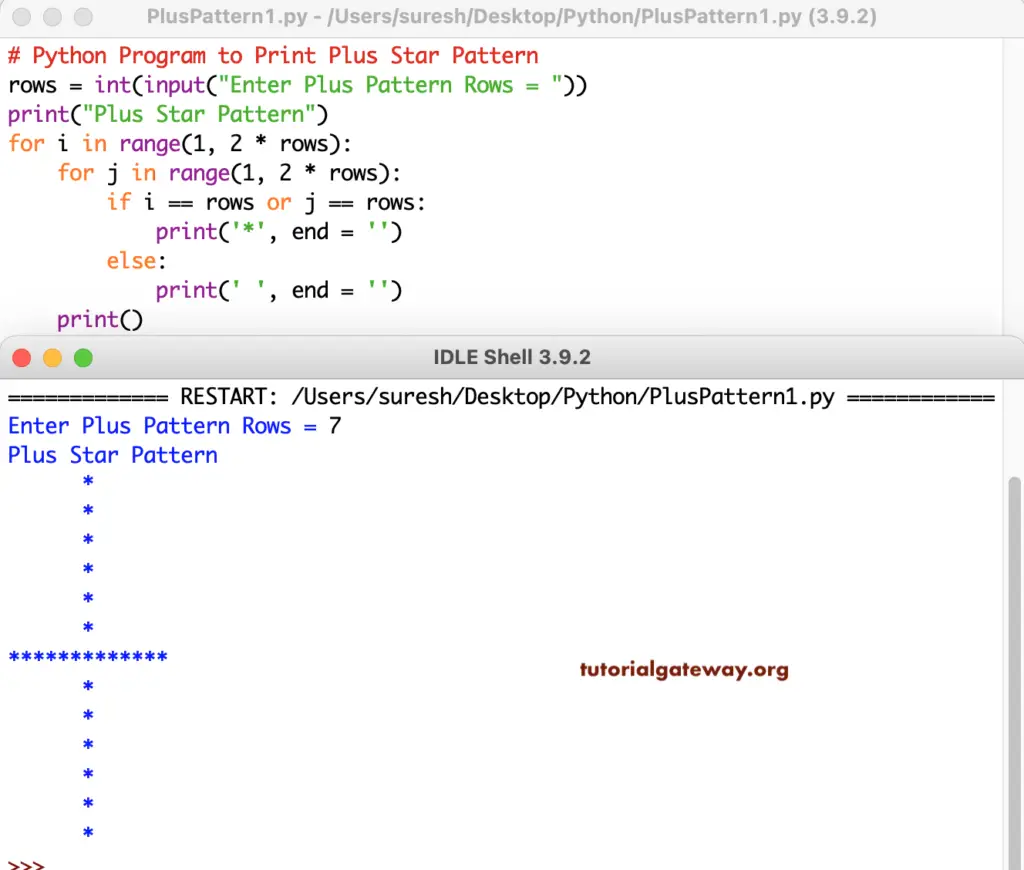
Python Program to Print Plus Star Pattern using a while loop.
# Python Program to Print Plus Star Pattern rows = int(input("Enter Plus Pattern Rows = ")) print("Plus Star Pattern") i = 1 while(i < 2 * rows): j = 1 while(j < 2 * rows): if i == rows or j == rows: print('*', end = '') else: print(' ', end = '') j = j + 1 i = i + 1 print()
Enter Plus Pattern Rows = 7
Plus Star Pattern
*
*
*
*
*
*
*************
*
*
*
*
*
*
>>>
In this Python Program, the plusPattern function prints the Plus Pattern of the given symbol.
# Python Program to Print Plus Star Pattern def plusPattern(rows, ch): for i in range(1, 2 * rows): for j in range(1, 2 * rows): if i == rows or j == rows: print('%c' %ch, end = '') else: print(' ', end = '') print() rows = int(input("Enter Plus Pattern Rows = ")) ch = input("Symbol to use in Plus Pattern = " ) print("Plus Pattern") plusPattern(rows, ch)
Enter Plus Pattern Rows = 8
Symbol to use in Plus Pattern = $
Plus Pattern
$
$
$
$
$
$
$
$$$$$$$$$$$$$$$
$
$
$
$
$
$
$
>>>