Write a Python Program to Print Odd Numbers in a Tuple using for loop range (for i in range(len(oddTuple))). The if statement (if(oddTuple[i] % 2 != 0) checks whether each Tuple item divisible by two not equal to zero. If True, print that Odd number in a Tuple.
# Tuple Odd Numbers oddTuple = (9, 22, 33, 45, 56, 77, 89, 90) print("Odd Tuple Items = ", oddTuple) print("\nThe Odd Numbers in oddTuple Tuple are:") for i in range(len(oddTuple)): if(oddTuple[i] % 2 != 0): print(oddTuple[i], end = " ")
Odd Tuple Items = (9, 22, 33, 45, 56, 77, 89, 90)
The Odd Numbers in oddTuple Tuple are:
9 33 45 77 89
Python Program to Print Odd Numbers in Tuple using the For Loop
In this Python Odd numbers example, we used the for loop (for tup in oddTuple) to iterate the actual tuple items to find the odd numbers.
# Tuple Odd Numbers oddTuple = (19, 98, 17, 23, 56, 77, 88, 99, 111) print("Tuple Items = ", oddTuple) print("\nThe Odd Numbers in this oddTuple Tuple are:") for tup in oddTuple: if(tup % 2 != 0): print(tup, end = " ")
Tuple Items = (19, 98, 17, 23, 56, 77, 88, 99, 111)
The Odd Numbers in this oddTuple Tuple are:
19 17 23 77 99 111
Python Program to return Odd Numbers in Tuple using the While Loop.
# Tuple Odd Numbers oddTuple = (25, 19, 44, 53, 66, 79, 89, 22, 67) print("Odd Tuple Items = ", oddTuple) i = 0 print("\nThe Odd Numbers in oddTuple Tuple are:") while (i < len(oddTuple)): if(oddTuple[i] % 2 != 0): print(oddTuple[i], end = " ") i = i + 1
Odd Tuple Items = (25, 19, 44, 53, 66, 79, 89, 22, 67)
The Odd Numbers in oddTuple Tuple are:
25 19 53 79 89 67
In this Python Tuple example, we created a function (tupleOddNumbers(oddTuple)) that finds and prints the Odd numbers.
# Tuple Odd Numbers def tupleOddNumbers(oddTuple): for tup in oddTuple: if(tup % 2 != 0): print(tup, end = " ") oddTuple = (122, 55, 12, 11, 67, 88, 42, 99, 17, 64) print("Tuple Items = ", oddTuple) print("\nThe Odd Numbers in oddTuple Tuple are:") tupleOddNumbers(oddTuple)
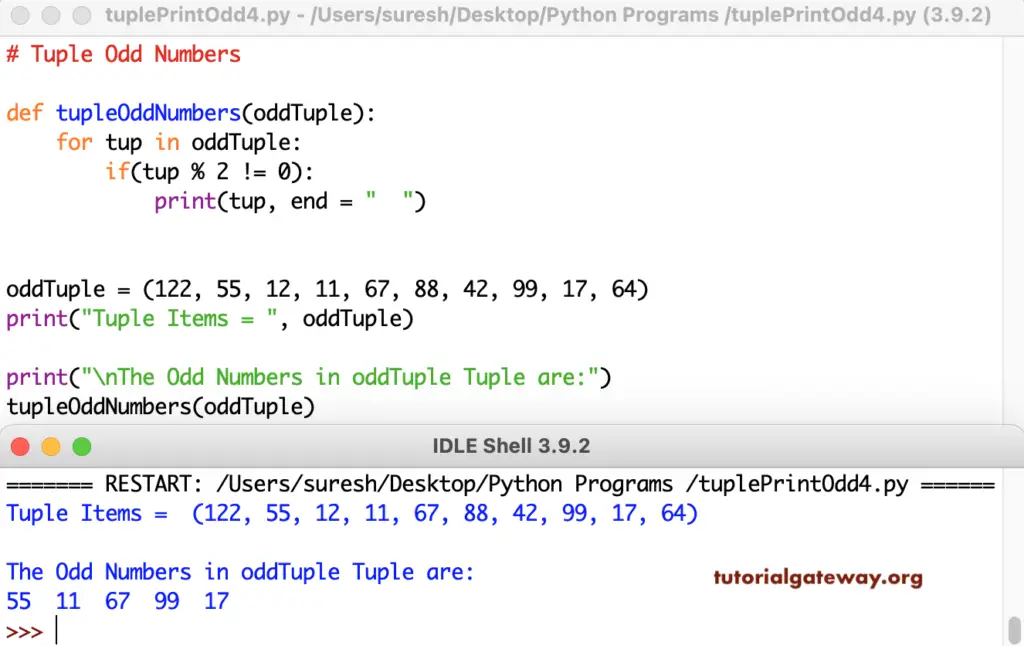