Write a Python Program to Print Negative Numbers in Tuple using for loop range. The if statement (if(negTuple[i] < 0)) checks whether the Tuple item is less than zero. If True, print that Negative Tuple number (print(negTuple[i], end = ” “)).
# Tuple Negative Numbers negTuple = (3, -8, -22, 4, -98, 14, -33, -78, 11) print("Negative Tuple Items = ", negTuple) print("\nThe Negative Numbers in negTuple Tuple are:") for i in range(len(negTuple)): if(negTuple[i] < 0): print(negTuple[i], end = " ")
Negative Tuple Items = (3, -8, -22, 4, -98, 14, -33, -78, 11)
The Negative Numbers in negTuple Tuple are:
-8 -22 -98 -33 -78
Python Program to Print Negative Numbers in Tuple using the For loop.
In this Python Negative numbers example, we used the for loop (for ngtup in negTuple) to iterate the actual tuple items.
# Tuple Negative Numbers negTuple = (25, -14, -28, 17, -61, -21, 89, 17, -48, 0) print("Negative Tuple Items = ", negTuple) print("\nThe Negative Numbers in this negTuple Tuple are:") for ngtup in negTuple: if(ngtup < 0): print(ngtup, end = " ")
Negative Tuple Items = (25, -14, -28, 17, -61, -21, 89, 17, -48, 0)
The Negative Numbers in this negTuple Tuple are:
-14 -28 -61 -21 -48
Python Program to return or display Negative Numbers in Tuple using the While Loop.
# Tuple Negative Numbers negTuple = (11, -22, -45, 67, -98, -87, 0, 16, -120) print("Negative Tuple Items = ", negTuple) i = 0 print("\nThe Negative Numbers in negTuple Tuple are:") while (i < len(negTuple)): if(negTuple[i] < 0): print(negTuple[i], end = " ") i = i + 1
Negative Tuple Items = (11, -22, -45, 67, -98, -87, 0, 16, -120)
The Negative Numbers in negTuple Tuple are:
-22 -45 -98 -87 -120
In this Python Tuple example, we created a function (tupleNegativeNumbers(negTuple)) that finds and prints the Negative numbers.
# Tuple Negative Numbers def tupleNegativeNumbers(negTuple): for potup in negTuple: if(potup < 0): print(potup, end = " ") negTuple = (9, -23, -17, 98, 66, -12, -77, 0, -2, 15) print("Negative Tuple Items = ", negTuple) print("\nThe Negative Numbers in negTuple Tuple are:") tupleNegativeNumbers(negTuple)
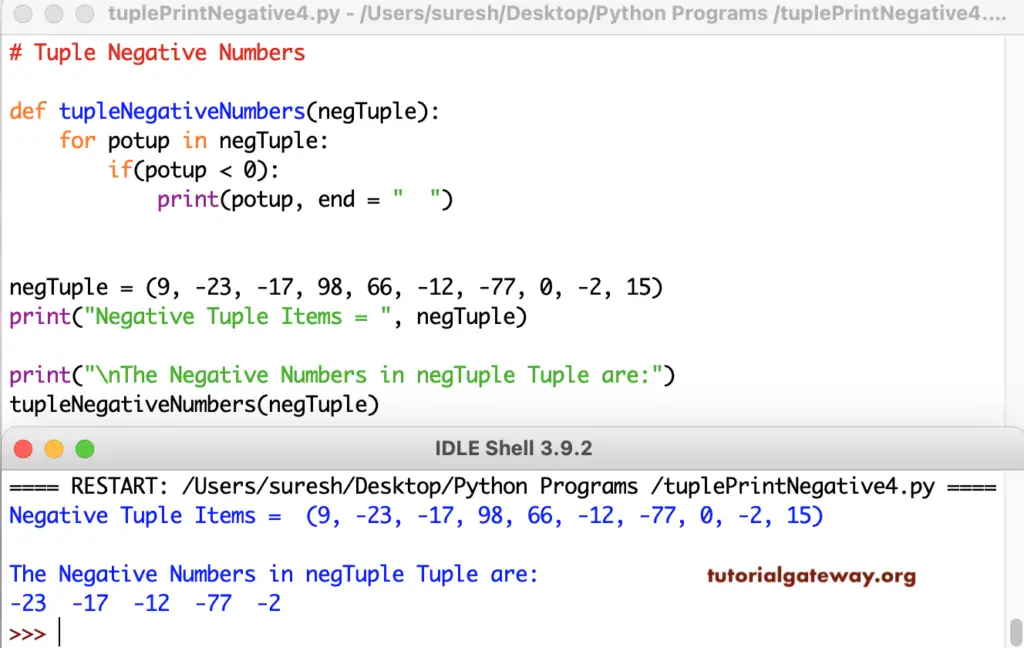