Write a Python Program to Print Negative Numbers in a Set. The if statement (if(negaVal < 0)) inside the for loop (for negaVal in NegativeSet) checks the Set item is less than zero. If True, print that Negative Set number.
# Set Negative Numbers NegativeSet = {6, -22, -33, 78, -88, 15, -55, -66, 17} print("Negative Set Items = ", NegativeSet) print("\nThe Negative Numbers in this NegativeSet Set are:") for negaVal in NegativeSet: if(negaVal < 0): print(negaVal, end = " ")
Print Negative Numbers in a Python Set output
Negative Set Items = {6, -88, -55, -22, 78, 15, 17, -66, -33}
The Negative Numbers in this NegativeSet Set are:
-88 -55 -22 -66 -33
This Python Program allows you to enter the set items and Print the Negative Numbers in Set.
# Set Negative Numbers negativeSet = set() number = int(input("Enter the Total Negative Set Items = ")) for i in range(1, number + 1): value = int(input("Enter the %d Set Item = " %i)) negativeSet.add(value) print("Negative Set Items = ", negativeSet) print("\nThe Negative Numbers in this negativeSet Set are:") for negaVal in negativeSet: if(negaVal < 0): print(negaVal, end = " ")
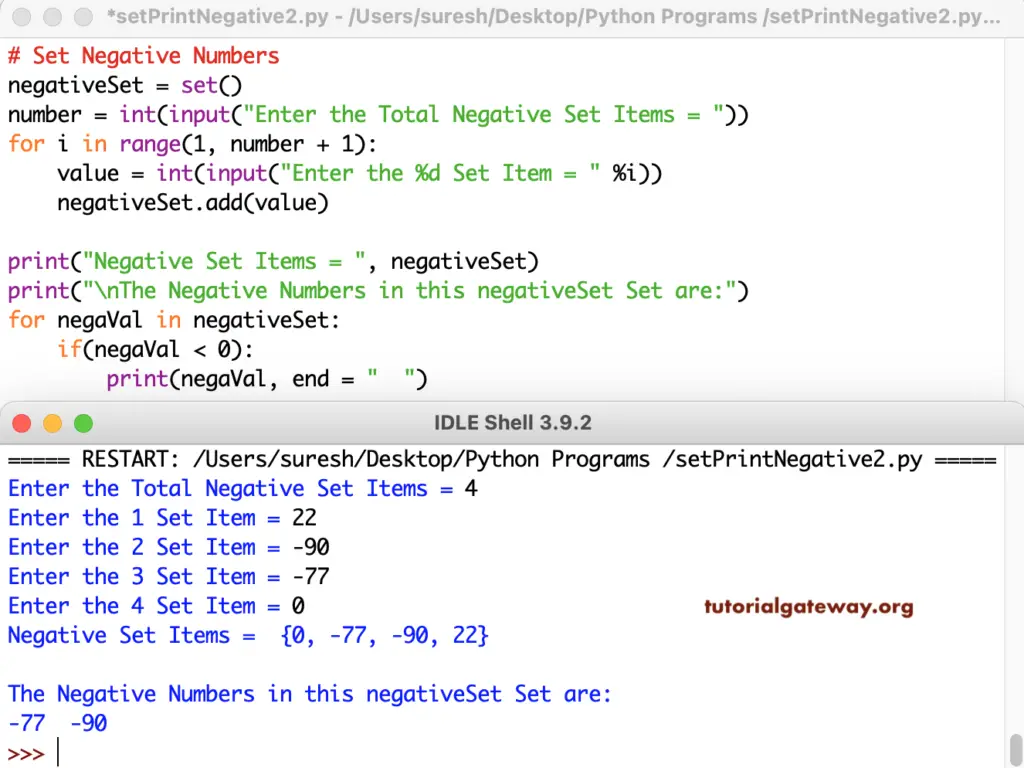
In this Python Set example, we created a setnegativeNumbers function that finds and prints the Negative numbers.
# Tuple Negative Numbers def setnegativeNumbers(negativeSet): for negVal in negativeSet: if(negVal < 0): print(negVal, end = " ") negativeSet = set() number = int(input("Enter the Total Negative Set Items = ")) for i in range(1, number + 1): value = int(input("Enter the %d Set Item = " %i)) negativeSet.add(value) print("Negative Set Items = ", negativeSet) print("\nThe Negative Numbers in this negativeSet Set are:") setnegativeNumbers(negativeSet)
Enter the Total Negative Set Items = 5
Enter the 1 Set Item = 22
Enter the 2 Set Item = -33
Enter the 3 Set Item = -87
Enter the 4 Set Item = 58
Enter the 5 Set Item = -71
Negative Set Items = {-87, 22, -71, 58, -33}
The Negative Numbers in this negativeSet Set are:
-87 -71 -33