Write a Python program to print negative numbers in a range. This example allows start and end numbers and prints the negative numbers within that range.
minimum = int(input("Enter the Minimum Number = ")) maximum = int(input("Enter the Maximum Number = ")) print("\nAll Negative Numbers from {0} and {1}".format(minimum, maximum)) for num in range(minimum, maximum + 1): if num < 0: print(num, end = ' ')
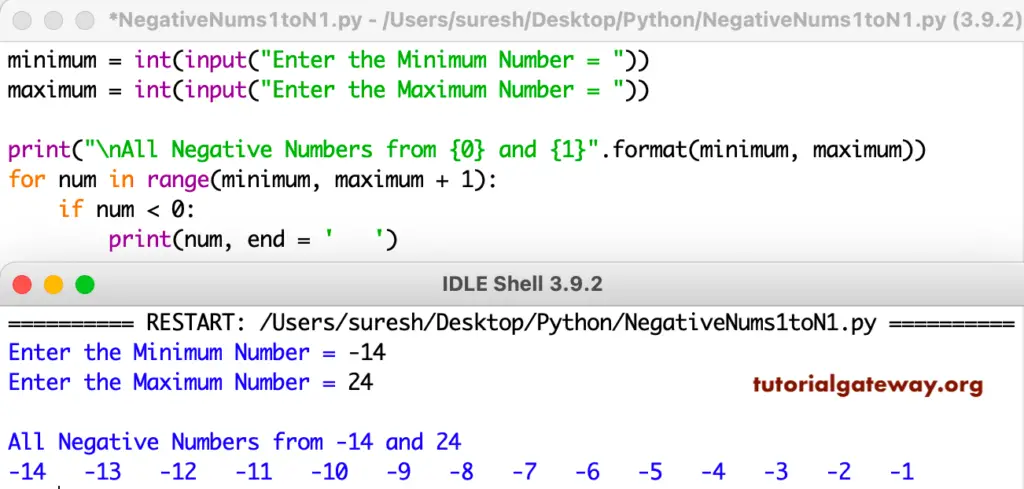
This program helps to print negative numbers in a range or from 1 to n using a while loop.
minimum = int(input("Enter the Minimum Number = ")) maximum = int(input("Enter the Maximum Number = ")) print("\nFrom {0} and {1}".format(minimum, maximum)) while minimum <= maximum: if minimum < 0: print(minimum, end = ' ') minimum = minimum + 1
Enter the Minimum Number = -35 Enter the Maximum Number = 400 From -35 and 400 -35 -34 -33 -32 -31 -30 -29 -28 -27 -26 -25 -24 -23 -22 -21 -20 -19 -18 -17 -16 -15 -14 -13 -12 -11 -10 -9 -8 -7 -6 -5 -4 -3 -2 -1
In this example, the negativeNumbers function accepts two integers and prints the negative numbers between them.
# using functions def negativeNumbers(x, y): for num in range(x, y + 1): if num < 0: print(num, end = ' ') minimum = int(input("Enter the Minimum = ")) maximum = int(input("Enter the Maximum = ")) print("\nFrom {0} and {1}".format(minimum, maximum)) negativeNumbers(minimum, maximum)
Enter the Minimum = -25
Enter the Maximum = 120
From -25 and 120
-25 -24 -23 -22 -21 -20 -19 -18 -17 -16 -15 -14 -13 -12 -11 -10 -9 -8 -7 -6 -5 -4 -3 -2 -1