Write a Python Program to Print Multiplication Table using For Loop and While Loop with an example. If you want the multiplication table for a single number, the loop will return the result. However, you have to use nested loops for multiple numbers. The first one is for the original number and the nested loop to iterate from 1 to 10.
Python Program to Print Multiplication Table using For loop
This program displays the multiplication table from 8 to 10 using For Loop. The first for loop iterates from 8 to 10 to print the table of 8 and 9. The nested for loop iterates from 1 to 10 and generates the table.
for i in range(8, 10): for j in range(1, 11): print('{0} * {1} = {2}'.format(i, j, i*j)) print('==============')
Multiplication Table output.
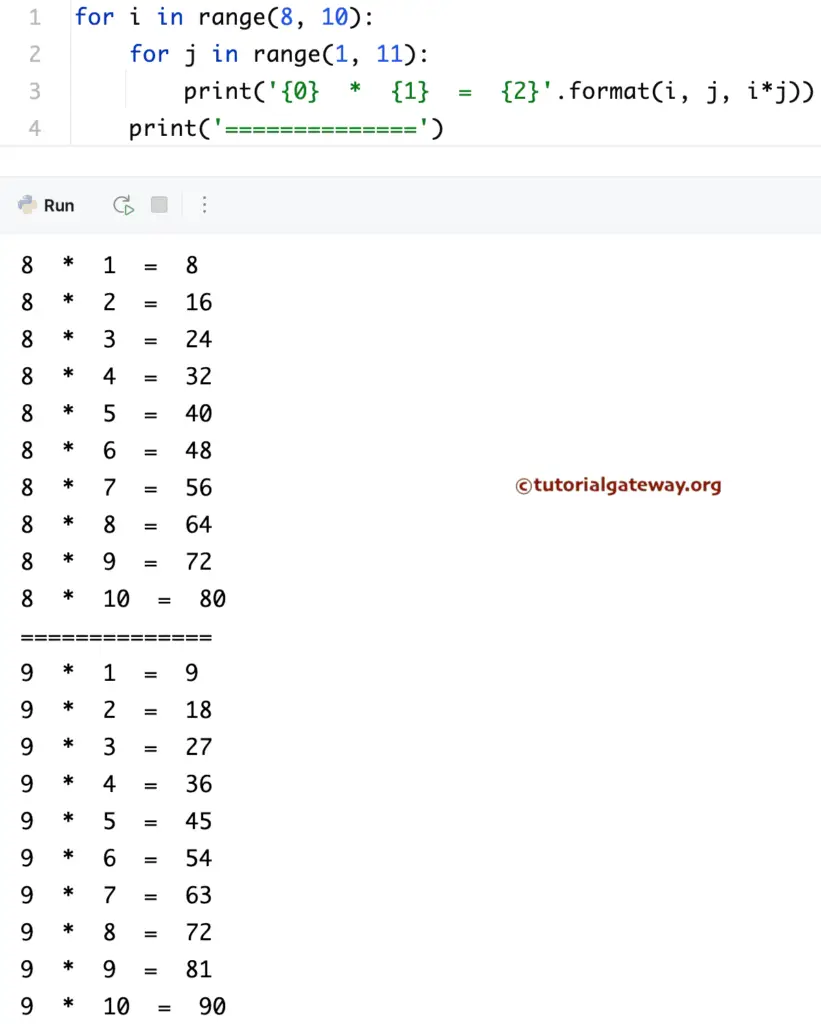
Multiplication Table using for loop Example 2
This Python program allows users to enter any integer value. Next, the print function in the For Loop prints the multiplication table from a user-entered value to 10.
# using Nested for loop num = int(input(" Please Enter any Positive Integer lessthan 10 : ")) print(" Multiplication Table ") for i in range(num, 10): for j in range(1, 11): print('{0} * {1} = {2}'.format(i, j, i*j)) print('==============')
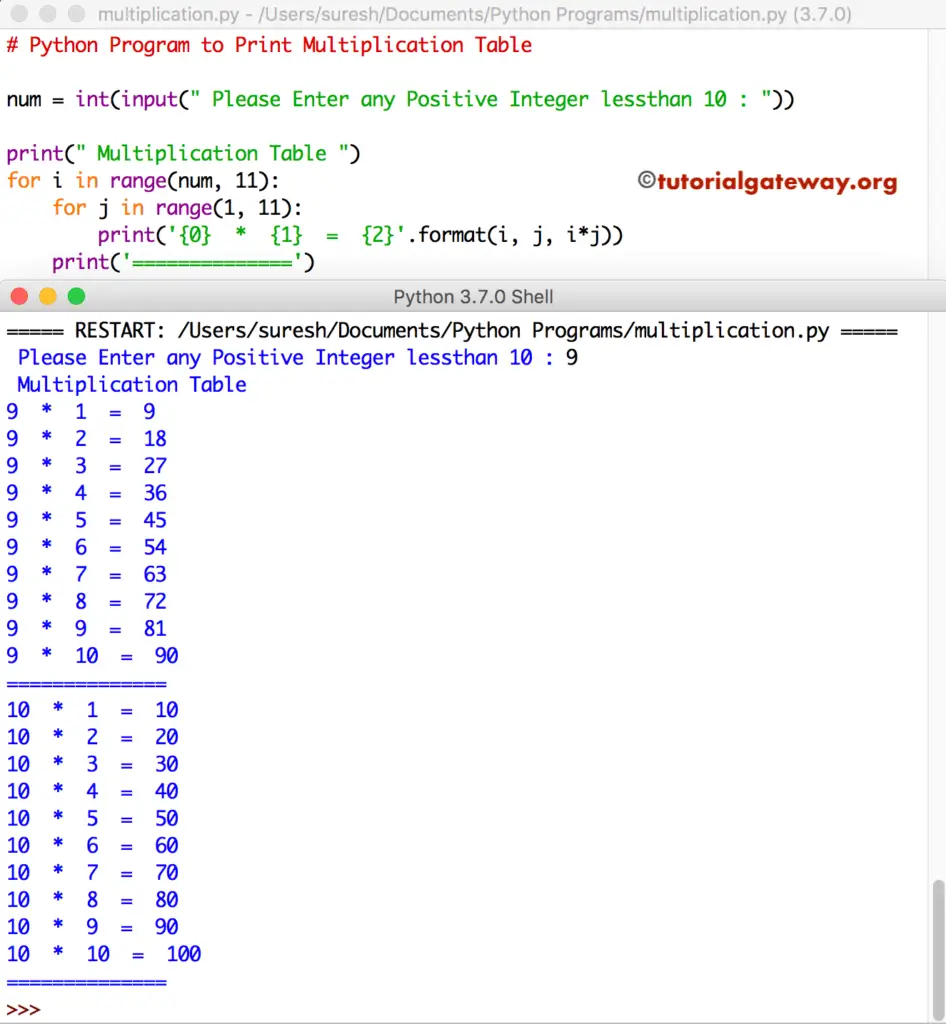
How to Print Multiplication Table using While loop?
This multiplication table program is the same as above. But this time, we are using While Loop, and don’t forget to increment the i (i = i + 1) and j (j = j + 1)variables.
# using while loop i = int(input(" Please Enter any Positive Integer less than 10 : ")) while(i <= 10): j = 1 while(j <= 10): print('{0} * {1} = {2}'.format(i, j, i*j)) j = j + 1 print('==============') i = i + 1
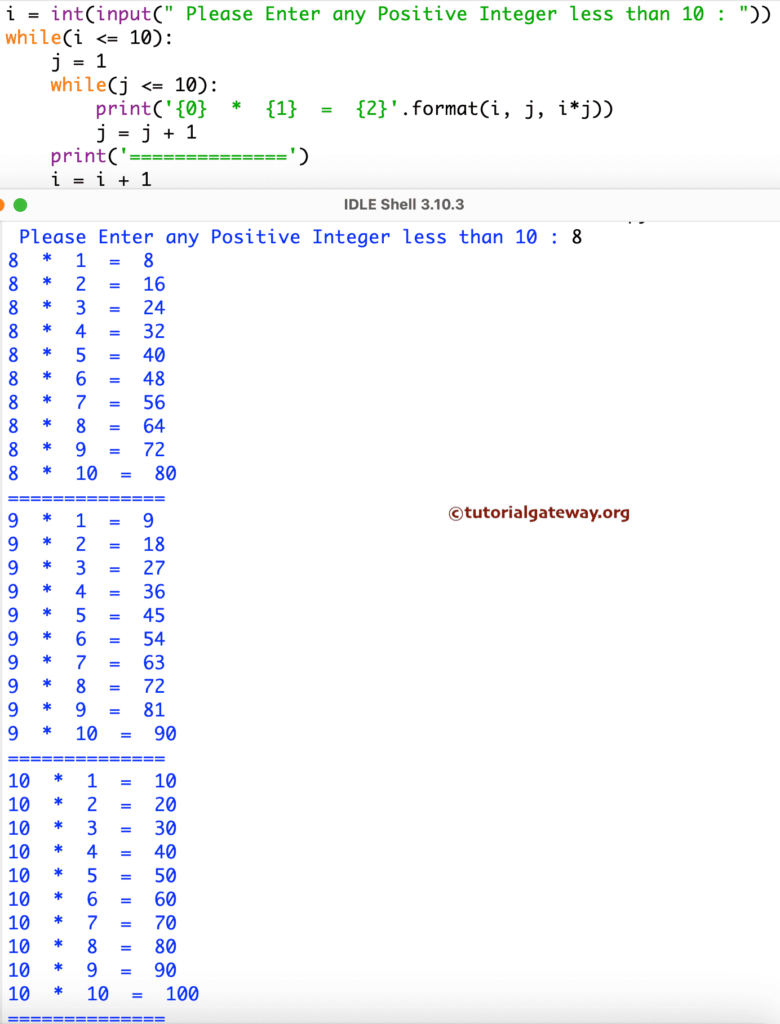