Write a Python program to print hollow sandglass star pattern using for loop.
rows = int(input("Enter Hollow Sandglass Star Pattern Rows = ")) print("====The Hollow Sandglass Star Pattern====") for i in range(1, rows + 1): for j in range(1, i): print(end = ' ') for k in range(i, rows + 1): if i == 1 or k == i or k == rows: print('*', end = ' ') else: print(end = ' ') print() for i in range(rows - 1, 0, -1): for j in range(1, i): print(end = ' ') for k in range(i, rows + 1): if i == 1 or k == i or k == rows: print('*', end = ' ') else: print(end = ' ') print()
Enter Hollow Sandglass Star Pattern Rows = 8
====The Hollow Sandglass Star Pattern====
* * * * * * * *
* *
* *
* *
* *
* *
* *
*
* *
* *
* *
* *
* *
* *
* * * * * * * *
In this Python example, we created a function that will run the repeated for loops to print the hollow sandglass star pattern.
def hollowsandcheck(i, rows): for j in range(1, i): print(end = ' ') for k in range(i, rows + 1): if i == 1 or k == i or k == rows: print('*', end = ' ') else: print(end = ' ') rows = int(input("Enter Hollow Sandglass Star Pattern Rows = ")) print("====The Hollow Sandglass Star Pattern====") for i in range(1, rows + 1): hollowsandcheck(i, rows) print() for i in range(rows - 1, 0, -1): hollowsandcheck(i, rows) print()
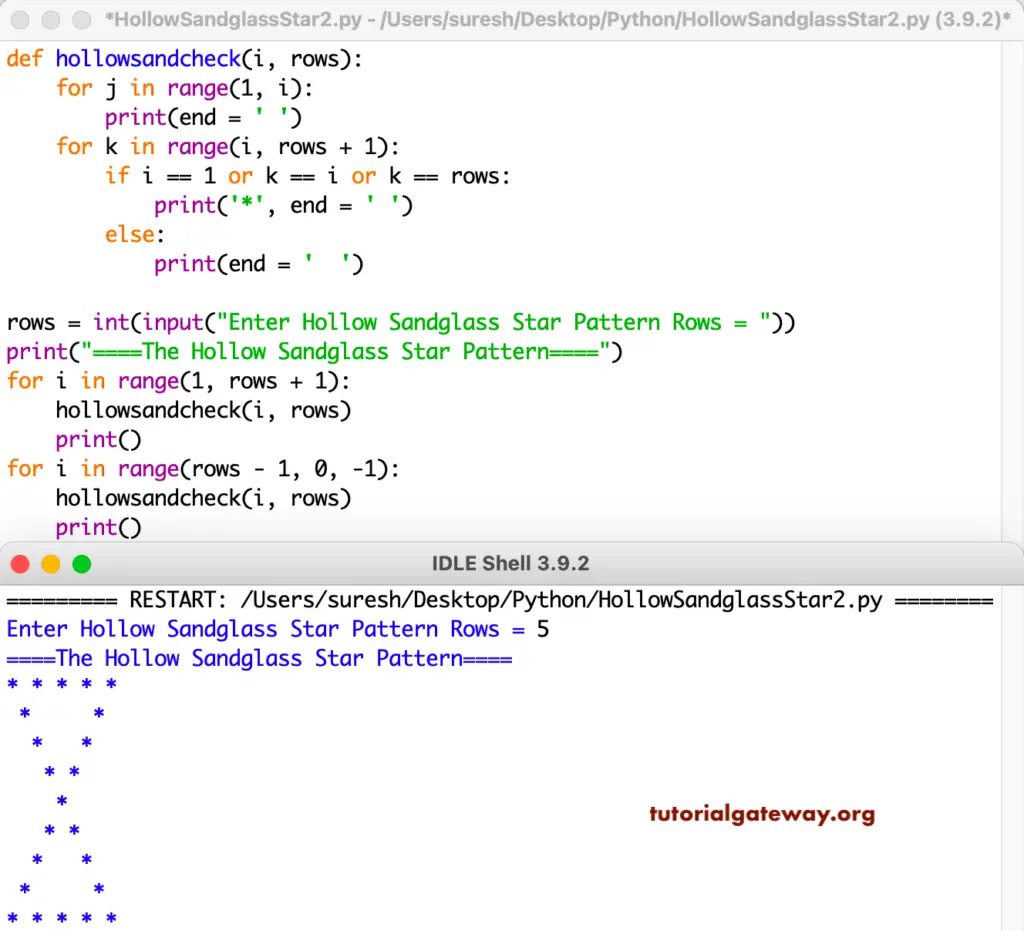
This Python program displays the hollow sandglass pattern of stars using a while loop.
def hollowsandcheck(i, rows): j = 1 while(j < i): print(end = ' ') j = j + 1 k = i while(k <= rows): if i == 1 or k == i or k == rows: print('*', end = ' ') else: print(end = ' ') k = k + 1 rows = int(input("Enter Hollow Sandglass Star Pattern Rows = ")) print("====The Hollow Sandglass Star Pattern====") i = 1 while(i <= rows): hollowsandcheck(i, rows) print() i = i + 1 i = rows - 1 while(i >= 1): hollowsandcheck(i, rows) print() i = i - 1
Enter Hollow Sandglass Star Pattern Rows = 10
====The Hollow Sandglass Star Pattern====
* * * * * * * * * *
* *
* *
* *
* *
* *
* *
* *
* *
*
* *
* *
* *
* *
* *
* *
* *
* *
* * * * * * * * * *
This Python example allows the user to enter the character and prints the hollow sandglass pattern of the given character.
def hollowsandcheck(i, rows, ch): for j in range(1, i): print(end = ' ') for k in range(i, rows + 1): if i == 1 or k == i or k == rows: print('%c' %ch, end = ' ') else: print(end = ' ') rows = int(input("Enter Hollow Sandglass Star Pattern Rows = ")) ch = input("Symbol to use in Hollow Sandglass Pattern = " ) print("====The Hollow Sandglass Star Pattern====") for i in range(1, rows + 1): hollowsandcheck(i, rows, ch) print() for i in range(rows - 1, 0, -1): hollowsandcheck(i, rows, ch) print()
Enter Hollow Sandglass Star Pattern Rows = 13
Symbol to use in Hollow Sandglass Pattern = @
====The Hollow Sandglass Star Pattern====
@ @ @ @ @ @ @ @ @ @ @ @ @
@ @
@ @
@ @
@ @
@ @
@ @
@ @
@ @
@ @
@ @
@ @
@
@ @
@ @
@ @
@ @
@ @
@ @
@ @
@ @
@ @
@ @
@ @
@ @ @ @ @ @ @ @ @ @ @ @ @