Write a Python Program to Print Box Number Pattern of 1 and 0 using For Loop and While Loop with an example.
Python Program to Print Box Number Pattern of 1 and 0 using For Loop
This Python program allows user to enter the total number of rows and columns. Next, we used Python Nested For Loop to iterate each row and column items. Within the loop, we used the If statement to check whether the row and column numbers are 1 or maximum. If True, Python print 1 otherwise 0.
# Python Program to Print Box Number Pattern of 1 and 0 rows = int(input("Please Enter the total Number of Rows : ")) columns = int(input("Please Enter the total Number of Columns : ")) print("Box Number Pattern of 1 and 0") for i in range(1, rows + 1): for j in range(1, columns + 1): if(i == 1 or i == rows or j == 1 or j == columns): print('1', end = ' ') else: print('0', end = ' ') print()
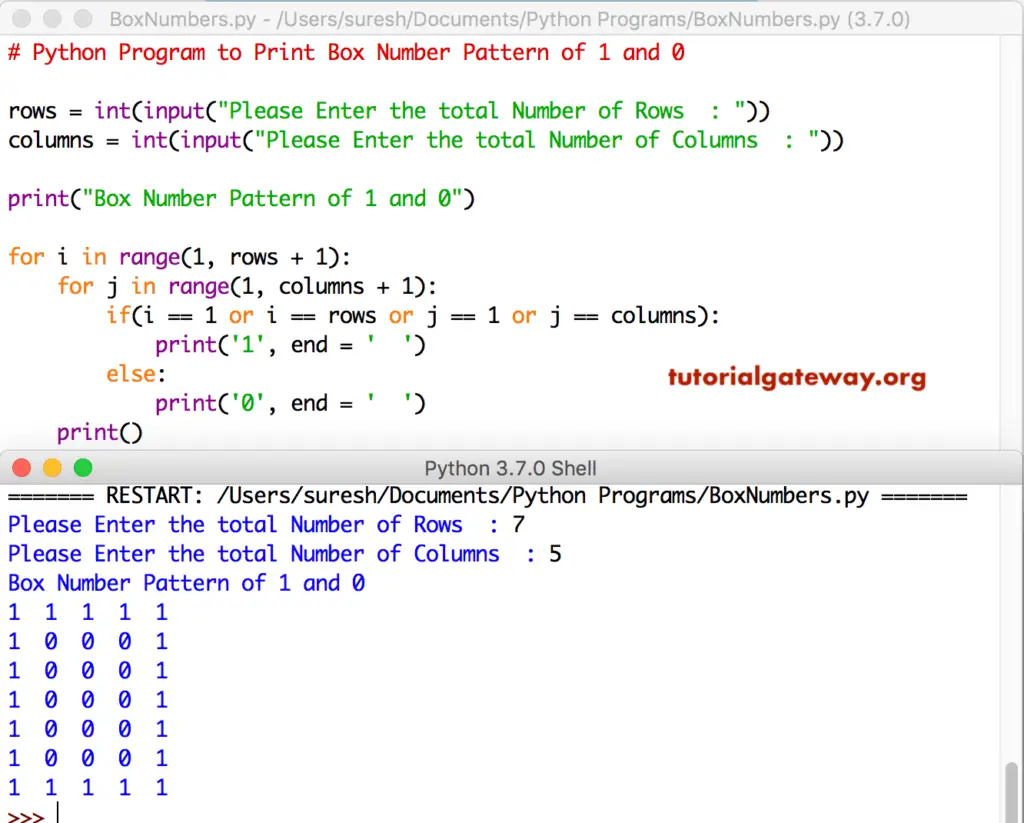
Python Program to Display Box Number Pattern of 1 and 0 using While Loop
This Python program is the same as above. However, we replaced the For Loop with While Loop
# Python Program to Print Box Number Pattern of 1 and 0 rows = int(input("Please Enter the total Number of Rows : ")) columns = int(input("Please Enter the total Number of Columns : ")) print("Box Number Pattern of 1 and 0") i = 1 while(i <= rows): j = 1; while(j <= columns ): if(i == 1 or i == rows or j == 1 or j == columns): print('1', end = ' ') else: print('0', end = ' ') j = j + 1 i = i + 1 print()
Please Enter the total Number of Rows : 8
Please Enter the total Number of Columns : 14
Box Number Pattern of 1 and 0
1 1 1 1 1 1 1 1 1 1 1 1 1 1
1 0 0 0 0 0 0 0 0 0 0 0 0 1
1 0 0 0 0 0 0 0 0 0 0 0 0 1
1 0 0 0 0 0 0 0 0 0 0 0 0 1
1 0 0 0 0 0 0 0 0 0 0 0 0 1
1 0 0 0 0 0 0 0 0 0 0 0 0 1
1 0 0 0 0 0 0 0 0 0 0 0 0 1
1 1 1 1 1 1 1 1 1 1 1 1 1 1
>>>
Python Program for Box Number Pattern of 0 and 1
If you want to Python to display box pattern of numbers 0 and 1, replace 1 in print statement with 0, and 0 with 1.
# Python Program to Print Box Number Pattern of 1 and 0 rows = int(input("Please Enter the total Number of Rows : ")) columns = int(input("Please Enter the total Number of Columns : ")) print("Box Number Pattern of 1 and 0") for i in range(1, rows + 1): for j in range(1, columns + 1): if(i == 1 or i == rows or j == 1 or j == columns): print('0', end = ' ') else: print('1', end = ' ') print()
Please Enter the total Number of Rows : 9
Please Enter the total Number of Columns : 15
Box Number Pattern of 1 and 0
0 0 0 0 0 0 0 0 0 0 0 0 0 0 0
0 1 1 1 1 1 1 1 1 1 1 1 1 1 0
0 1 1 1 1 1 1 1 1 1 1 1 1 1 0
0 1 1 1 1 1 1 1 1 1 1 1 1 1 0
0 1 1 1 1 1 1 1 1 1 1 1 1 1 0
0 1 1 1 1 1 1 1 1 1 1 1 1 1 0
0 1 1 1 1 1 1 1 1 1 1 1 1 1 0
0 1 1 1 1 1 1 1 1 1 1 1 1 1 0
0 0 0 0 0 0 0 0 0 0 0 0 0 0 0
>>>