Write a Python program to print array elements present on odd position or odd index position. In this example, the list slicing starts at 0 and increments by 2.
import numpy as np arr = np.array([1, 3, 5, 7, 9, 11, 13, 15, 17, 19, 21]) print(arr[0:len(arr):2])
[ 1 5 9 13 17 21]
Python program to print array elements present on odd index position using a for loop.
import numpy as np arr = np.array([10, 25, 20, 33, 40, 55, 60, 77]) print('Printing the Array Elements at Even Position') for i in range(0, len(arr), 2): print(arr[i], end = ' ')
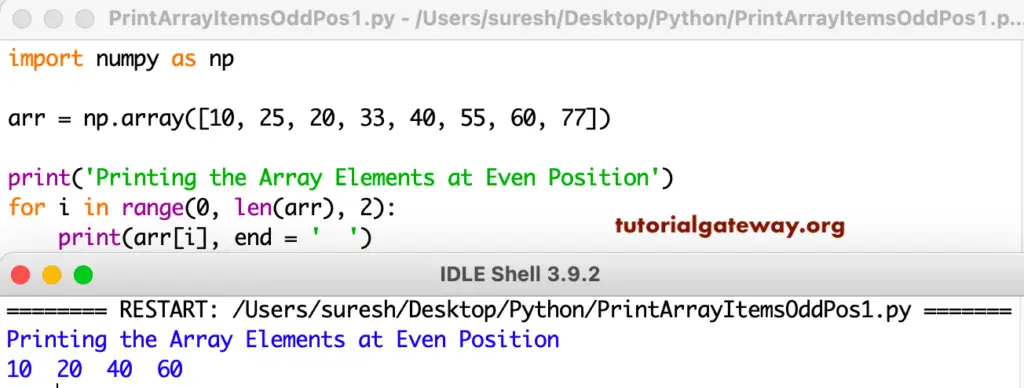
This Python program helps to print array elements present in odd positions using a while loop.
import numpy as np odarr = np.array([3, 9, 11, 4, 22, 8, 99, 19, 7]) i = 0 while i < len(odarr): print(odarr[i], end = ' ') i = i + 2
3 11 22 99 7
In this example, the if statement (if i % 2 == 0) finds the odd index position and prints the array of elements present on the odd position.
import numpy as np odarrlist = [] odarrTot = int(input("Total Array Elements to enter = ")) for i in range(1, odarrTot + 1): odarrvalue = int(input("Please enter the %d Array Value = " %i)) odarrlist.append(odarrvalue) odarr = np.array(odarrlist) print('Printing the Array Elements at Odd Position') for i in range(0, len(odarr), 2): print(odarr[i], end = ' ') print('\nPrinting the Array Elements at Odd Position') for i in range(len(odarr)): if i % 2 == 0: print(odarr[i], end = ' ')
Total Array Elements to enter = 9
Please enter the 1 Array Value = 17
Please enter the 2 Array Value = 22
Please enter the 3 Array Value = 33
Please enter the 4 Array Value = 45
Please enter the 5 Array Value = 56
Please enter the 6 Array Value = 76
Please enter the 7 Array Value = 78
Please enter the 8 Array Value = 89
Please enter the 9 Array Value = 90
Printing the Array Elements at Odd Position
17 33 56 78 90
Printing the Array Elements at Odd Position
17 33 56 78 90