Write a Python program to left rotate a Numpy Array by n times or positions. In order to left rotate the array, we sliced the array based on the position and combined it with the numpy concatenate function in this example.
import numpy as np ltArray = np.array([4, 7, 9, 11, 22, 55, 99, 125, 78, 345]) ltRotate = int(input("Enter Position to Left Rotate Array Elements = ")) print('Original Array Elements Before Left Rotating') print(ltArray) arr = np.concatenate((ltArray[ltRotate:], ltArray[:ltRotate]), axis = 0) print('\nFinal Array Elements After Left Rotating') print(arr)
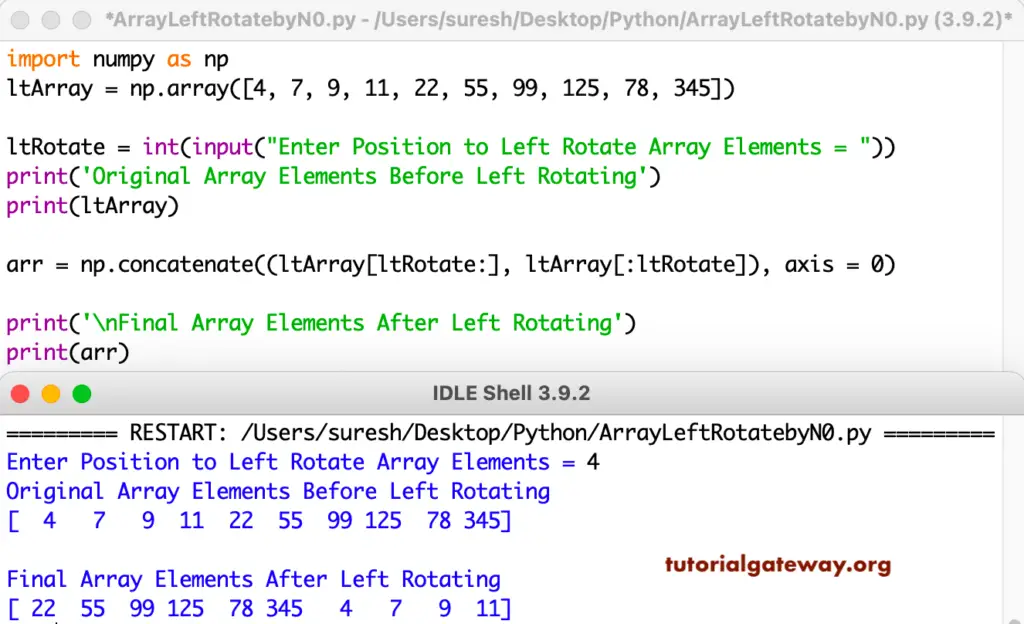
This Python program uses the for loop to left rotate the Numpy array items by the user given position.
import numpy as np ltArray = np.array([19, 25, 32, 77, 28, 99, 44, 88]) ltRotate = int(input("Enter Position to Left Rotate Array Elements = ")) print('Original Array Elements Before Left Rotating') print(ltArray) for i in range(ltRotate): firstValue = ltArray[0] for j in range(len(ltArray) - 1): ltArray[j] = ltArray[j + 1] ltArray[len(ltArray) - 1] = firstValue print('\nFinal Array Elements After Left Rotating') print(ltArray)
Enter Position to Left Rotate Array Elements = 5
Original Array Elements Before Left Rotating
[19 25 32 77 28 99 44 88]
Final Array Elements After Left Rotating
[99 44 88 19 25 32 77 28]
In this example, the leftRotateArray function will rotate the NumPy array items to the left hand side, and printArrayItems prints the array items.
import numpy as np def printArrayItems(ltArray): for i in ltArray: print(i, end = ' ') def leftRotateArray(ltArray, ltRotate): for i in range(ltRotate): firstValue = ltArray[0] for j in range(len(ltArray) - 1): ltArray[j] = ltArray[j + 1] ltArray[len(ltArray) - 1] = firstValue ltArray = np.random.randint(11, 110, size = 10) ltRotate = int(input("\nEnter Position to Left Rotate Array Elements = ")) print('Original Array Elements Before Left Rotating') printArrayItems(ltArray) leftRotateArray(ltArray, ltRotate) print('\nFinal Array Elements After Left Rotating') printArrayItems(ltArray)
Enter Position to Left Rotate Array Elements = 7
Original Array Elements Before Left Rotating
73 45 65 97 84 99 40 18 77 105
Final Array Elements After Left Rotating
18 77 105 73 45 65 97 84 99 40
This program helps to left rotate a Numpy Array by n using a while loop.
import numpy as np def printArrayItems(ltArray): i = 0 while i < len(ltArray): print(ltArray[i], end = ' ') i = i + 1 def leftRotateArray(ltArray, ltRotate): i = 0 while(i < ltRotate): firstValue = ltArray[0] j = 0 while(j < len(ltArray) - 1): ltArray[j] = ltArray[j + 1] j = j + 1 ltArray[len(ltArray) - 1] = firstValue i = i + 1 ltArray = np.random.randint(70, 500, size = 15) ltRotate = int(input("\nEnter Position to Left Rotate Array Elements = ")) print('Original Array Elements Before Left Rotating') printArrayItems(ltArray) leftRotateArray(ltArray, ltRotate) print('\nFinal Array Elements After Left Rotating') printArrayItems(ltArray)
Enter Position to Left Rotate Array Elements = 6
Original Array Elements Before Left Rotating
204 76 370 211 180 453 407 250 213 332 456 333 292 460 354
Final Array Elements After Left Rotating
407 250 213 332 456 333 292 460 354 204 76 370 211 180 453