Write a Python Program to Find the second Largest Number in a Numpy Array. We used the numpy sort function to sort the array in ascending order. Next, we print the value at the last but one index position.
import numpy as np secLarr = np.array([11, 55, 99, 22, 7, 35, 70]) print("Array Items = ", secLarr) secLarr.sort() print("The Second Largest Item in this Array = ", secLarr[len(secLarr) - 2])
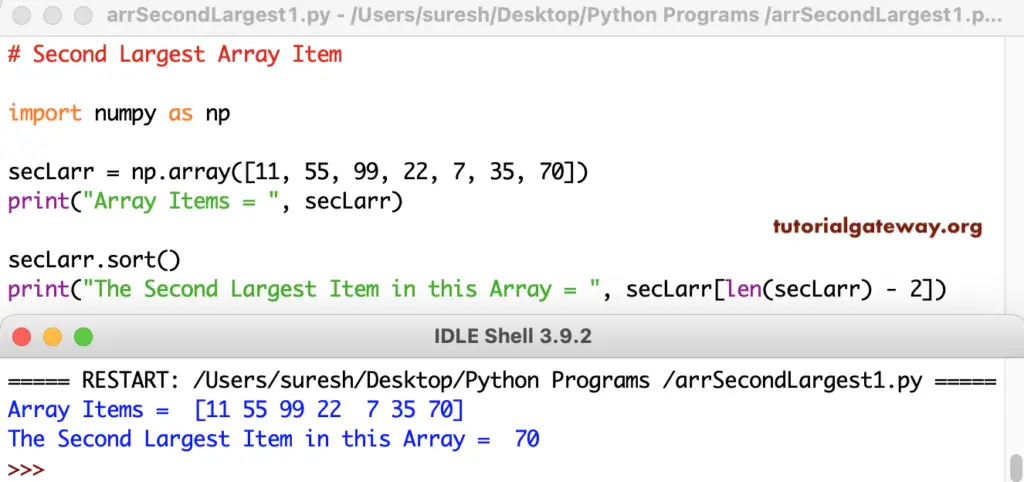
This Python Program helps to Find the Second Largest in the numpy Array using the For Loop range.
import numpy as np secLarr = np.array([15, 22, 75, 99, 35, 70, 120, 60]) print("Items = ", secLarr) first = second = min(secLarr) for i in range(len(secLarr)): if (secLarr[i] > first): second = first first = secLarr[i] elif(secLarr[i] > second and secLarr[i] < first): second = secLarr[i] print("The Largest Item = ", first) print("The Second Largest Item = ", second)
The Second Largest numpy array item using the for loop range output.
Items = [ 15 22 75 99 35 70 120 60]
The Largest Item = 120
The Second Largest Item = 99