Write a Python Program to find the Last Digit in a Number with a practical example.
Python Program to find the Last Digit in a Number
This python program allows the user to enter any integer value. Next, Python is going to find the Last Digit of the user-entered value.
# Python Program to find the Last Digit in a Number number = int(input("Please Enter any Number: ")) last_digit = number % 10 print("The Last Digit in a Given Number %d = %d" %(number, last_digit))
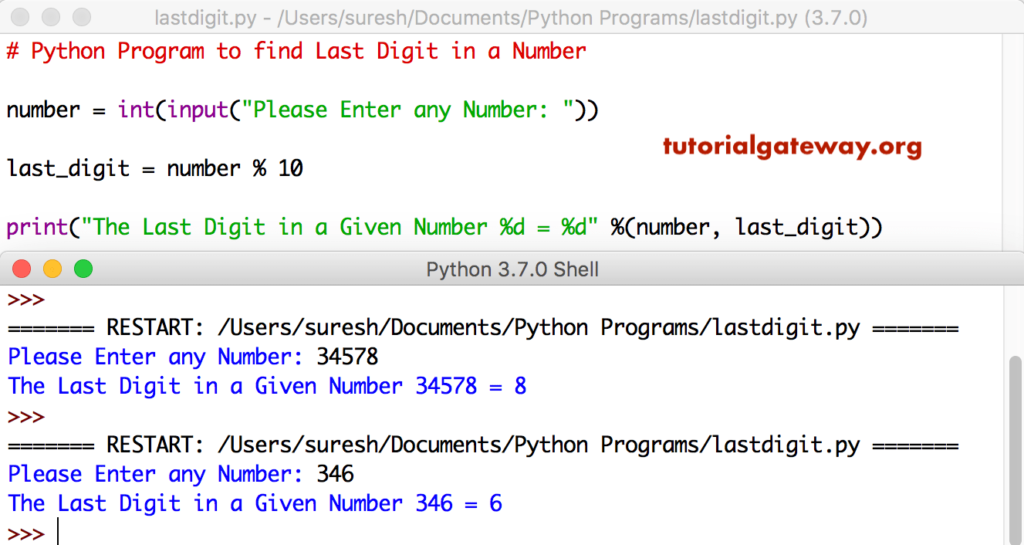
Python Program to find the Last Digit in a Number using Function
This Python last digit in a number program is the same as above. But this time, we separated the Python logic and placed it in a separate function.
# Python Program to find the Last Digit in a Number def lastDigit(num): return num % 10 number = int(input("Please Enter any Number: ")) last_digit = lastDigit(number) print("The Last Digit in a Given Number %d = %d" %(number, last_digit))
Python last digit output
Please Enter any Number: 1925
The Last Digit in a Given Number 1925 = 5
>>>
Please Enter any Number: 90876
The Last Digit in a Given Number 90876 = 6