Write a Python program to find the distance between two points. This example accepts the first and second coordinate points and calculates the distance using math pow and sqrt functions.
import math x1 = int(input("Enter the First Point Coordinate x1 = ")) y1 = int(input("Enter the First Point Coordinate y1 = ")) x2 = int(input("Enter the Second Point Coordinate x2 = ")) y2 = int(input("Enter the Second Point Coordinate y2 = ")) x = math.pow((x2 - x1), 2) y = math.pow((y2 - y1), 2) print(x) print(y) print(math.sqrt(x + y)) distance = math.sqrt(x + y) print('The Distance Between Two Points = {0} Units'.format(distance))
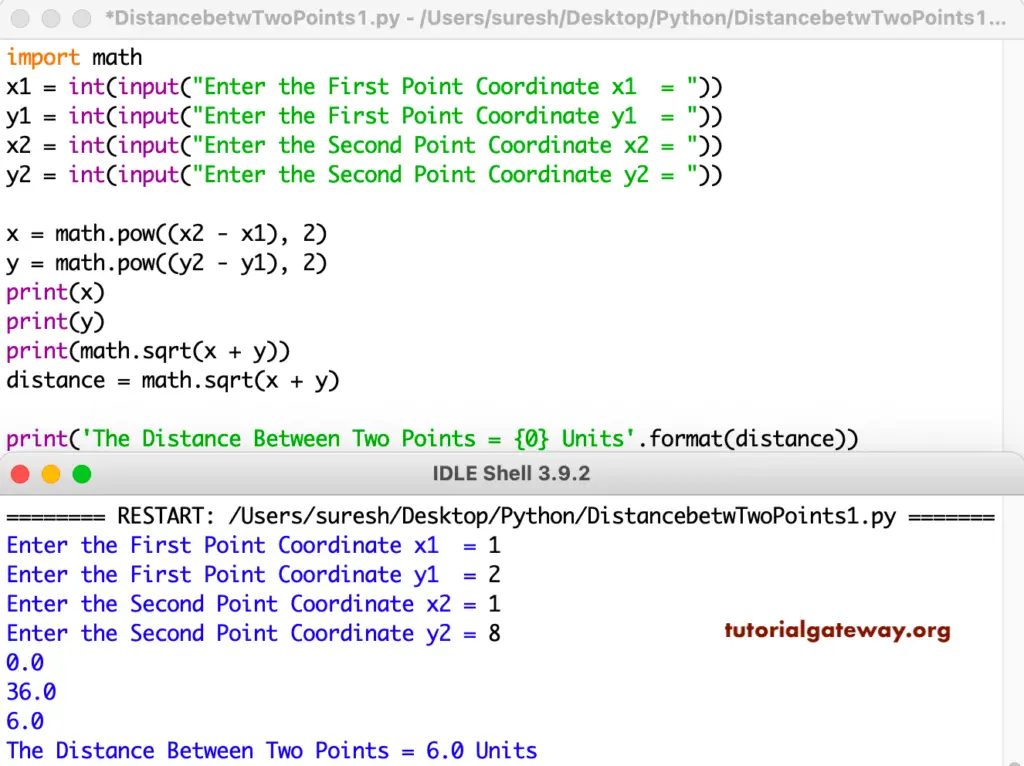
Python program to find the distance between two points using functions.
This example accepts two points and returns the distance between those two.
import math def distanceBetweenTwo(x1, y1, x2, y2): return math.sqrt((math.pow((x2 - x1), 2)) + (math.pow((y2 - y1), 2))) x1 = int(input("Enter the First Point Coordinate x1 = ")) y1 = int(input("Enter the First Point Coordinate y1 = ")) x2 = int(input("Enter the Second Point Coordinate x2 = ")) y2 = int(input("Enter the Second Point Coordinate y2 = ")) distance = distanceBetweenTwo(x1, y1, x2, y2) print('The Distance Between Two Points = {0} Units'.format(distance))
Enter the First Point Coordinate x1 = 1
Enter the First Point Coordinate y1 = 11
Enter the Second Point Coordinate x2 = 3
Enter the Second Point Coordinate y2 = 25
The Distance Between Two Points = 14.142135623730951 Units