Write Python Program to find Diameter Circumference and Area Of a Circle with a practical example. The mathematical formulas are:
- Diameter of a Circle = 2r = 2 * radius
- Area of a circle is: A = πr² = π * radius * radius
- Circumference of a Circle = 2πr = 2 * π * radius
Python Program to find Diameter Circumference and Area Of a Circle example 1
This Python program allows users to enter the radius of a circle. By using the radius value, this Python code finds the Circumference, Diameter, and Area Of a Circle.
TIP: Refer Find Area Of a Circle article to understand the various ways to find the Python Area of a Circle.
# Python Program to find Diameter, Circumference, and Area Of a Circle PI = 3.14 radius = float(input(' Please Enter the radius of a circle: ')) diameter = 2 * radius circumference = 2 * PI * radius area = PI * radius * radius print(" \nDiameter Of a Circle = %.2f" %diameter) print(" Circumference Of a Circle = %.2f" %circumference) print(" Area Of a Circle = %.2f" %area)
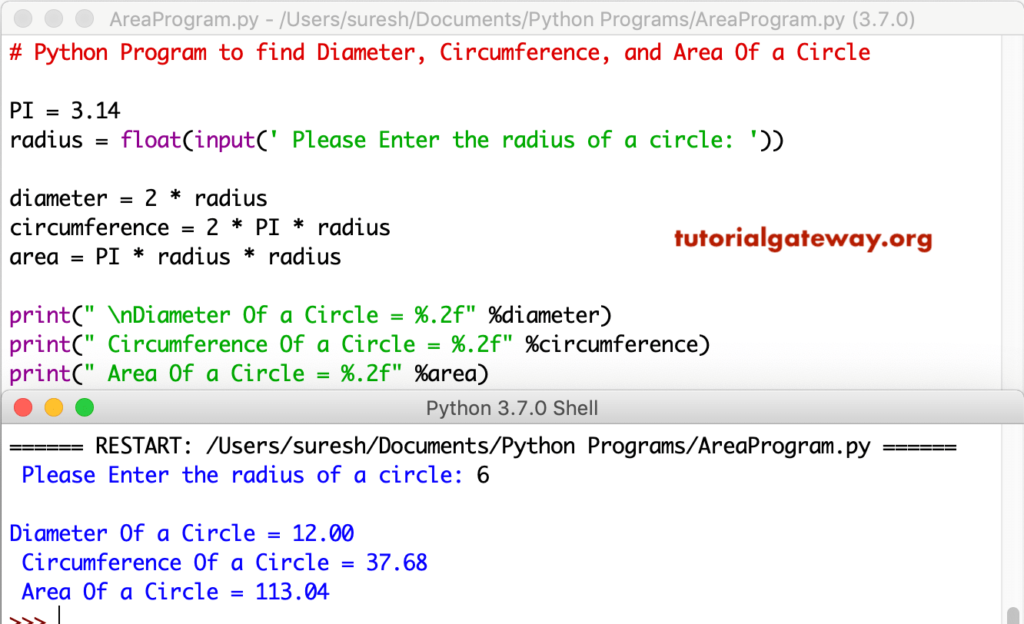
Python Program to Calculate Diameter Circumference and Area Of a Circle example 2
This python code to find Diameter, Circumference, and Area of Circle is the same as above. However, we separated the logic using Python functions.
# Python Program to find Diameter, Circumference, and Area Of a Circle import math def find_Diameter(radius): return 2 * radius def find_Circumference(radius): return 2 * math.pi * radius def find_Area(radius): return math.pi * radius * radius r = float(input(' Please Enter the radius of a circle: ')) diameter = find_Diameter(r) circumference = find_Circumference(r) area = find_Area(r) print("\n Diameter Of a Circle = %.2f" %diameter) print(" Circumference Of a Circle = %.2f" %circumference) print(" Area Of a Circle = %.2f" %area)
Python Diameter and Circumference of a Circle output
Please Enter the radius of a circle: 12
Diameter Of a Circle = 24.00
Circumference Of a Circle = 75.40
Area Of a Circle = 452.39