Write a Python Program to find Angle of a Triangle if two angles are given with a practical example.
Python Program to find Angle of a Triangle if two angles are given Example 1
This python program helps the user to enter two angles of a triangle. Next, we subtracted those two angles from 180 because the sum of all angles in a triangle = 180.
# Python Program to find Angle of a Triangle if two angles are given a = float(input('Please Enter the First Angle of a Triangle: ')) b = float(input('Please Enter the Second Angle of a Triangle: ')) # Finding the Third Angle c = 180 - (a + b) print("Third Angle of a Triangle = ", c)
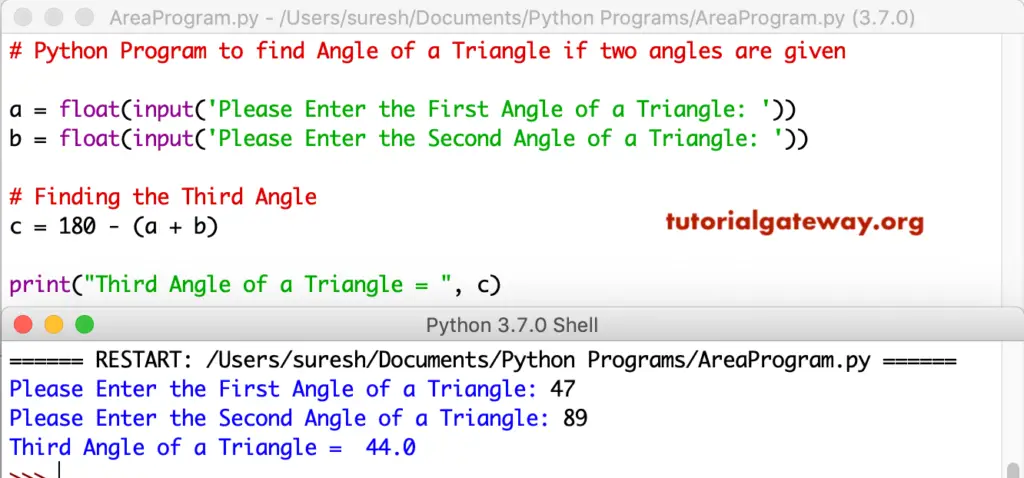
Python Program to find Angle of Triangle using two angles Example 2
This Python code to find Angle of a Triangle is the same as above. However, we separated the angle of a triangle program logic using the concept of functions.
# Python Program to find Angle of a Triangle if two angles are given def triangle_angle(a, b): return 180 - (a + b) a = float(input('Please Enter the First Angle of a Triangle: ')) b = float(input('Please Enter the Second Angle of a Triangle: ')) # Finding the Third Angle c = triangle_angle(a, b) print("Third Angle of a Triangle = ", c)
Python Angle of a Triangle output
Please Enter the First Angle of a Triangle: 45
Please Enter the Second Angle of a Triangle: 95
Third Angle of a Triangle = 40.0