Write a Python Program to Count Positive and Negative Numbers in Set. The for loop (for eoVal in pongtSet) iterates all the set items. And the if condition (if(eoVal >= 0)) checks whether the Set item is greater than or equals to zero. If True, we add one to the Positive Set count; otherwise, add one to the Negative Set count value.
# Count of Set Positive and Negative Numbers pongtSet = {6, -33, 99, -28, -56, 11, -45, -43} print("Positive and Negative Set Items = ", pongtSet) sPositiveCount = sNegativeCount = 0 for eoVal in pongtSet: if(eoVal >= 0): sPositiveCount = sPositiveCount + 1 else: sNegativeCount = sNegativeCount + 1 print("The Count of Positive Numbers in pongtSet = ", sPositiveCount) print("The Count of Negative Numbers in pongtSet = ", sNegativeCount)
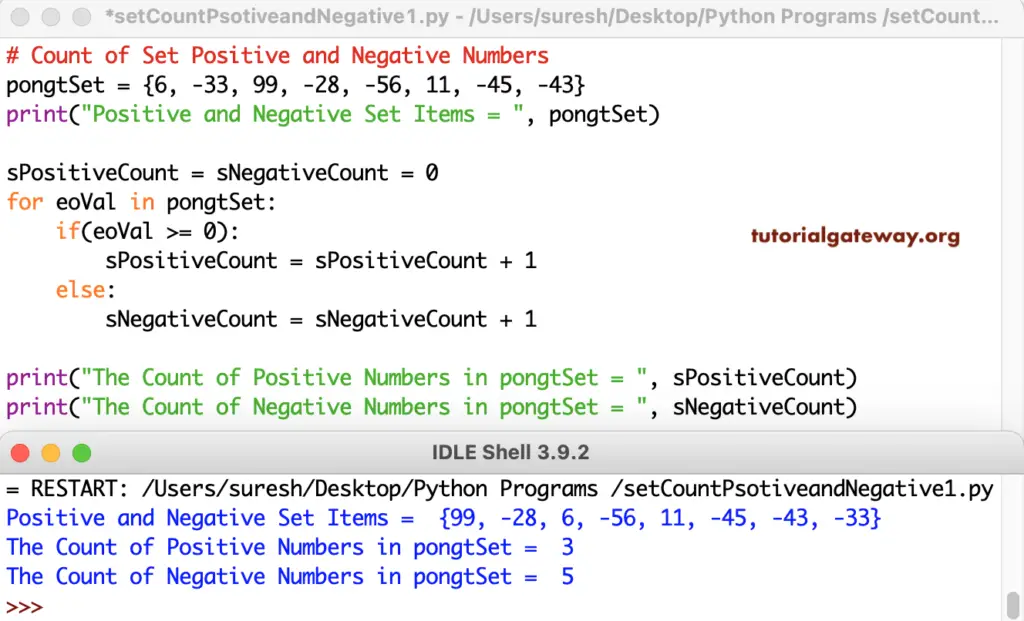
Python Program to Count Positive and Negative Numbers in Set
This Python Positive and Negative numbers example allows entering the set items using the for loop range.
# Count of Set Positive and Negative Numbers pongtSet = set() number = int(input("Enter the Total Positive Negative Set Items = ")) for i in range(1, number + 1): value = int(input("Enter the %d Set Item = " %i)) pongtSet.add(value) print("Positive and Negative Set Items = ", pongtSet) sPositiveCount = sNegativeCount = 0 for eoVal in pongtSet: if(eoVal >= 0): sPositiveCount = sPositiveCount + 1 else: sNegativeCount = sNegativeCount + 1 print("The Count of Positive Numbers in pongtSet = ", sPositiveCount) print("The Count of Negative Numbers in pongtSet = ", sNegativeCount)
Python Count Positive and Negative set numbers output
Enter the Total Positive Negative Set Items = 4
Enter the 1 Set Item = -22
Enter the 2 Set Item = -99
Enter the 3 Set Item = 8
Enter the 4 Set Item = -67
Positive and Negative Set Items = {8, -22, -99, -67}
The Count of Positive Numbers in pongtSet = 1
The Count of Negative Numbers in pongtSet = 3
In this Python Set example, we created a CountOfSetPositiveandNegativeNumbers function that returns the Positive and Negative numbers count.
# Count of Set Positive and Negative Numbers def CountOfSetPositiveandNegativeNumbers(pongtSet): sPositiveCount = sNegativeCount = 0 for eoVal in pongtSet: if(eoVal >= 0): sPositiveCount = sPositiveCount + 1 else: sNegativeCount = sNegativeCount + 1 return sPositiveCount, sNegativeCount pongtSet = set() number = int(input("Enter the Total Positive Negative Set Items = ")) for i in range(1, number + 1): value = int(input("Enter the %d Set Item = " %i)) pongtSet.add(value) print("Positive and Negative Set Items = ", pongtSet) sECount, sOCount = CountOfSetPositiveandNegativeNumbers(pongtSet) print("The Count of Positive Numbers in pongtSet = ", sECount) print("The Count of Negative Numbers in pongtSet = ", sOCount)
Enter the Total Positive Negative Set Items = 6
Enter the 1 Set Item = 22
Enter the 2 Set Item = -90
Enter the 3 Set Item = -78
Enter the 4 Set Item = 9
Enter the 5 Set Item = 32
Enter the 6 Set Item = 8
Positive and Negative Set Items = {32, -90, 8, 9, -78, 22}
The Count of Positive Numbers in pongtSet = 4
The Count of Negative Numbers in pongtSet = 2