Write a Python Program to Count Even and Odd Numbers in Set. The for loop (for eoVal in evodSet) iterates all the set items. And the if condition (if(eoVal % 2 == 0)) checks whether the Set item divisible by two equals zero. If True, we add one to the even Set count; otherwise (sOddCount = sOddCount + 1), add one to the odd Set count value.
# Count of Set Even and Odd Numbers evodSet = {78, 11, 54, 95, 16, 36, 61, 77, 150, 122} print("Even and Odd Set Items = ", evodSet) sEvenCount = sOddCount = 0 for eoVal in evodSet: if(eoVal % 2 == 0): sEvenCount = sEvenCount + 1 else: sOddCount = sOddCount + 1 print("The Count of Even Numbers in evodSet = ", sEvenCount) print("The Count of Odd Numbers in evodSet = ", sOddCount)
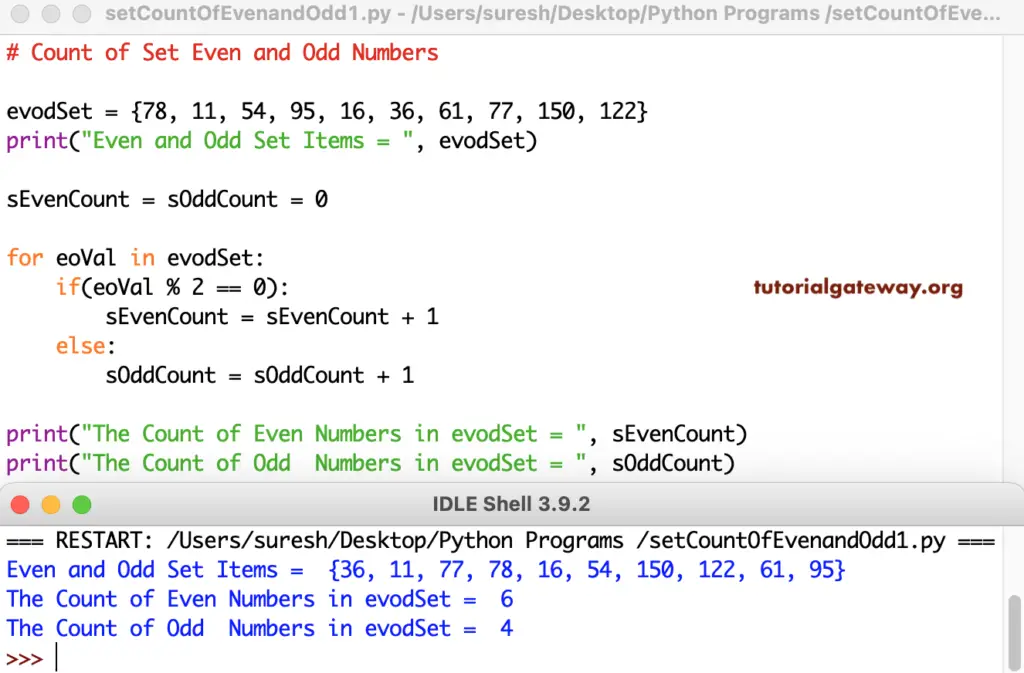
Python Program to Count Even and Odd Numbers in Set
This Python even and odd numbers example allows to enter the set items using the for loop range.
# Count of Set Even and Odd Numbers evodSet = set() number = int(input("Enter the Total Even Odd Set Items = ")) for i in range(1, number + 1): value = int(input("Enter the %d Set Item = " %i)) evodSet.add(value) print("Even and Odd Set Items = ", evodSet) sEvenCount = sOddCount = 0 for eoVal in evodSet: if(eoVal % 2 == 0): sEvenCount = sEvenCount + 1 else: sOddCount = sOddCount + 1 print("The Count of Even Numbers in evodSet = ", sEvenCount) print("The Count of Odd Numbers in evodSet = ", sOddCount)
Python Count Even and Odd Set Numbers output
Enter the Total Even Odd Set Items = 4
Enter the 1 Set Item = 22
Enter the 2 Set Item = 9
Enter the 3 Set Item = 32
Enter the 4 Set Item = 78
Even and Odd Set Items = {32, 9, 22, 78}
The Count of Even Numbers in evodSet = 3
The Count of Odd Numbers in evodSet = 1
In this Python Set example, we created a CountOfSetEvenandOddNumbers function that returns the Even and Odd numbers count.
# Count of Set Even and Odd Numbers def CountOfSetEvenandOddNumbers(evodSet): sEvenCount = sOddCount = 0 for eoVal in evodSet: if(eoVal % 2 == 0): sEvenCount = sEvenCount + 1 else: sOddCount = sOddCount + 1 return sEvenCount, sOddCount evodSet = set() number = int(input("Enter the Total Even Odd Set Items = ")) for i in range(1, number + 1): value = int(input("Enter the %d Set Item = " %i)) evodSet.add(value) print("Even and Odd Set Items = ", evodSet) sECount, sOCount = CountOfSetEvenandOddNumbers(evodSet) print("The Count of Even Numbers in evodSet = ", sECount) print("The Count of Odd Numbers in evodSet = ", sOCount)
Python Count Even and Odd Numbers in Set output
Enter the Total Even Odd Set Items = 6
Enter the 1 Set Item = 22
Enter the 2 Set Item = 33
Enter the 3 Set Item = 44
Enter the 4 Set Item = 55
Enter the 5 Set Item = 66
Enter the 6 Set Item = 88
Even and Odd Set Items = {33, 66, 44, 22, 55, 88}
The Count of Even Numbers in evodSet = 4
The Count of Odd Numbers in evodSet = 2