Write a Python program to Convert Tuple to String. We used the string join function to join or convert the tuple Items.
strTuple = ('p', 'y', 't', 'h', 'o', 'n') print("Tuple Items = ", strTuple) stringVal = ''.join(strTuple) print("String from Tuple = ", stringVal)
Tuple Items = ('p', 'y', 't', 'h', 'o', 'n')
String from Tuple = python
Python Program to Convert Tuple to String using for loop
In this Python Tuple to String example, we used the for loop (for t in strTuple) and for loop range (for i in range(len(strTuple))) to iterate the tuple items. Within the loop, we are concatenating each tuple item to a declared string.
strTuple = ('t', 'u', 't', 'o', 'r', 'i', 'a', 'l') print("Tuple Items = ", strTuple) strVal = '' for t in strTuple: strVal = strVal + t print("String from Tuple = ", strVal) stringVal = '' for i in range(len(strTuple)): stringVal = stringVal + strTuple[i] print("String from Tuple = ", stringVal)
Tuple Items = ('t', 'u', 't', 'o', 'r', 'i', 'a', 'l')
String from Tuple = tutorial
String from Tuple = tutorial
This example uses the lambda function to convert the tuple items to string.
from functools import reduce strTuple = ('t', 'u', 't', 'o', 'r', 'i', 'a', 'l', 's') print("Tuple Items = ", strTuple) strVal = reduce(lambda x, y: str(x) + str(y), strTuple, '') print("String from Tuple = ", strVal)
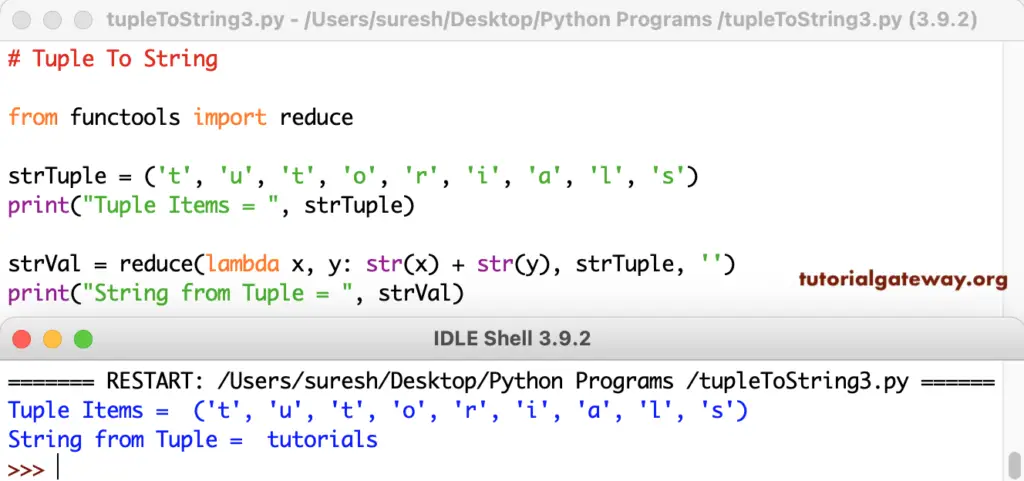