Write a Python program to check a number is a spy number or not using the for loop. For example, if the sum of digits equals the product of individual digits in a number, it is a spy. In this Python spy number example, we used a while loop to divide the number into digits and find the sum and the product of the digits. The if statement checks whether the sum equals product, if true, spy number.
Number = int(input("Enter the Number to Check Spy Number = ")) Temp = Number Sum = 0 prod = 1 while Temp > 0: lastDigit = Temp % 10 Sum = Sum + lastDigit prod = prod * lastDigit Temp = Temp // 10 print("The Sum of the Digits = %d" %Sum) print("The Product of the Digits = %d" %prod) if Sum == prod: print("\n%d is Spy Number." %Number) else: print("%d is Not a Spy Number." %Number)
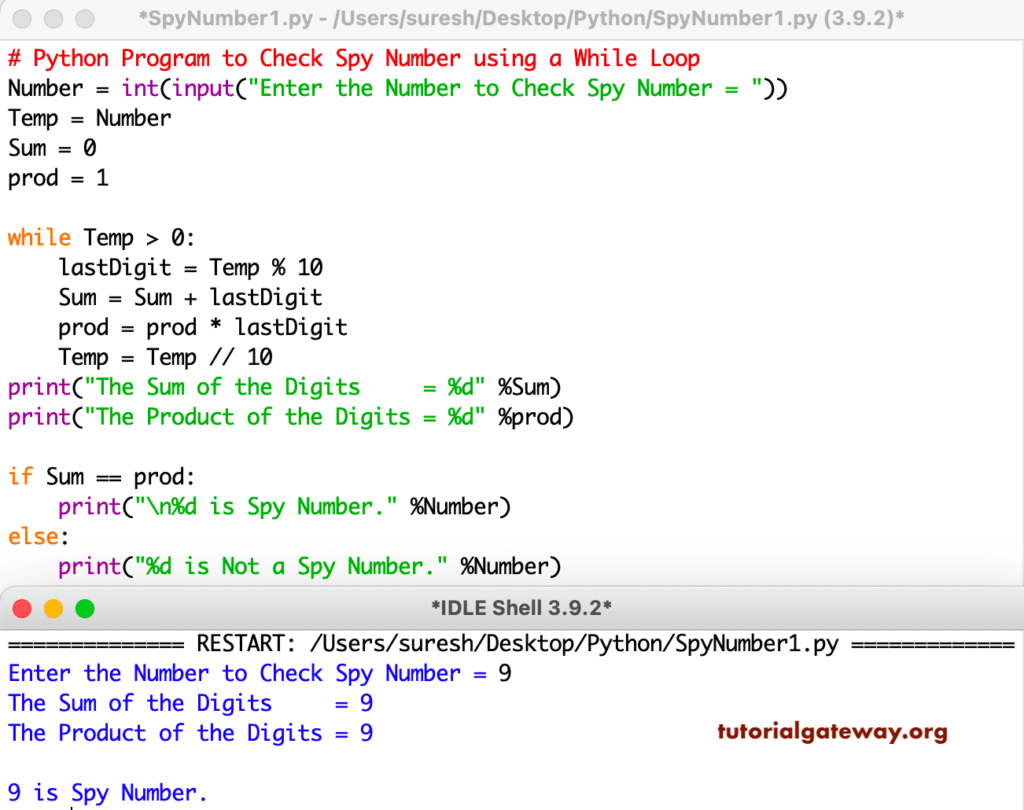
Python program to check whether a number is a spy number or not using recursion or recursive functions.
total = 0 prod = 1 reminder = 0 def spyNumber(number): global reminder, total, prod if(number > 0): reminder = number % 10 total = total + reminder prod = prod * reminder spyNumber(number // 10) return total, prod else: return 0 Number = int(input("Enter the Number = ")) total, prod = spyNumber(Number) print("The Sum of the Digits = %d" %total) print("The Product of the Digits = %d" %prod) if total == prod: print("\n%d is Spy Number." %Number) else: print("%d is Not." %Number)
Enter the Number = 1421
The Sum of the Digits = 8
The Product of the Digits = 8
1421 is Spy Number.
This Python program prints the spy numbers from 1 to n or within a range.
MinSpy = int(input("Please Enter the Minimum Value: ")) MaxSpy = int(input("Please Enter the Maximum Value: ")) for i in range(MinSpy, MaxSpy + 1): Temp = i Sum = 0 prod = 1 while Temp > 0: lastDigit = Temp % 10 Sum = Sum + lastDigit prod = prod * lastDigit Temp = Temp // 10 if Sum == prod: print(i, end = ' ')
Please Enter the Minimum Value: 1
Please Enter the Maximum Value: 10000
1 2 3 4 5 6 7 8 9 22 123 132 213 231 312 321 1124 1142 1214 1241 1412 1421 2114 2141 2411 4112 4121 4211