Write a Python program to check a number is a Harshad number or not using a while loop. If a number is divisible by the sum of the digits, it is a Harshad. For instance, 156 is divisible by 12 ( 1 + 5 + 6) so, it is a Harshad number.
Number = int(input("Enter the Number to Check Harshad Number = ")) Sum = 0 rem = 0 Temp = Number while Temp > 0: rem = Temp % 10 Sum = Sum + rem Temp = Temp // 10 print("The Sum of the Digits = %d" %Sum) if Number % Sum == 0: print("\n%d is a Harshad Number." %Number) else: print("%d is Not a Harshad Number." %Number)
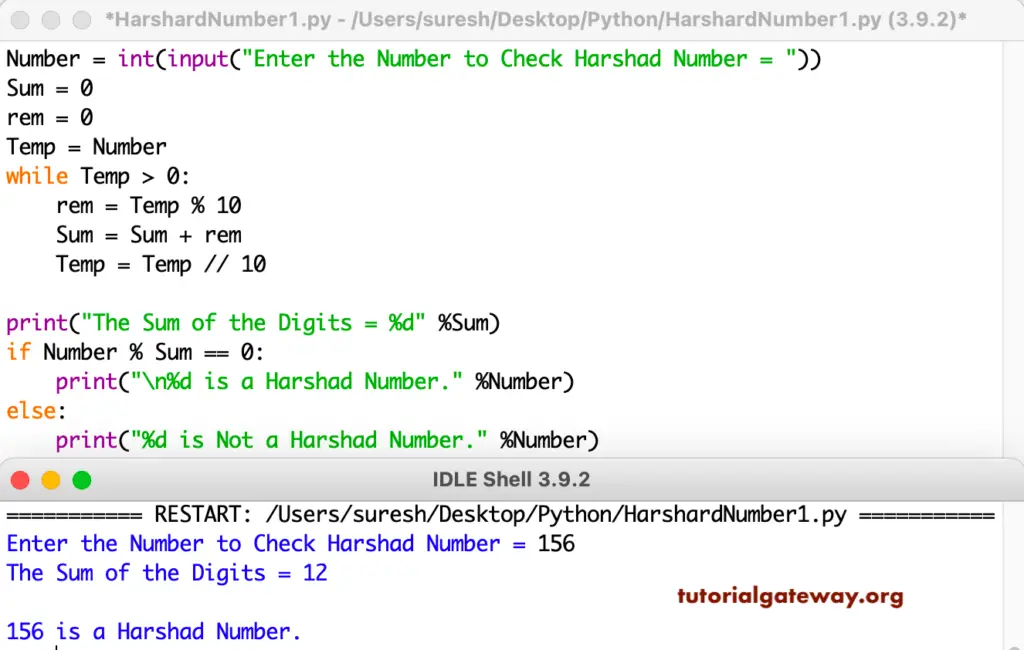
In this Python program, we used the while loop to divide the digit and calculate all digits’ sum. Next, the if condition checks whether the number is divisible by sum. If True, it is a Harshad number.
def digitsSum(Number): Sum = rem = 0 while Number > 0: rem = Number % 10 Sum = Sum + rem Number = Number // 10 return Sum Number = int(input("Enter the Number = ")) Sum = digitsSum(Number) print("The Sum of the Digits = %d" %Sum) if Number % Sum == 0: print("%d is a Harshad Number." %Number) else: print("%d is Not." %Number)
Enter the Number = 481
The Sum of the Digits = 13
481 is a Harshad Number.
Enter the Number = 472
The Sum of the Digits = 13
472 is Not.
Python program to check whether a given number is a Harshad or not using functions.
Sum = 0 def digitsSum(Number): global Sum if Number > 0: rem = Number % 10 Sum = Sum + rem digitsSum(Number // 10) return Sum Number = int(input("Enter the Number = ")) Sum = digitsSum(Number) if Number % Sum == 0: print("%d is a Harshad Number." %Number) else: print("%d is Not." %Number)
Enter the Number = 92
92 is Not.
Enter the Number = 448
448 is a Harshad Number.