Write a Python Program to Add an Item to the tuple. The Python tuple won’t allow you to add an item to the existing tuple. The below Python example will create a new tuple by concatenating the new item with the current tuple.
# Add an Item to Tuple intTuple = (10, 20, 30, 40, 50) print("Tuple Items = ", intTuple) intTuple = intTuple + (70,) print("Tuple Items = ", intTuple) intTuple = intTuple + (80, 90) print("Tuple Items = ", intTuple) intTuple = intTuple[2:5] + (11, 22, 33, 44) + intTuple[7:] print("Tuple Items = ", intTuple)
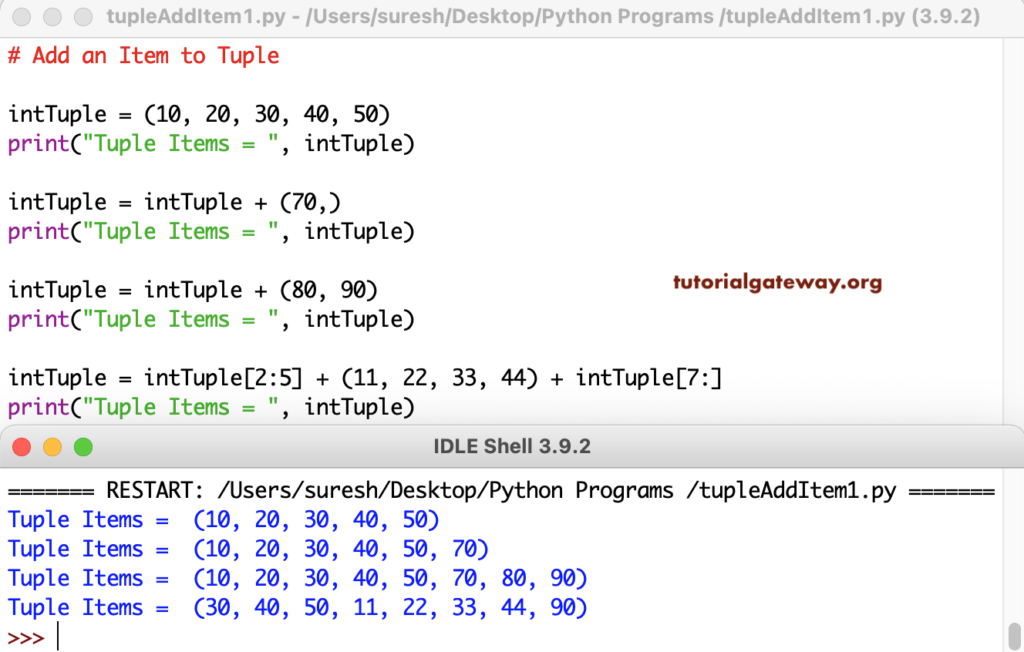
In this Python example, we allow the user to enter Tuple items and add them to an empty tuple. To achieve the same, we used the for loop range to iterate the tuple items.
# Add an Item to Tuple intTuple = () number = int(input("Enter the Total Number of Tuple Items = ")) for i in range(1, number + 1): value = int(input("Enter the %d Tuple value = " %i)) intTuple = intTuple + (value,) print("Tuple Items = ", intTuple)
Enter the Total Number of Tuple Items = 4
Enter the 1 Tuple value = 22
Enter the 2 Tuple value = 99
Enter the 3 Tuple value = 122
Enter the 4 Tuple value = 77
Tuple Items = (22, 99, 122, 77)