The SQL Else If statement is useful to check multiple conditions at once. SQL Else If statement is an extension to the If then Else (which we discussed in the earlier post).
If Else statement only executes the statements when the given condition is either true or False. But in the real world, we may have to check more than two conditions. In these situations, we can use SQL Else If statement. Let us see the syntax of the SQL Server Else if statement:
SQL Else If Syntax
The syntax of the Else If in SQL Server is
IF (Expression 1) BEGIN Statement 1; END ELSE IF (Expression 2) BEGIN Statement 2; END .......... ELSE BEGIN Default Statement; END
The SQL Server else if statement handles multiple statements effectively by executing them sequentially. It will check for the first condition. If the condition is TRUE, then it will execute the statements present in that block. If the condition is FALSE, then it will check the Next one (Else If condition) and so forth.
In Sql Server Else If examples, there will be some situations where condition 1, condition 2 is TRUE, for example:
x = 30, y = 15
Condition 1: x > y — TRUE
Condition 2: x != y — TRUE
In these situations, statements under the Condition 1 executed. Because ELSE IF conditions only execute if it’s previous IF or ELSE IF statement fails.
SQL Else If Flow chart
Let us see the flow chart of the SQL server else if statement for better understanding.
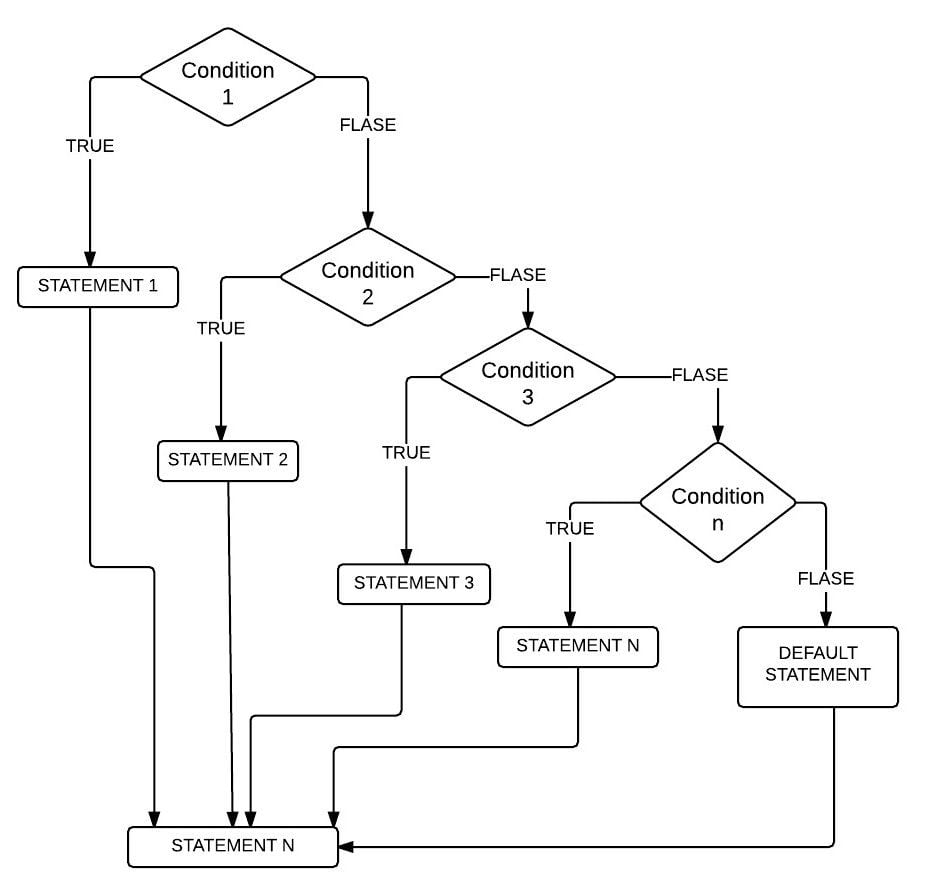
SQL Else If Example
In this program, We are going to calculate whether he is eligible for a scholarship or not using SQL Else if statement
-- SQL Else If Example --Declaring Total Marks Out of 1000 DECLARE @Total_Marks INT = 940 ; IF @Total_Marks > = 900 BEGIN PRINT ' Congratulations '; PRINT ' You are eligible for Full Scholarship '; END ELSE IF @Total_Marks > = 800 BEGIN PRINT ' Congratulations '; PRINT ' You are eligible for 50 Percent Scholarship '; END ELSE IF @Total_Marks > = 750 BEGIN PRINT ' Congratulations '; PRINT ' You are eligible for 10 Percent Scholarship '; END ELSE BEGIN PRINT ' You are Not eligible for Scholarship '; PRINT ' We are really Sorry for You '; END
OUTPUT 1: In this SQL Server Else If demo, the Total_Marks = 940. It means First If condition is TRUE that’s why statements inside the If Statement displayed as Browser output
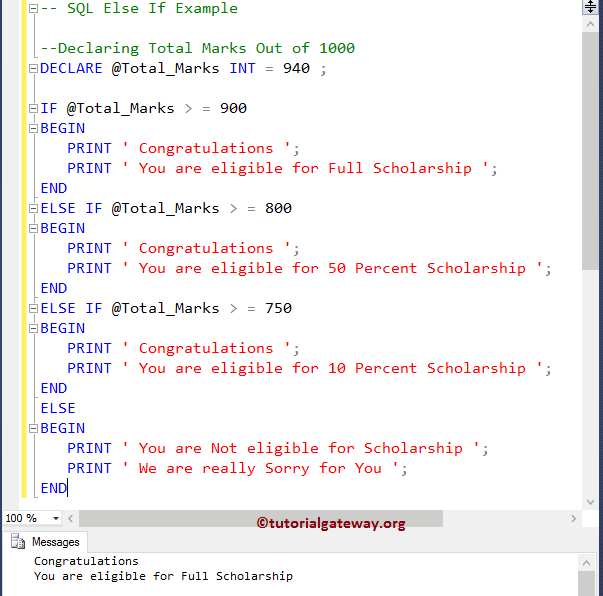
OUTPUT 2: Let us change the Total_Marks from 940 to 820 to show the SQL else if statement example. Here, first IF condition Fails so, It will check the else if (@Total_Marks >= 800), which is TRUE. So it will display the statements within this block. Although else if (@Total_Marks >= 750) condition is TRUE, but it won’t check that condition.
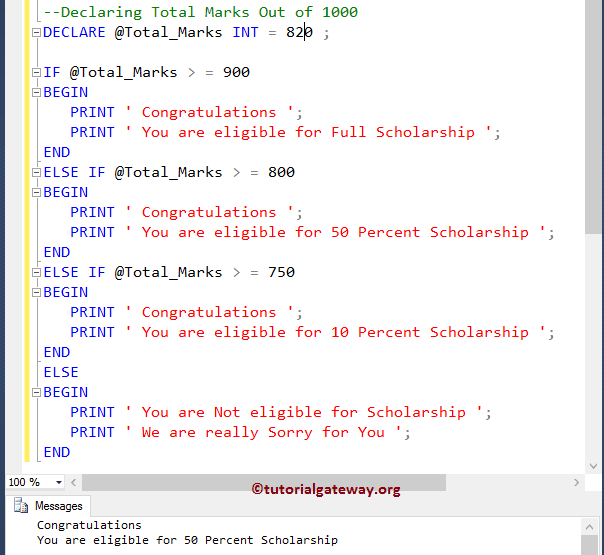
OUTPUT 3: Let us change the Total_Marks from 820 to 760, which means first IF condition and Else if (@Total_Marks >= 800) fails. So, It will check the else if (@Total_Marks >= 750), which is TRUE, so that it will print the statements inside this block.
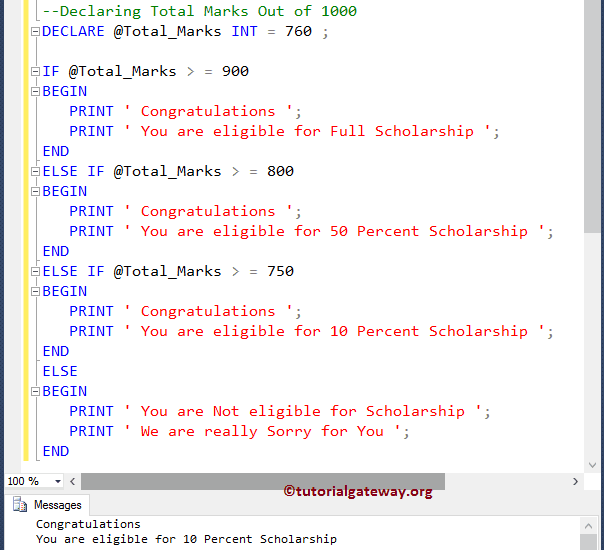
OUTPUT 4: We are going to change the Total_Marks to 735. It means all the IF and Else If conditions Fail. So, It will print statements inside the else block.
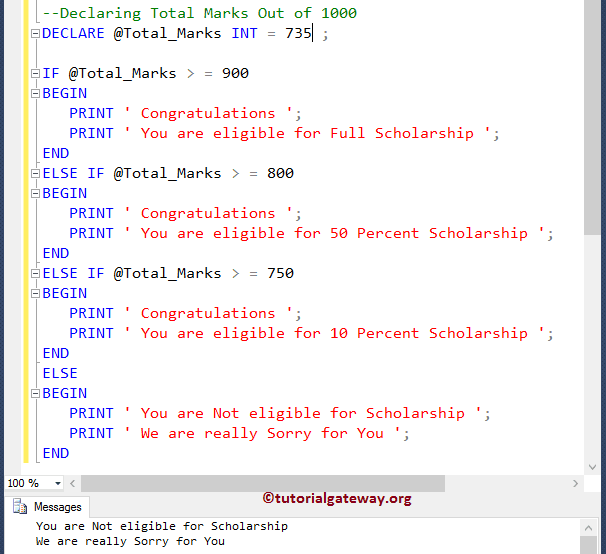
I suggest you to refer the If then Else article in SQL Server