The R repeat executes the statements inside the code block multiple number times. Repeat loop in R programming doesn’t provide any condition to check, so we have to give the condition to exit from the loop.
This article shows you how to use repeat in R Programming with an example. Before we get into the case, let us see the syntax.
R repeat loop Syntax
The syntax of the repeat in R Programming language is
repeat { statement 1 statement 2 …………. statement N # Please provide Condition to exit or use Break Statement } #This statement is from Outside
First, it will execute the statements inside the loop, and if there are any loop breaking statements, then it will exit from the loop.
Flow Chart
The following picture will show you the flow chart behind the R repeat.
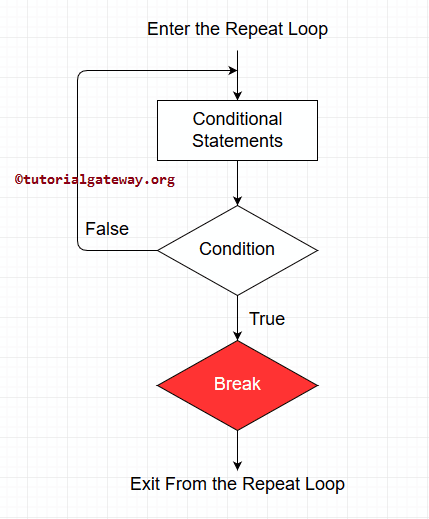
The R repeat Flow chart sequence is:
- First, we initialize our variables. Next, it will enter into the Repeat loop.
- It will execute the group of statements inside the loop.
- Next, we have to use any expression inside the loop to exit.
- Now it will check for the condition. If the condition is True, then it executes the Break statement exit from the loop.
- If the condition is False, the statements inside the Repeat loop are executed again.
R repeat Loop example
This program helps us to understand R repeat. It allows the user to enter an integer value below 10. Using this value, it adds those values up to 10.
# Example total <- 0 number <- as.integer(readline(prompt="Please Enter any integer Value below 10: ")) repeat { total = total + number number = number + 1 if (number > 10) { break } } print(paste("The total Sum of Numbers From the Repeat Loop is: ", total))
In this R Programming example, We are going to enter the number = 7. It means total = 7 + 8 + 9 + 10 = 34
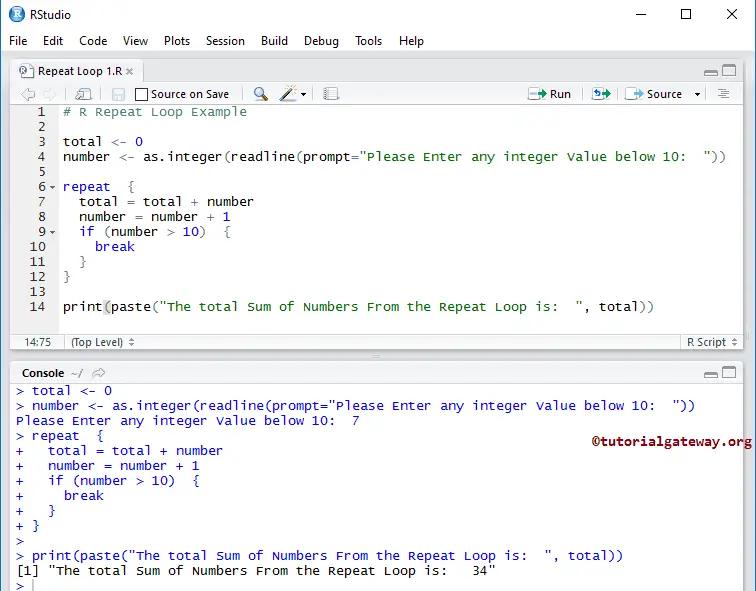
Within the following statements, First, we declared the total variable and assigned it to Zero. Next, it will ask the user to enter any integer value below 10, and we are assigning the user entered value to a number variable.
total <- 0 number <- as.integer(readline(prompt="Please Enter any integer Value below 10: "))
Next line, we used the While loop, and the expression inside the While loop will make sure that the given number is less than or equal to 10.
repeat { total = total + number number = number + 1 }
In this R repeat example, the User Entered value: number = 7, and we initialized the total = 0
First Iteration
- total= total + number
- total= 0 + 7 ==> 7
Next, the number will be incremented by 1 (number= number + 1). Please refer R Arithmetic Operators article to understand this + notation.
Second Iteration
Within the first Iteration, the values of both the number and total changed as number= 8 and total = 7
- total = total + number
- total= 7 + 8 ==> 15
Next, the number incremented by 1.
Third Iteration
Within the second Iteration of R repeat, the values of both the number and total changed as number = 9 and total = 15
- total = total +number
- total = 15 + 9 ==> 24
Next, number= number + 1
Fourth Iteration
Within the third Iteration, the values of both the number and total changed as number= 10 and total = 24
- total = total + number
- total = 24 + 10 ==> 34
Next, number= number + 1
Here, Number= 11, and the condition present inside the If Statement (number > 10) is True. So, the Break statement is executed, and it will exit from the loop.
if (number> 10) { break }
The last print statement prints the total sum of digits present in the given number as output.
print(paste("The total Sum of Numbers From the While Loop is: ", total))
Infinite Repeat in R Programming
If you forgot to use the Conditions or Break statement to terminate the Repeat loop, then statements inside the loop are executed infinite times (also called an infinite loop).
# Infinite Repeat Example total <- 0 number <- as.integer(readline(prompt="Please Enter any integer Value: ")) repeat { total = total + number number = number + 1 print(number) }
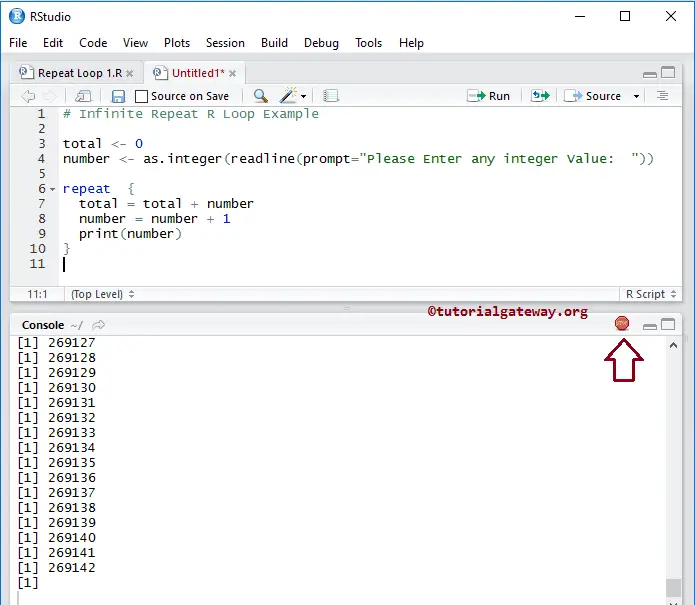
In this example, there is no condition to check or no break statement to break the loop, so the R repeat loop will go on executing the statements infinite times. Now, let us add the Break statement inside the If statement.
total <- 0 number <- as.integer(readline(prompt="Please Enter any integer Value below 10: ")) repeat { total = total + number number = number + 1 if (number > 15) { break } print(number) }
Now, when it reaches 15, the condition will fail. Let us see the output.
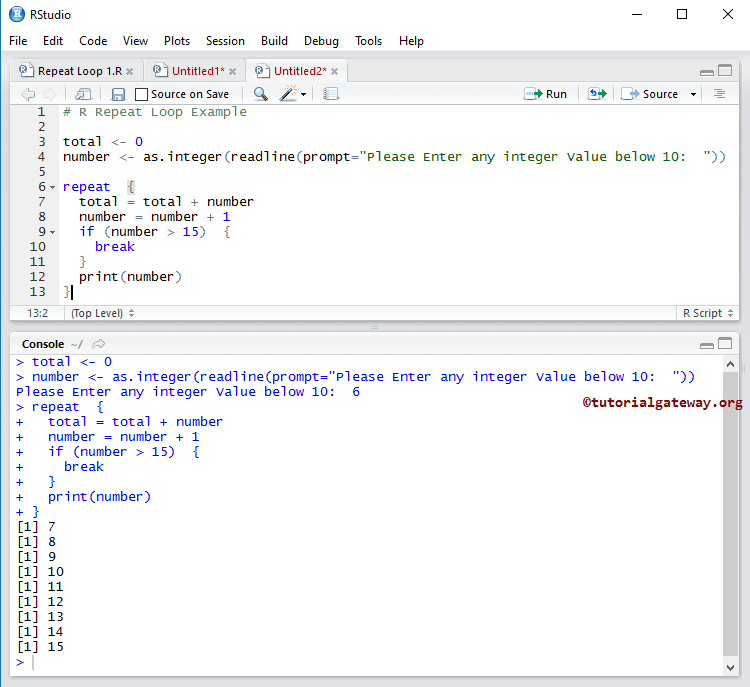