SQL Server Transaction is helpful for executing one more statement as a set. If it is successful, all the changes made in that transaction will apply to the table. If any single statement in the SQL Server transaction encounters an error, then changes made will be erased or rolled back.
Let me show you the list of various examples that can explain Transactions. They are BEGIN TRANSACTION, COMMIT, ROLLBACK, named, Trans inside the IF ELSE, and SQL Server Transactions inside the TRY CATCH block. List of things to remember while working on the Transactions.
- Every trans should start with BEGIN TRANSACTION, BEGIN TRAN, or BEGIN TRANSACTION Transaction_Name
- Every Transaction in SQL Server must end with either COMMIT or ROLLBACK statements.
- COMMIT TRANSACTION: This statement tells the server to save the changes made between the BEGIN and COMMIT. There are multiple ways to write this statement. Either you can write COMMIT, COMMIT TRAN, COMMIT TRANSACTION, or COMMIT TRANSACTION Transaction_Name
- ROLLBACK TRANSACTION: This statement tells the SQL Server to erase all the changes between the BEGIN and ROLLBACK. There are multiple ways to write this statement. You can write ROLLBACK, ROLLBACK TRAN, ROLLBACK TRANSACTION, or ROLLBACK TRANSACTION Transaction_Name.
SQL Server Transaction Example
In this SQL Server example, we will place an INSERT INTO SELECT statement inside the BEGIN and COMMIT transaction. As you can see, it will select the top four records from the Employee table and store them in the Employee Records table.
BEGIN TRAN INSERT INTO [dbo].[EmployeeRecords] ( [EmpID], [FirstName], [LastName], [Education], [Occupation], [YearlyIncome], [Sales]) SELECT TOP 4 [EmpID], [FirstName], [LastName], [Education], [Occupation], [YearlyIncome], [Sales] FROM [dbo].[Employee] COMMIT TRANSACTION
Run the above query
Messages
--------
(4 row(s) affected)
Let us see the inserted rows in the Employee Records table.
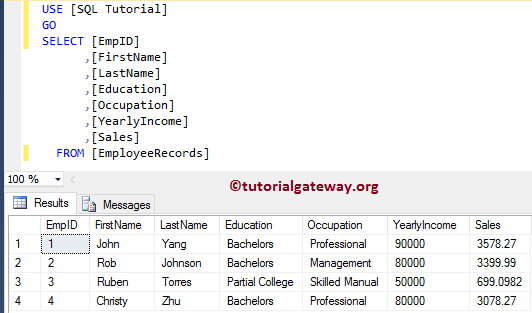
Transaction in the SQL server is not limited to a single statement. We can place multiple statements inside one trans. In this SQL Server example, we will put one Insert Statement and one Update Statement.
BEGIN TRANSACTION INSERT INTO [dbo].[EmployeeRecords] ( [EmpID], [FirstName], [LastName], [Education], [Occupation], [YearlyIncome], [Sales]) VALUES (5, 'SQL', 'Server', 'Education', 'Teaching', 10000, 200) UPDATE [dbo].[EmployeeRecords] SET [Education] = 'Tutorials', [YearlyIncome] = 98000 WHERE [EmpID] = 5 COMMIT TRANSACTION
Run the Insert and Update statements in a single query
Messages
--------
(1 row(s) affected)
(1 row(s) affected)
The records are in the Employee table after that.
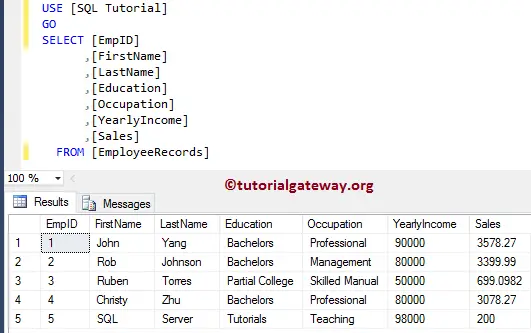
Auto Rollback in SQL Server Transaction
The statements inside a SQL Server transaction are executed as a set; if one statement fails, the remaining statements will not execute. This process is also called Auto Rollback transactions.
This time we will deliberately fail one statement inside the SQL Server Transaction. As you can see, the update statement will return an error because we are entering the string (VARCHAR) information into the float data type.
BEGIN TRANSACTION INSERT INTO [dbo].[EmployeeRecords] ( [EmpID], [FirstName], [LastName], [Education], [Occupation], [YearlyIncome], [Sales]) VALUES (6, 'Tutorial', 'Gateway', 'Education', 'Learning', 65000, 1400) UPDATE [dbo].[EmployeeRecords] SET [Education] = 'Masters', [YearlyIncome] = 'Wrong Data' WHERE [EmpID] = 6 COMMIT TRANSACTION
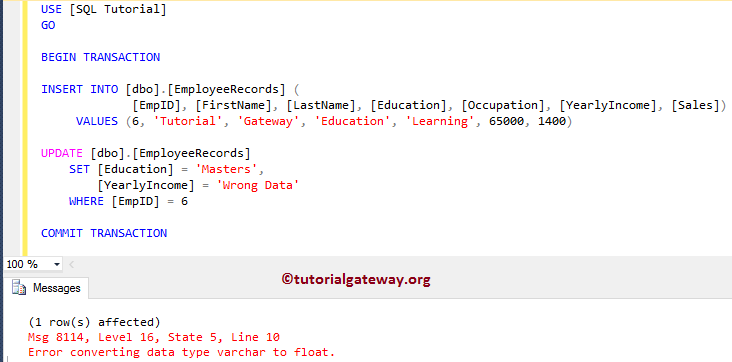
Though, there is an error in the Update statement. We cannot see the inserted record because the SQL Server transaction is rolled back.
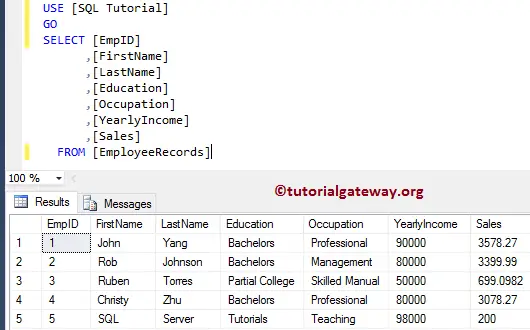
Let me remove the BEGIN and COMMIT from the above code snippet and execute the statements.
INSERT INTO [dbo].[EmployeeRecords] ( [EmpID], [FirstName], [LastName], [Education], [Occupation], [YearlyIncome], [Sales]) VALUES (6, 'Tutorial', 'Gateway', 'Education', 'Learning', 65000, 1400) UPDATE [dbo].[EmployeeRecords] SET [Education] = 'Masters', [YearlyIncome] = 'Wrong Data' WHERE [EmpID] = 6
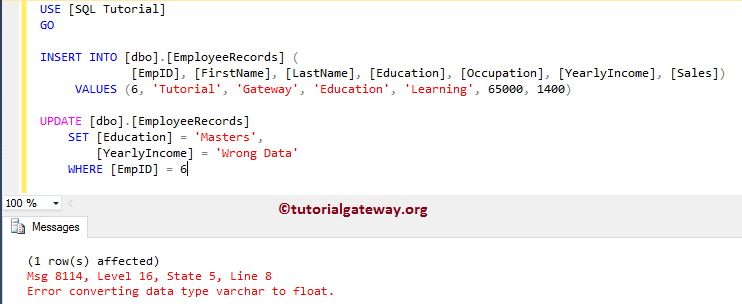
I have inserted the new record of Emp Id 6 but failed to update it.
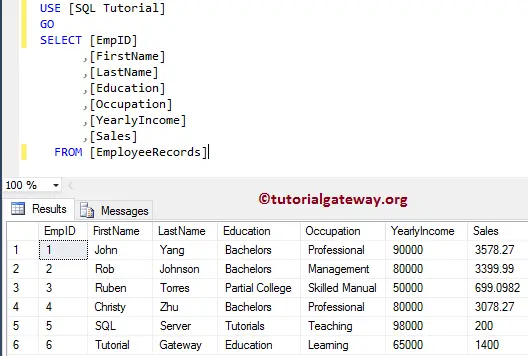
Rollback Transaction in SQL Server
SQL Rollback is useful to roll back to the transaction’s beginning or save point. You can use this Rollback to remove the half-completed rows or to handle errors.
For example, if your transaction inserts a new record and throws an error, then you can use this rollback to revert the table to the original position.
In this example, we will insert a new record into the Employee table and apply the rollback transaction after the insert. We use SQL Server Select Statement inside and outside the transaction to demonstrate this. The first select statement will show you the table records before completing the transaction.
BEGIN TRANSACTION INSERT INTO [dbo].[EmployeeRecords] ( [EmpID], [FirstName], [LastName], [Education], [Occupation], [YearlyIncome], [Sales]) VALUES (7, 'SQL Server', 'Tutorial', 'Masters', 'Learn', 55000, 1250) SELECT * FROM [dbo].[EmployeeRecords] ROLLBACK TRANSACTION SELECT * FROM [dbo].[EmployeeRecords]
Though there is no error in the above statement, it has not inserted the record.
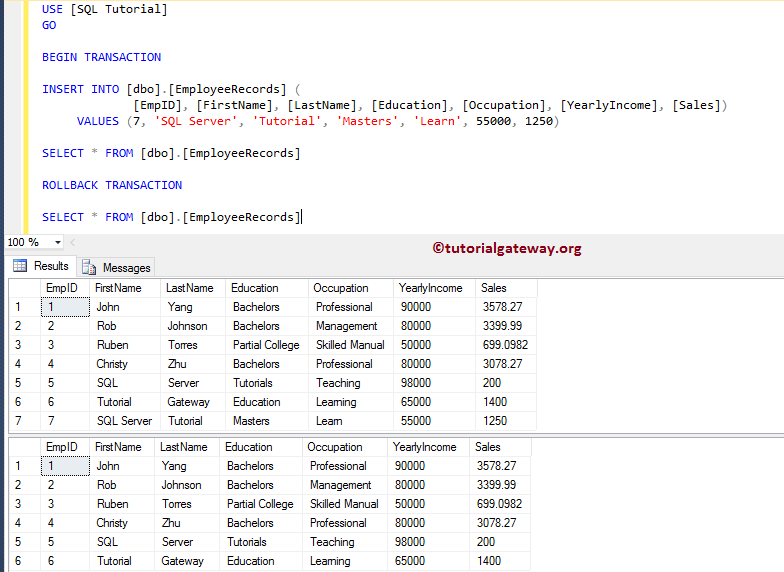
Named Transactions in SQL Server
It allows you to name your transaction. When working with multiple trans in one query, it is always advisable to use the named transaction.
-- AddEmployee is the Transaction name BEGIN TRANSACTION AddEmployee INSERT INTO [dbo].[EmployeeRecords] ( [EmpID], [FirstName], [LastName], [Education], [Occupation], [YearlyIncome], [Sales]) VALUES (7, 'SQL Server', 'Tutorial', 'Masters', 'Learn', 55000, 1250) COMMIT TRANSACTION AddEmployee SELECT * FROM [dbo].[EmployeeRecords]
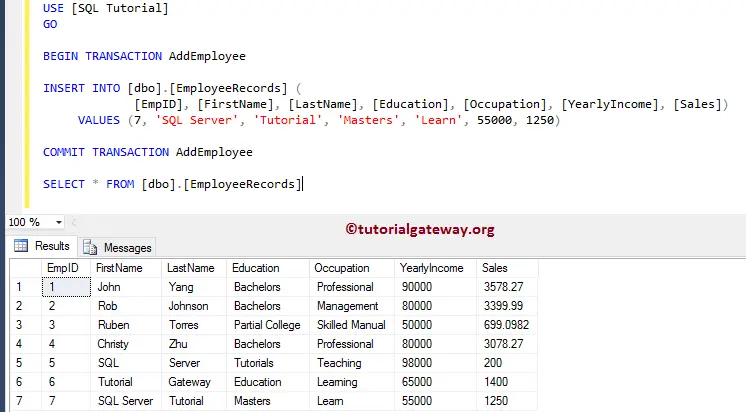
SQL Transaction in IF ELSE statement
The Transactions are much more useful if we place them inside any conditional statements such as IF ELSE. For instance, check for the existing records in the employee table before the insertion, and if it is there, rollback, else commit, etc.
The below statement is a simple SQL Server Transactions example, where we declare a variable (assuming the user will enter the value). Next, we used the insert statement, next within the If we are checking whether the @sales is less than 1000 or not. If it is true, ROLLBACK the transaction; otherwise, Commit it in the Select statement.
DECLARE @Sales FLOAT = 250.0 BEGIN TRANSACTION AddEmployee INSERT INTO [dbo].[EmployeeRecords] ( [EmpID], [FirstName], [LastName], [Education], [Occupation], [YearlyIncome], [Sales]) VALUES (8, 'Dave', 'Rob', 'High School', 'Professional', 85000, @Sales) IF @Sales < 1000 BEGIN ROLLBACK TRANSACTION AddEmployee PRINT 'Sorry! Sales Amount Should be More than 1000' END ELSE BEGIN COMMIT TRANSACTION AddEmployee PRINT 'Inserted the Record Successfully' END SELECT * FROM [dbo].[EmployeeRecords]
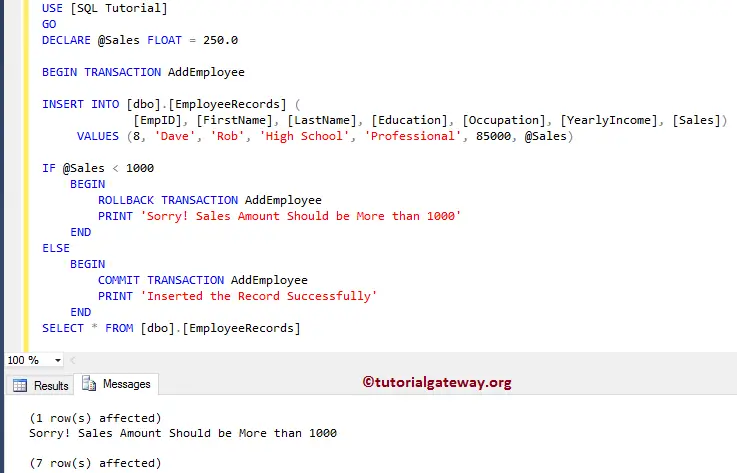
Let me show you the records.
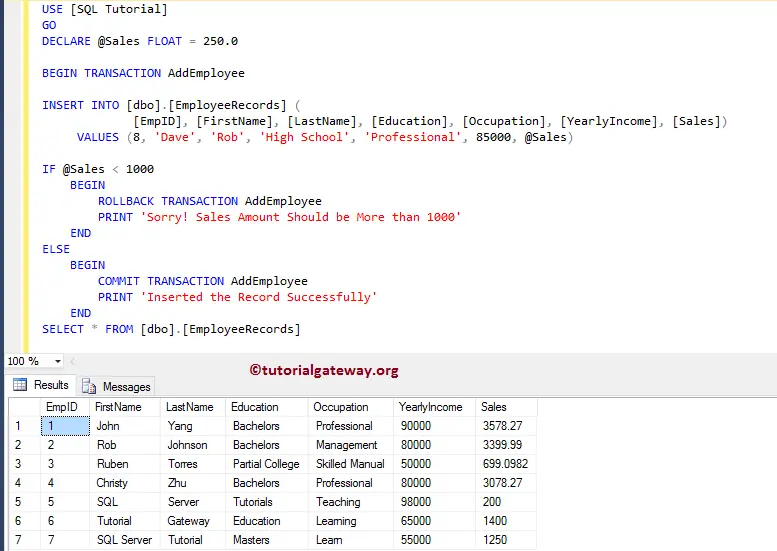
Change Values
This time we changed the variable @Sales value to 1450.02
DECLARE @Sales FLOAT = 1450.02 BEGIN TRANSACTION AddEmployee INSERT INTO [dbo].[EmployeeRecords] ( [EmpID], [FirstName], [LastName], [Education], [Occupation], [YearlyIncome], [Sales]) VALUES (8, 'Dave', 'Rob', 'High School', 'Professional', 85000, @Sales) IF @Sales < 1000 BEGIN ROLLBACK TRANSACTION AddEmployee PRINT 'Sorry! Sales Amount Should be More than 1000' END ELSE BEGIN COMMIT TRANSACTION AddEmployee PRINT 'Inserted the Record Successfully' END SELECT * FROM [dbo].[EmployeeRecords]
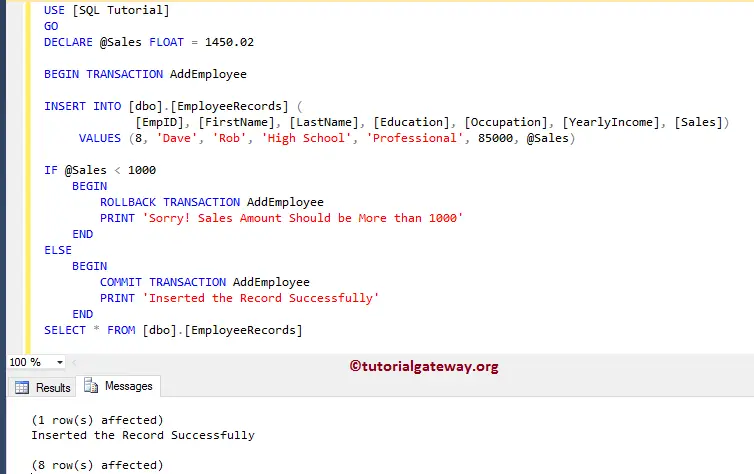
The result data is in the employee table.
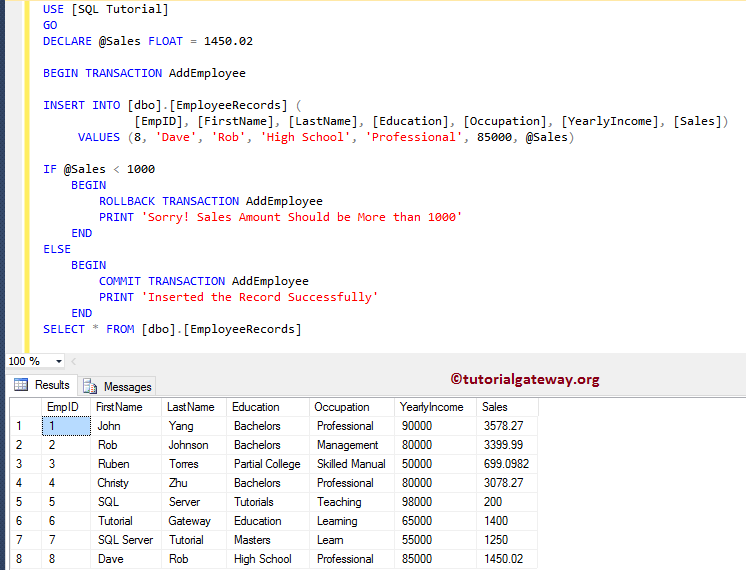
SQL Transaction in TRY CATCH
Transactions are very useful if we place them inside the TRY CATCH block. For instance, if there is an error inside, you can use the catch block to roll back the transaction to the original position.
It is a simple Transactions example in SQL Server, where we placed one select and one update statement inside the Try Catch block. If you observe closely, our update statement will throw an error because of data type conflict.
BEGIN TRY BEGIN TRANSACTION AddEmployee INSERT INTO [dbo].[EmployeeRecords] ( [EmpID], [FirstName], [LastName], [Education], [Occupation], [YearlyIncome], [Sales]) VALUES (9, 'Chong', 'Lee', 'Tutorials', 'Developer', 66500, 1950) UPDATE [dbo].[EmployeeRecords] SET [Education] = 'Bachelors', [YearlyIncome] = 'Hey You gotme Wrong!' WHERE [EmpID] = 9 COMMIT TRANSACTION AddEmployee PRINT 'Inserted the Record Successfully' END TRY BEGIN CATCH ROLLBACK TRANSACTION AddEmployee PRINT 'Sorry! There is a Data Type Mismatch for yearly Income' END CATCH SELECT * FROM [dbo].[EmployeeRecords]
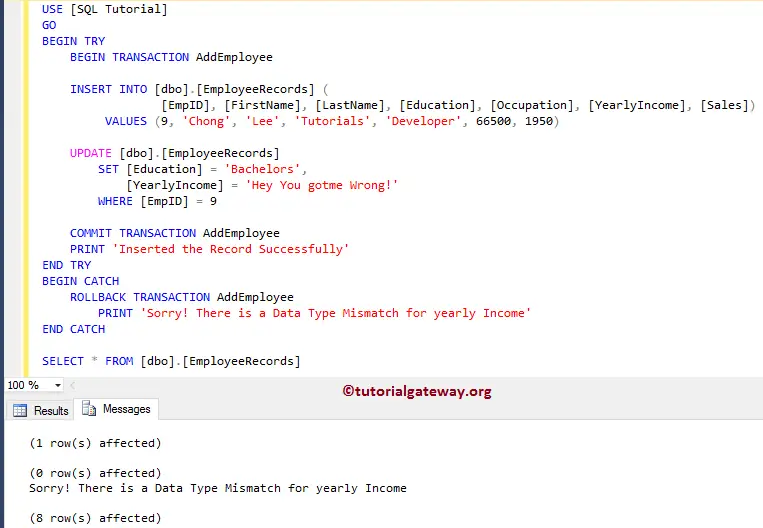
and the data inside the table is:
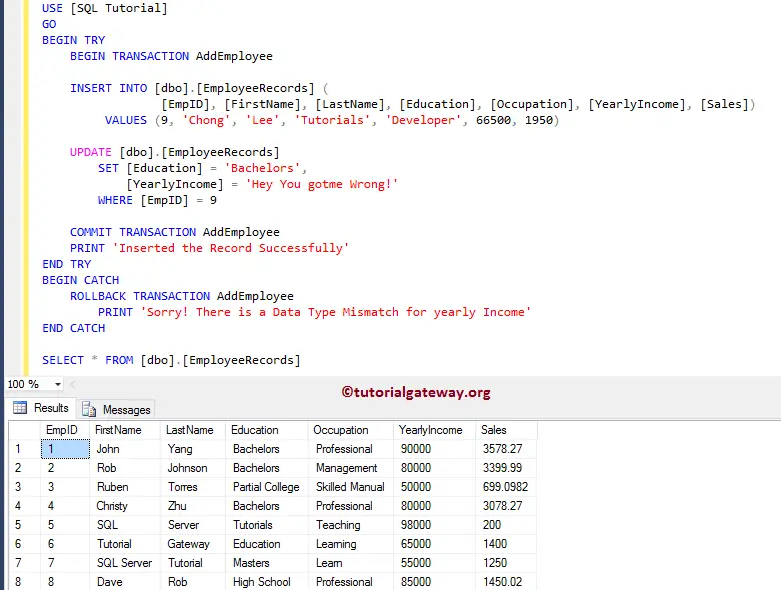
Let me change the yearly income value to 91567 and run it
BEGIN TRY BEGIN TRANSACTION AddEmployee INSERT INTO [dbo].[EmployeeRecords] ( [EmpID], [FirstName], [LastName], [Education], [Occupation], [YearlyIncome], [Sales]) VALUES (9, 'Chong', 'Lee', 'Tutorials', 'Developer', 66500, 1950) UPDATE [dbo].[EmployeeRecords] SET [Education] = 'Bachelors', [YearlyIncome] = 91567 -- We changed it WHERE [EmpID] = 9 COMMIT TRANSACTION AddEmployee PRINT 'Inserted the Record Successfully' END TRY BEGIN CATCH ROLLBACK TRANSACTION AddEmployee PRINT 'Sorry! There is a Data Type Mismatch for yearly Income' END CATCH SELECT * FROM [dbo].[EmployeeRecords]
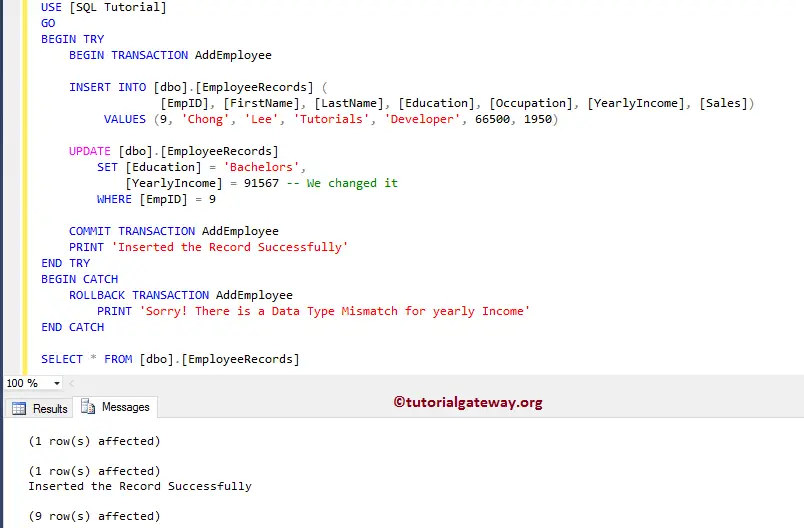
and the data inside the Employee table is:
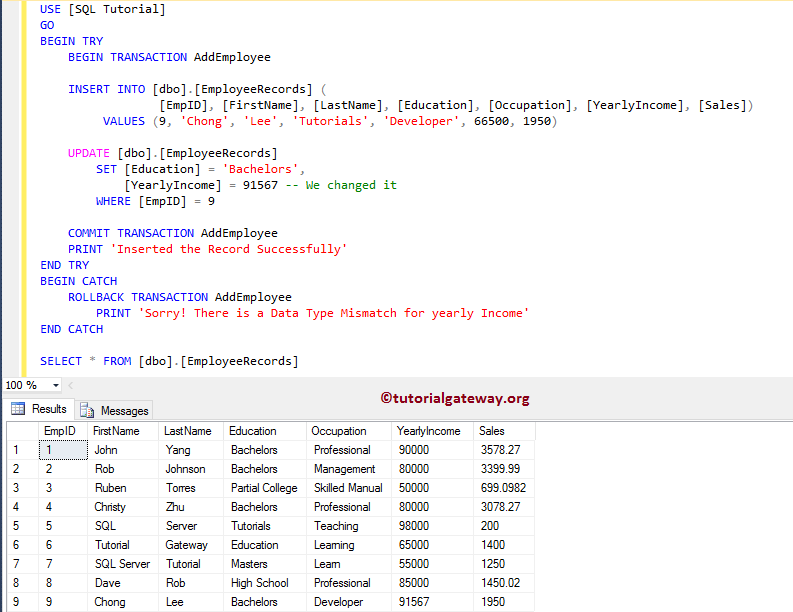
Common Mistakes
One of the common mistakes that will happen while using these in a query. As you can see, we use a simple Update statement after the SQL BEGIN TRANSACTION but forgot to mention COMMIT or ROLLBACK.
BEGIN TRANSACTION UPDATE [dbo].[EmployeeRecords] SET [YearlyIncome] = 125896 WHERE [EmpID] = 6
Messages
--------
(1 row(s) affected)
Let me open one more query window (click New query in SSMS) and write a simple select statement to select all the records in the Employee Records table.
See, the execution is taking longer than usual. It is because, by default, the Select statement will return the committed data, and we forgot to commit the update.
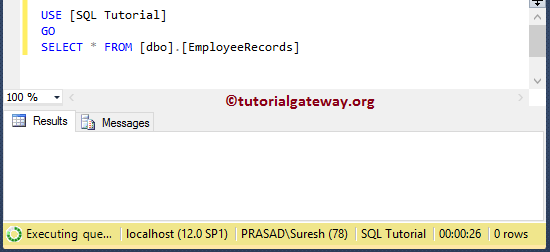
Let me set the SQL Server Transaction Isolation level to Read Uncommitted. It will read the uncommitted data.
SET TRANSACTION ISOLATION LEVEL READ UNCOMMITTED SELECT * FROM [dbo].[EmployeeRecords]
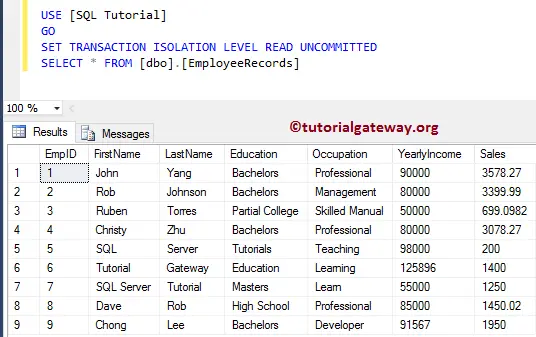
Nested Transactions in SQL Server
In our previous article, we explained everything about the Transactions with examples. Here, we show you the Nested Transactions in SQL Server and how to create them with examples. We will also discuss the Transaction Save Points.
From the screenshot below, we created a new table to demonstrate the SQL Server Nested Transactions.
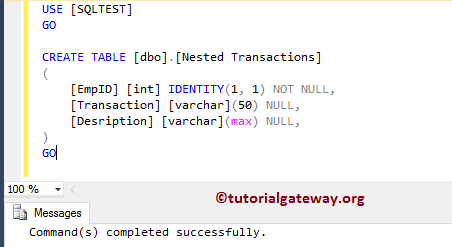
Simple Nested Transactions in SQL Server Example
In this SQL Server Nested Transactions example, we will use the @@TRANCOUNT to display the number of transactions at each layer. It is a simple example without any problem.
SELECT 'Before Staring any Transaction', @@TRANCOUNT BEGIN TRANSACTION TRAN1 SELECT 'After Staring First Transaction', @@TRANCOUNT -- Second Transaction Start BEGIN TRANSACTION TRAN2 SELECT 'After Staring Second Transaction', @@TRANCOUNT COMMIT TRANSACTION TRAN2 -- End Of Second Transaction SELECT 'After Commiting the Second Transaction', @@TRANCOUNT COMMIT TRANSACTION TRAN1 SELECT 'After Commiting the First Transaction', @@TRANCOUNT
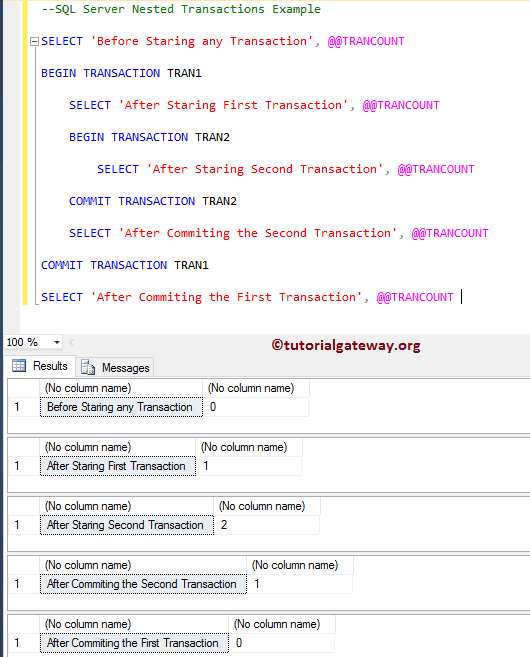
Let me show you a practical example of this Nested Transaction. In this example, we are using two INSERT Statements. One is inside the Main transaction, and another one is in Nested Transaction.
USE [SQLTEST] GO BEGIN TRANSACTION TRAN1 INSERT INTO [dbo].[Nested Transactions] ([Transaction], [Desription]) VALUES ('Tran1', 'This is Outer Transaction 1') BEGIN TRANSACTION TRAN2 INSERT INTO [dbo].[Nested Transactions] ([Transaction], [Desription]) VALUES ('Tran2', 'This is Inner Transaction 1') COMMIT TRANSACTION TRAN2 COMMIT TRANSACTION TRAN1 SELECT [EmpID], [Transaction], [Desription] FROM [Nested Transactions]
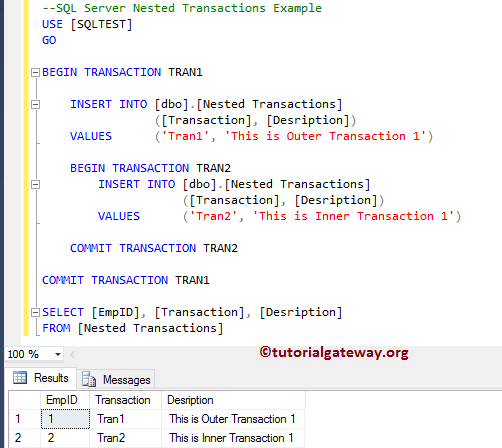
Nested Transactions in SQL ServerWith Rollback
In this example, we are checking what will happen if we use the ROLLBACK TRANSACTION as the nested transaction.
SELECT 'Before Staring any Transaction', @@TRANCOUNT BEGIN TRANSACTION TRAN1 SELECT 'After Staring First Transaction', @@TRANCOUNT BEGIN TRANSACTION SELECT 'After Staring Second Transaction', @@TRANCOUNT ROLLBACK TRANSACTION SELECT 'After Rollback the Second Transaction', @@TRANCOUNT COMMIT TRANSACTION TRAN1 SELECT 'After Commiting the First Transaction', @@TRANCOUNT
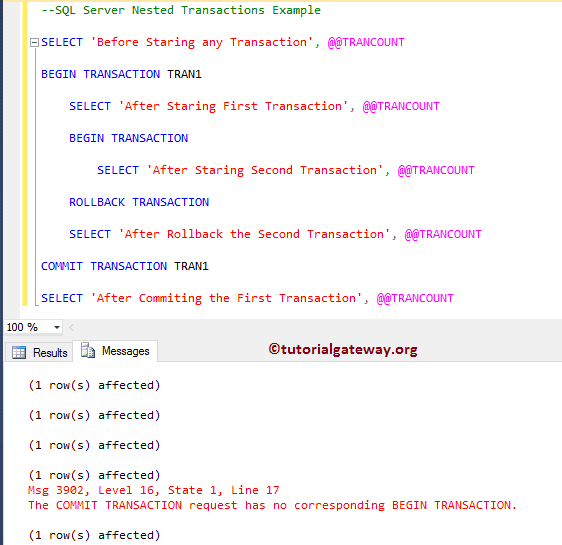
As you can see, it throws an error message for the Last COMMIT TRANSACTION TRAN1 statement because ROLLBACK will roll back all the statements.
You can observe that no transactions are running after the Rollback is executed.
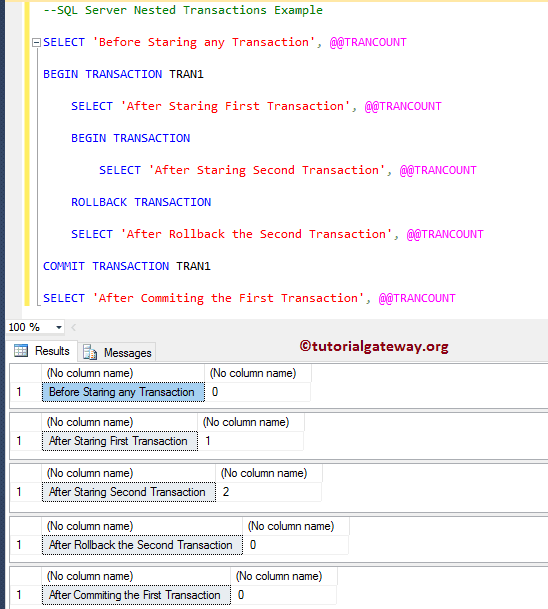
In this example, we are using two INSERT Statements. One is inside the Main transaction, and another one is in Nested Transaction.
USE [SQLTEST] GO BEGIN TRANSACTION TRAN1 INSERT INTO [dbo].[Nested Transactions] ([Transaction], [Desription]) VALUES ('Tran3', 'This is Outer Transaction 2') BEGIN TRANSACTION INSERT INTO [dbo].[Nested Transactions] ([Transaction], [Desription]) VALUES ('Tran4', 'This is Inner Transaction 2') ROLLBACK TRANSACTION COMMIT TRANSACTION TRAN1 SELECT [EmpID], [Transaction], [Desription] FROM [Nested Transactions]
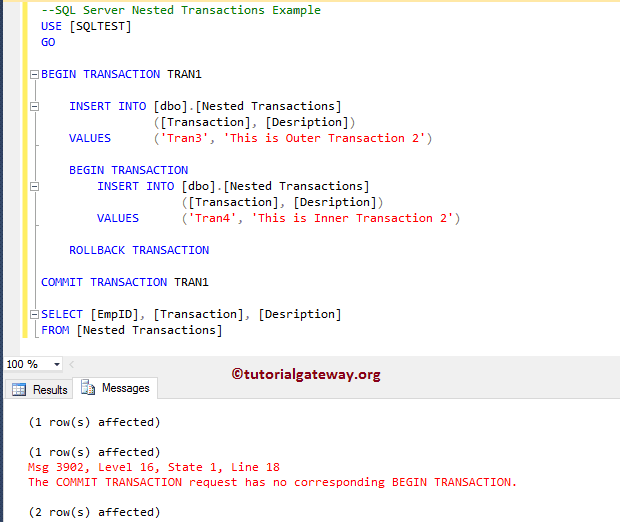
From the below screenshot, see that there is no insertion happened.
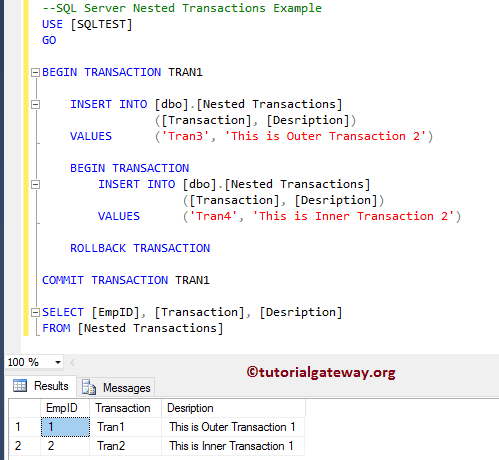
SQL Nested Transactions With Rollback as Outer
Here, we are checking what happens if we use the ROLLBACK TRANSACTION as the outer transaction.
SELECT 'Before Staring any Transaction', @@TRANCOUNT BEGIN TRANSACTION SELECT 'After Staring First Transaction', @@TRANCOUNT BEGIN TRANSACTION TRAN2 SELECT 'After Staring Second Transaction', @@TRANCOUNT COMMIT TRANSACTION TRAN2 SELECT 'After Commiting the Second Transaction', @@TRANCOUNT ROLLBACK TRANSACTION SELECT 'After the First Transaction is Rollbacked', @@TRANCOUNT
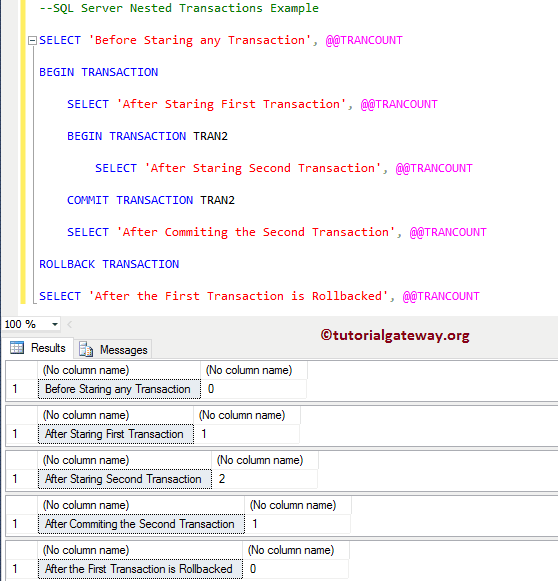
Let me show you one practical nested transactions example.
USE [SQLTEST] GO BEGIN TRANSACTION INSERT INTO [dbo].[Nested Transactions] ([Transaction], [Desription]) VALUES ('Tran4', 'This is Outer Transaction 4') BEGIN TRANSACTION INSERT INTO [dbo].[Nested Transactions] ([Transaction], [Desription]) VALUES ('Tran5', 'This is Inner Transaction 5') COMMIT TRANSACTION ROLLBACK TRANSACTION SELECT [EmpID], [Transaction], [Desription] FROM [Nested Transactions]
It will roll back everything inside the first transaction and the second transaction.
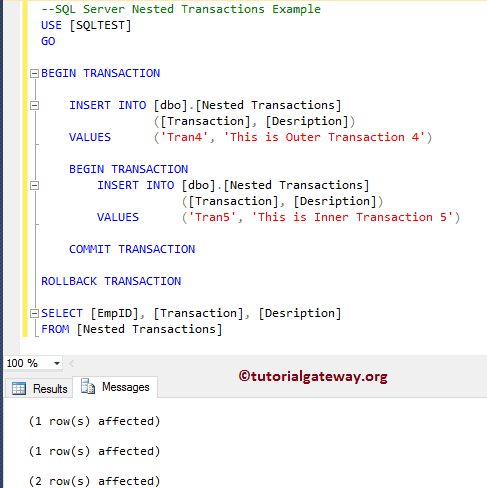
SQL Server Transactions Save Point Example
Let us see the working function of the SQL Transaction save points. In the below code snippet, we have SAVE TRAN, and this command will save the data up to that point.
For example, if any disaster happens after that point or any rollback command executes, don’t delete data before the Transaction save point.
SELECT 'Before Staring any Transaction', @@TRANCOUNT BEGIN TRANSACTION TRAN1 SELECT 'After Staring First Transaction', @@TRANCOUNT SAVE TRAN TRAN2 SELECT 'Within the Save Transaction', @@TRANCOUNT ROLLBACK TRAN TRAN2 SELECT 'After Rollback th Save Transaction', @@TRANCOUNT COMMIT TRANSACTION TRAN1 SELECT 'After the First Transaction is Commited', @@TRANCOUNT
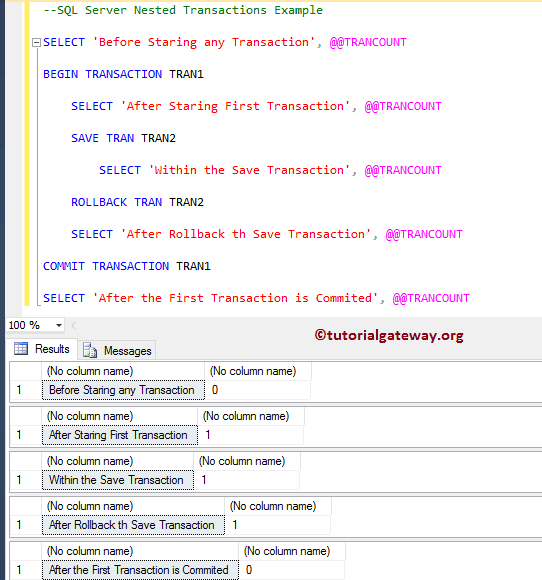
The practical example of Sql Server Transaction Save Point.
USE [SQLTEST] GO BEGIN TRANSACTION TRAN1 INSERT INTO [dbo].[Nested Transactions] ([Transaction], [Desription]) VALUES ('Tran6', 'This is Outer Transaction 6') SAVE TRANSACTION TRAN2 INSERT INTO [dbo].[Nested Transactions] ([Transaction], [Desription]) VALUES ('Tran7', 'This is Inner Transaction 7') ROLLBACK TRANSACTION TRAN2 COMMIT TRANSACTION TRAN2 SELECT [EmpID], [Transaction], [Desription] FROM [Nested Transactions]
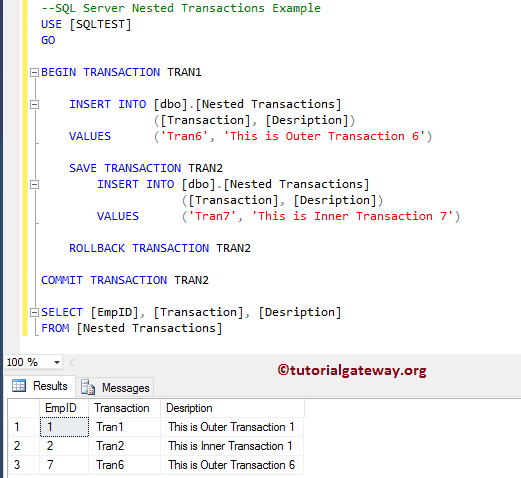