Write a Java Program to Print Even Numbers from 1 to N using If Statement and Conditional Operator with example. If the given number is divisible by 2, then it is an even number.
Java Program to Print Even Numbers from 1 to N Example
This program allows the user to enter the maximum limit value. Next, this Java program prints the even numbers from 1 to the maximum limit value using For Loop and If statements.
In Java Programming, we have a % (Module) Arithmetic Operator to check the remainder. If the remainder is 0, then the number is even.
import java.util.Scanner; public class EvenNumbers { private static Scanner sc; public static void main(String[] args) { int number, i; sc = new Scanner(System.in); System.out.print(" Please Enter any Number : "); number = sc.nextInt(); for(i = 1; i <= number; i++) { if(i % 2 == 0) { System.out.print(i +"\t"); } } } }
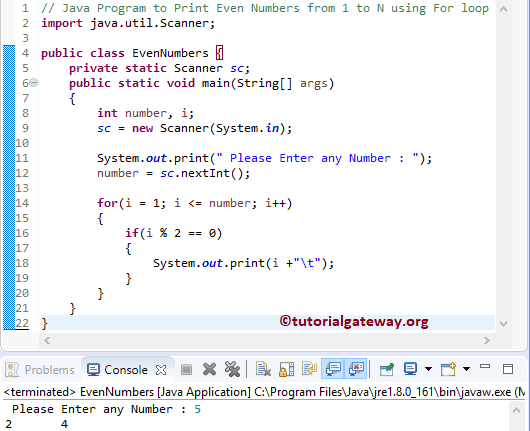
First, we used the For loop to iterate from 1 to the maximum value (Here, number = 5). As we know, if the number is entirely divisible by 2, it is an even number. So, we used the If condition to check whether the remainder of the number divided by 2 is exactly equal to 0 or not.
User entered value in this Java Program to Print Even Numbers from 1 to N : number = 5
For Loop First Iteration: for(i = 1; i <= 5; i++)
if(i % 2 == 0) => if(1 % 2 == 0) – the For loop Condition is False
Second Iteration: for(i = 2; 2 <= 5; 2++)
if(2 % 2 == 0) – Condition is True. So, i value printed
Third Iteration: for(i = 3; 3 <= 5; 3++)
if(3 % 2 == 0) – Condition is False
Fourth Iteration: for(i = 4; 4 <= 5; 4++)
if(4 % 2 == 0) – Condition is True. So, I value printed
Fifth Iteration: for(i = 5; 5 <= 5; 5++)
if(5 % 2 == 0) – Condition is False
Sixth Iteration: for(i = 6; 6 <= 5; 6++)
Condition (6 <= 5) is False. So, the Java compiler exits from the For Loop
Java Program to Print Even Numbers from 1 to N Example 2
This program is the same as above, but we altered for loop to eliminate the If statement. If you observe the below code snippet, We started i from 2 and increment it by 2 (not 1). It means, for the first iteration, i become 2, and for the second iteration, i become 4 (not 3) so on.
import java.util.Scanner; public class EvenNumbers1 { private static Scanner sc; public static void main(String[] args) { int number, i; sc = new Scanner(System.in); System.out.print(" Please Enter any Number : "); number = sc.nextInt(); for(i = 2; i <= number; i = i + 2) { System.out.print(i +"\t"); } } }
Even numbers using for loop output
Please Enter any Number : 40
2 4 6 8 10 12 14 16 18 20 22 24 26 28 30 32 34 36 38 40
Java Program to Print Even Numbers from 1 to N Example 3
This program to return even numbers from 1 to 100 is the same as the second example, but we are using the While Loop.
import java.util.Scanner; public class EvenNumbers2 { private static Scanner sc; public static void main(String[] args) { int number, i; sc = new Scanner(System.in); System.out.print(" Please Enter any Number : "); number = sc.nextInt(); i = 2; while(i <= number) { System.out.print(i +"\t"); i = i + 2; } } }
Using a While Loop output
Please Enter any Number : 25
2 4 6 8 10 12 14 16 18 20 22 24
Java Program to Print Even Numbers from 1 to N using Method
This Java program is the same as the first example. But we separated the even number from 1 to N logic and placed it in a separate method.
import java.util.Scanner; public class EvenNumbers3 { private static Scanner sc; public static void main(String[] args) { int number; sc = new Scanner(System.in); System.out.print(" Please Enter any Number : "); number = sc.nextInt(); findEven(number); } public static void findEven(int num) { int i; for(i = 1; i <= num; i++) { if(i % 2 == 0) { System.out.print(i +"\t"); } } } }
Please Enter any Number : 70
2 4 6 8 10 12 14 16 18 20 22 24 26 28 30 32 34 36 38 40 42 44 46 48 50 52 54 56 58 60 62 64 66 68 70
Java Program to Print Even Numbers between a Given Range
This Java program allows the user to enter Minimum and maximum values. Next, this program displays a list of even numbers between the minimum and maximum values.
import java.util.Scanner; public class EvenNumbers4 { private static Scanner sc; public static void main(String[] args) { int minimum, maximum; sc = new Scanner(System.in); System.out.print(" Please Enter the Minimum value : "); minimum = sc.nextInt(); System.out.print(" Please Enter the Maximum value : "); maximum = sc.nextInt(); findEven(minimum, maximum); } public static void findEven(int minimum, int maximum) { int i; if(minimum % 2 != 0) { minimum++; } for(i = minimum; i <= maximum; i++) { if(i % 2 == 0) { System.out.print(i +"\t"); } } } }
Even numbers from 5 to 150 Output
Please Enter the Minimum value : 5
Please Enter the Maximum value : 150
6 8 10 12 14 16 18 20 22 24 26 28 30 32 34 36 38 40 42 44 46 48 50 52 54 56 58 60 62 64 66 68 70 72 74 76 78 80 82 84 86 88 90 92 94 96 98 100 102 104 106 108 110 112 114 116 118 120 122 124 126 128 130 132 134 136 138 140 142 144 146 148 150
Please refer to
- First 10 Even Natural Numbers
- First 10 Natural Numbers
- First 10 Natural Numbers in Reverse
- First 10 Odd Natural Numbers
- Print Odd Numbers from 1 to N
- Print Natural Numbers from 1 to N
- Print Natural Numbers in Reverse Order
- Java Program to Find the Sum of Natural Numbers from 1 to N
- The Sum of Even and Odd Numbers
- The Sum of Even Numbers
- The Sum of Odd Numbers