Write a Java program to multiply two Matrices with an example, or write a program to perform the multiplication of two multidimensional arrays. To perform the matrix multiplication, the number of columns in the first matrix must equal the total number of rows in the second matrix.
Java program to Multiply two Matrices
In this example, we declared two integer matrices. Next, we used the For Loop to iterate those values. Then, we performed multiplication on x and y matrixes within that loop and assigned it to another one called multi. Later, we used another for loop to print the final output.
- Z11 = x11 * y11 = 1 * 5 = 5
- Z12 = x12 * y12 = 2 * 6 = 12
- Z13 = x13 * y13 = 3 * 7 = 21
- Z22 = x22 * y22 = 5 * 9 = 45
public class MultiplyTwoMatrix { public static void main(String[] args) { int[][] x = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}}; int[][] y = {{ 5, 6, 7}, {8, 9,10}, {2,3, 4}}; int[][] multi = new int[3][3]; int i, j; for(i = 0; i < x.length; i++) { for(j = 0; j < x[0].length; j++) { multi[i][j] = x[i][j] * y[i][j]; } } System.out.println("------ The Multiplication of two Matrix ------"); for(i = 0; i < x.length; i++) { for(j = 0; j < x[0].length; j++) { System.out.format("%d \t", multi[i][j]); } System.out.println(""); } } }
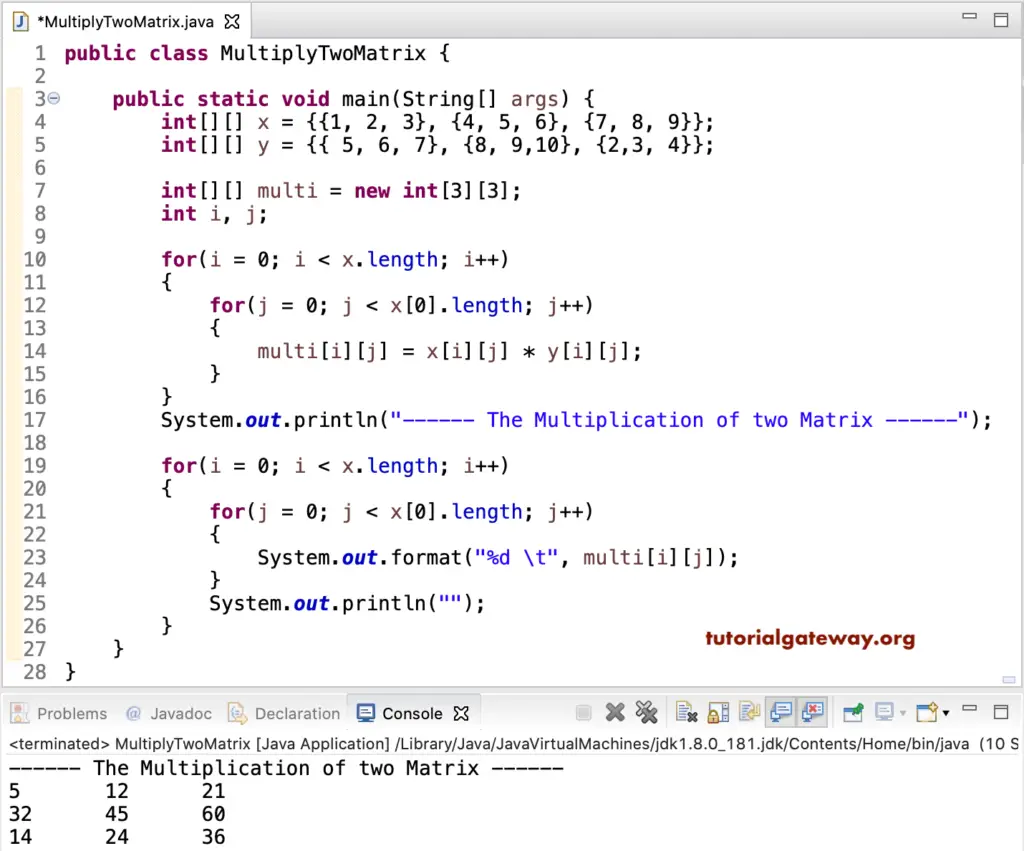
Java program to Multiply two Matrices using for loop
This Java program is the same as above. However, this code allows the users to enter the rows, columns, and matrix items.
import java.util.Scanner; public class Example { private static Scanner sc; public static void main(String[] args) { int i, j, rows, columns; sc= new Scanner(System.in); System.out.println("\n Enter Rows & Columns : "); rows = sc.nextInt(); columns = sc.nextInt(); int[][] arr1 = new int[rows][columns]; int[][] arr2 = new int[rows][columns]; System.out.println("\n Enter the First Mat Items : "); for(i = 0; i < rows; i++) { for(j = 0; j < columns; j++) { arr1[i][j] = sc.nextInt(); } } System.out.println("\n Enter the Second Mat Items : "); for(i = 0; i < rows; i++) { for(j = 0; j < columns; j++) { arr2[i][j] = sc.nextInt(); } } System.out.println("\n-----The Multiplication of two Matrix----- "); for(i = 0; i < rows; i++) { for(j = 0; j < columns; j++) { System.out.format("%d \t", (arr1[i][j] * arr2[i][j])); } System.out.println(""); } } }
Enter Rows & Columns :
3 3
Enter the First Mat Items :
1 2 3
4 5 6
7 8 9
Enter the Second Mat Items :
10 20 30
40 50 60
70 80 90
-----The Multiplication of two Matrix-----
10 40 90
160 250 360
490 640 810